Angular
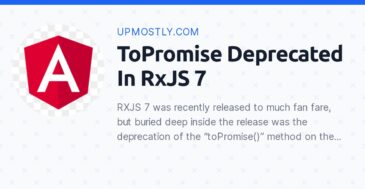
ToPromise Deprecated In RxJS 7
RXJS 7 was recently released to much fan fare, but buried deep inside the release was the deprecation of the “toPromise()” method on the Observable type. For example, this sort of code : let myPromise = myObservable.toPromise(); Now gives you the following warning : @deprecated — Replaced with firstValueFrom and [...]
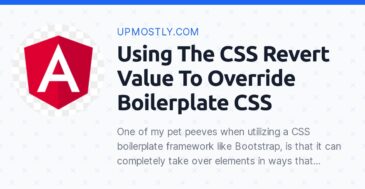
Using The CSS Revert Value To Override Boilerplate CSS
One of my pet peeves when utilizing a CSS boilerplate framework like Bootstrap, is that it can completely take over elements in ways that I may want to back out of later. Bootstrap isn’t the worst offender of this these days, because for the most part you have to apply [...]
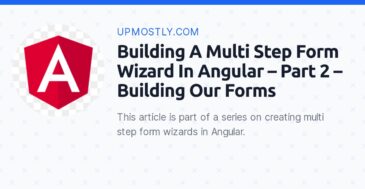
Building A Multi Step Form Wizard In Angular – Part 2 – Building Our Forms
This article is part of a series on creating multi step form wizards in Angular. Part 1 – Basic SetupPart 2 – Building Our FormsPart 3 – Tying It All Together In the last part of this series on [...]
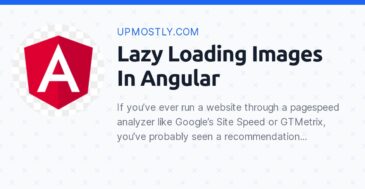
Lazy Loading Images In Angular
If you’ve ever run a website through a pagespeed analyzer like Google’s Site Speed or GTMetrix, you’ve probably seen a recommendation that you should lazy load images below the fold. That is, when your website first loads, only show images that are actually visible on screen, then as you scroll, [...]
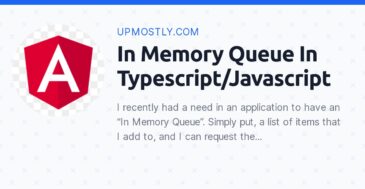
In Memory Queue In Typescript/Javascript
I recently had a need in an application to have an “In Memory Queue”. Simply put, a list of items that I add to, and I can request the oldest items out of the queue. It’s a very common construct in other programming languages/platforms such as Java or C#. Unfortunately, [...]
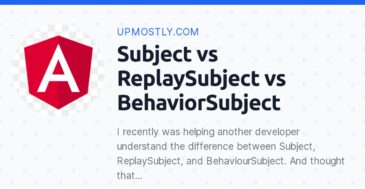
Subject vs ReplaySubject vs BehaviorSubject
I recently was helping another developer understand the difference between Subject, ReplaySubject, and BehaviourSubject. And thought that the following examples explain the differences perfectly. Subject A subject is like a turbocharged observable. It can almost be thought of an event message pump in that everytime a value is emitted, all [...]
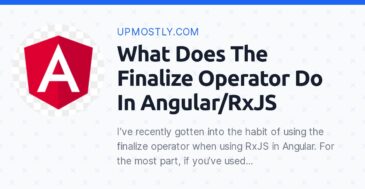
What Does The Finalize Operator Do In Angular/RxJS
I’ve recently gotten into the habit of using the finalize operator when using RxJS in Angular. For the most part, if you’ve used languages that have try/catch statements, they generally have a “finally” statement too. For example, in plain old javascript you can do something like : try { DoWork(); [...]
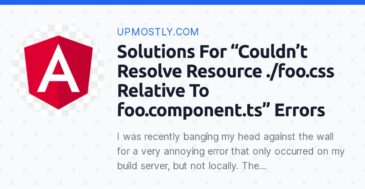
Solutions For “Couldn’t Resolve Resource ./foo.css Relative To foo.component.ts” Errors
I was recently banging my head against the wall for a very annoying error that only occurred on my build server, but not locally. The error in question was when a component referenced an external style sheet, I was getting the following error : Couldn’t Resolve Resource [...]
