Yes, we should always be doing unit tests, E2E tests, integration tests, and whatever other sort of tests are the flavor of the month. But you might also find yourself working on a proof of concept that tests are just going to be overkill on.
Even so, each time you run an ng generate command, you end up with an annoying spec file that isn’t too hard to delete, but is just hella annoying to have to do each time. Luckily Angular provides a way to turn off spec files via the CLI. Let’s take a look.
When Creating A New Project
When creating a new project, there is a way to skip the entire rest of this tutorial by one simple flag.
ng new --skipTests
When creating your new project, simply pass the –skip-tests flag and “theoretically” it should skip *ALL* tests right?
Well wrong (As of Angular 8). You will probably see the following fly by :
CREATE SkipTestsFromStart/e2e/protractor.conf.js (808 bytes)
CREATE SkipTestsFromStart/e2e/tsconfig.json (214 bytes)
CREATE SkipTestsFromStart/e2e/src/app.e2e-spec.ts (651 bytes)
CREATE SkipTestsFromStart/e2e/src/app.po.ts (262 bytes)
So as it turns out there is an open bug on the Angular Github Repo for this exact issue : https://github.com/angular/angular-cli/issues/9160. Personally I think it makes sense that when you say “Hey, I’m not doing tests”, that you actually mean it, but Angular gonna Angular.
So if it still creates the e2e folders, what does it actually do when you pass –skipTests? Well it adds the following into your angular.json file :
{
...
"projects": {
"SkipTestsFromStart": {
"projectType": "application",
"schematics": {
"@schematics/angular:component": {
"style": "scss",
"skipTests": true
},
"@schematics/angular:class": {
"skipTests": true
},
"@schematics/angular:directive": {
"skipTests": true
},
"@schematics/angular:guard": {
"skipTests": true
},
"@schematics/angular:module": {
"skipTests": true
},
"@schematics/angular:pipe": {
"skipTests": true
},
"@schematics/angular:service": {
"skipTests": true
}
}
...
}
What it’s essentially doing is saying “Hey, when I create these things, don’t create tests”. So for example if we run the following command :
ng generate component MyNewFeature
It won’t generate the spec file along with it. Awesome!
When Working On An Existing Project
You may have already created your project so passing the –skipTests flag to the ng new command isn’t going to cut it. So you have two ways you can work around this (Or really one if I’m being honest).
The first way is less than ideal because 9 times out of 10 you forget to pass the flag. That is, when you run ng generate , you can pass skipTests in there :
ng generate component MyNewFeature --skipTests
Note that in older versions of Angular this was a spec flag that you passed true or false in. This has now been deprecated but if you are on an old version of Angular it will look like so :
ng generate component MyNewFeature --spec=false
It’s quickly going to become a headache to always pass this into your generate commands. So a better way is to take the above schematics JSON that we talked about in the “When Create A New Project” section, and put that into your angular.json.
For example, if I don’t want new components generating a spec file, we can add the following to our angular.json file :
{
"$schema": "./node_modules/@angular/cli/lib/config/schema.json",
"version": 1,
"newProjectRoot": "projects",
"projects": {
"MyProject": {
"projectType": "application",
"schematics": {
"@schematics/angular:component": {
"style": "scss",
"skipTests": true
}
.....
}
I’ll also note that you can pick and choose where you do this. For example you could disable tests for components only, but still generate tests for your services, pipes and guards.
The best way I’ve found to get the JSON just right is to create a brand new project in another folder using
ng new --skipTests
And then copy and paste over the JSON you need. This will also give you a good idea of what how fine grain you can go when turning off tests (Or not turning them off as it might be).
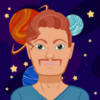
💬 Leave a comment