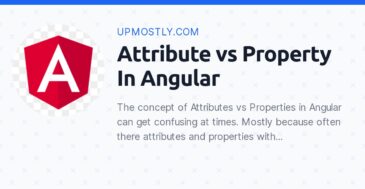
Attribute vs Property In Angular
The concept of Attributes vs Properties in Angular can get confusing at times. Mostly because often there attributes and properties with the exact same name. If we take a simply example, using an input html tag such as : <input type="text" id="nameInput" value="Jim"> Here we have an input HTML tag [...]
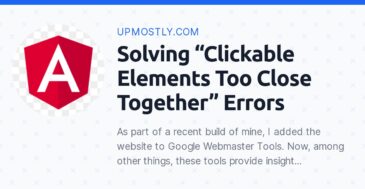
Solving “Clickable Elements Too Close Together” Errors
As part of a recent build of mine, I added the website to Google Webmaster Tools. Now, among other things, these tools provide insight into how Google sees your website functioning, and also gives feedback on any issues it found while crawling your pages. A big focus for Google in [...]
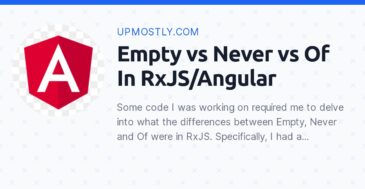
Empty vs Never vs Of In RxJS/Angular
Some code I was working on required me to delve into what the differences between Empty, Never and Of were in RxJS. Specifically, I had a method that returned an observable purely so that I could “wait” or “subscribe” for it to finish (e.g. It did not return an actual [...]
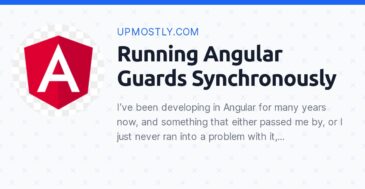
Running Angular Guards Synchronously
I’ve been developing in Angular for many years now, and something that either passed me by, or I just never ran into a problem with it, is that if a route has multiple route guards, they will all run at once asynchronously. Obviously the result of all of these are [...]
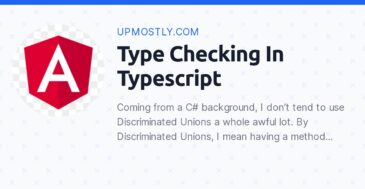
Type Checking In Typescript
Coming from a C# background, I don’t tend to use Discriminated Unions a whole awful lot. By Discriminated Unions, I mean having a method that may take multiple different types, or may return multiple different types. If you’ve come from a C# world, it’s basically like method overloads when passing [...]
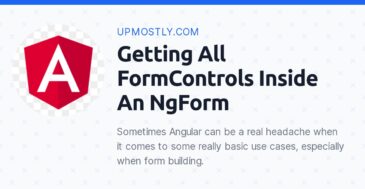
Getting All FormControls Inside An NgForm
Sometimes Angular can be a real headache when it comes to some really basic use cases, especially when form building. Case and point, I recently had to get all controls within a ngForm so that I could run a custom method on them. At first, I figured that something like [...]
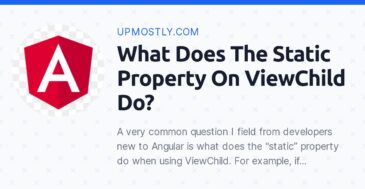
What Does The Static Property On ViewChild Do?
A very common question I field from developers new to Angular is what does the “static” property do when using ViewChild. For example, if we have code like so : @ViewChild(ChildComponent, {static : false}) childComponent : ChildComponent; What exactly does setting static to false do? I think the documentation is [...]
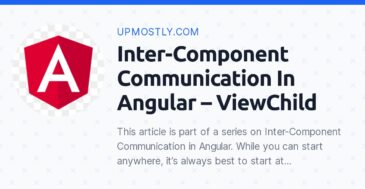
Inter-Component Communication In Angular – ViewChild
This article is part of a series on Inter-Component Communication in Angular. While you can start anywhere, it’s always best to start at the beginning right! Part 1 – Input BindingPart 2 – Output BindingPart 3 – Joining [...]
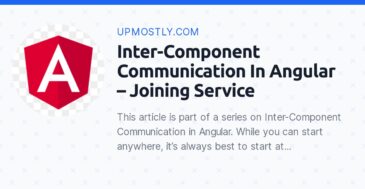
Inter-Component Communication In Angular – Joining Service
This article is part of a series on Inter-Component Communication in Angular. While you can start anywhere, it’s always best to start at the beginning right! Part 1 – Input BindingPart 2 – Output BindingPart 3 – Joining Service [...]
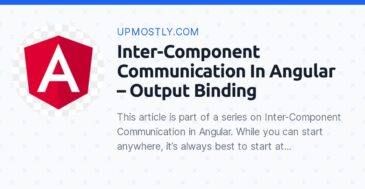
Inter-Component Communication In Angular – Output Binding
This article is part of a series on Inter-Component Communication in Angular. While you can start anywhere, it’s always best to start at the beginning right! Part 1 – Input BindingPart 2 – Output BindingPart 3 – Joining Service [...]
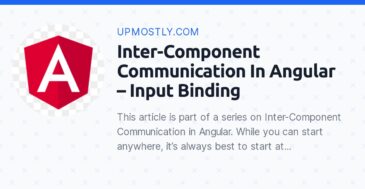
Inter-Component Communication In Angular – Input Binding
This article is part of a series on Inter-Component Communication in Angular. While you can start anywhere, it’s always best to start at the beginning right! Part 1 – Input BindingPart 2 – Output BindingPart 3 – Joining Service [...]
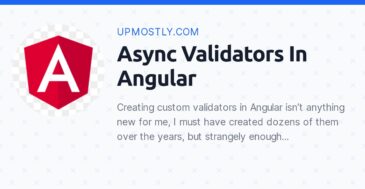
Async Validators In Angular
Creating custom validators in Angular isn’t anything new for me, I must have created dozens of them over the years, but strangely enough I haven’t had to create “async” validators in recent memory. When I tried to find more information about making a custom validator that returned an observable (or [...]
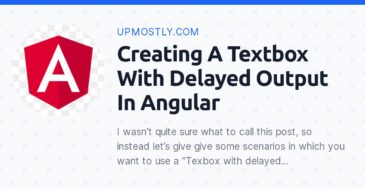
Creating A Textbox With Delayed Output In Angular
I wasn’t quite sure what to call this post, so instead let’s give give some scenarios in which you want to use a “Texbox with delayed output” You want to create your own custom typeahead component that waits until a user stops typing before looking up resultsYou want a textbox [...]
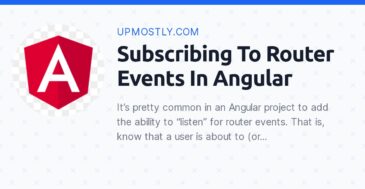
Subscribing To Router Events In Angular
It’s pretty common in an Angular project to add the ability to “listen” for router events. That is, know that a user is about to (or already has) navigated to a new page. The most common reason is to push pageview events to analytics platforms (think Google Analytics or Azure [...]
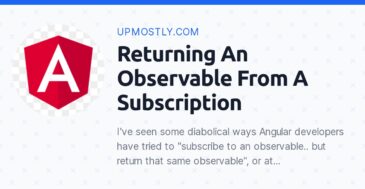
Returning An Observable From A Subscription
I’ve seen some diabolical ways Angular developers have tried to “subscribe to an observable.. but return that same observable”, or at least that’s how it was explained to me when I saw the following code : getData() { let observable = this.myService.getData(); observable.subscribe(x => { //do something with the data [...]
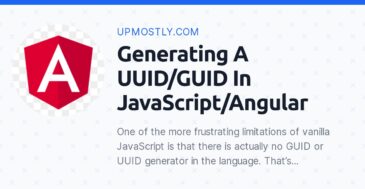
Generating A UUID/GUID In JavaScript/Angular
One of the more frustrating limitations of vanilla JavaScript is that there is actually no GUID or UUID generator in the language. That’s right, out of the box, you cannot generate a GUID with a nice single line of code! Very annoying. Luckily, there are two ways that I’ve used [...]
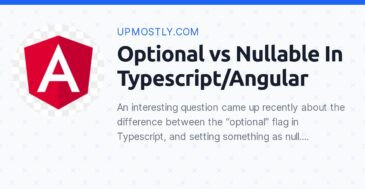
Optional vs Nullable In Typescript/Angular
An interesting question came up recently about the difference between the “optional” flag in Typescript, and setting something as null. More importantly, it was a question of whether the following were synonymous : export class MyClass { myProperty? : string; } export class MyClass { myProperty : string | null; [...]
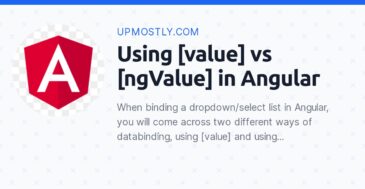
Using [value] vs [ngValue] in Angular
When binding a dropdown/select list in Angular, you will come across two different ways of databinding, using [value] and using [ngValue]. They actually have a very simple difference. But let’s whip up an example first. Imagine I have a component that holds a list of books : export class AppComponent [...]
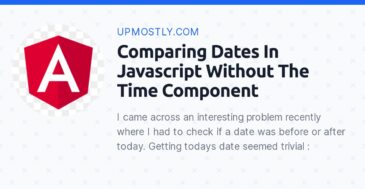
Comparing Dates In Javascript Without The Time Component
I came across an interesting problem recently where I had to check if a date was before or after today. Getting todays date seemed trivial : let today = new Date(); But in my test cases, things were blowing up, often at weird times of the day. And it occurred [...]
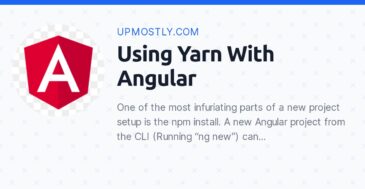
Using Yarn With Angular
One of the most infuriating parts of a new project setup is the npm install. A new Angular project from the CLI (Running “ng new”) can even take several minutes. And even if it’s an existing project that you know you have already setup, but you just want to check, [...]
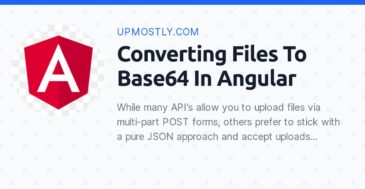
Converting Files To Base64 In Angular
While many API’s allow you to upload files via multi-part POST forms, others prefer to stick with a pure JSON approach and accept uploads in base64 format allowing you to always be sending and receiving JSON. This means that if a user selects a file in your Angular app, you [...]
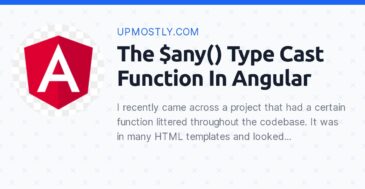
The $any() Type Cast Function In Angular
I recently came across a project that had a certain function littered throughout the codebase. It was in many HTML templates and looked something like : {{$any(myVariable).property}} At first, the use of the dollar sign $ threw me off (Is someone introducing jQuery here?!). But infact, it’s a special operator [...]
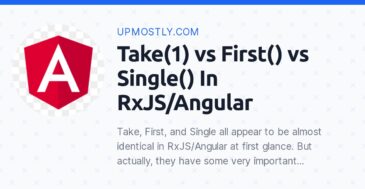
Take(1) vs First() vs Single() In RxJS/Angular
Take, First, and Single all appear to be almost identical in RxJS/Angular at first glance. But actually, they have some very important differences that could throw up some very weird errors if you aren’t expecting them. If you have spent any time working with C# LINQ expressions at all, you [...]
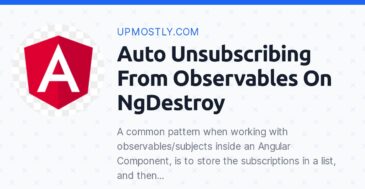
Auto Unsubscribing From Observables On NgDestroy
A common pattern when working with observables/subjects inside an Angular Component, is to store the subscriptions in a list, and then unsubscribe when the component is destroyed. Now I’ve seen many developers not bother unsubscribing at all when it comes to HttpClient requests, but it’s a good habit to get [...]
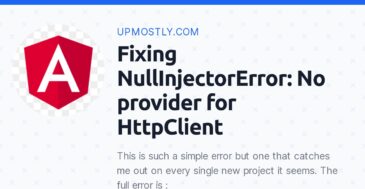
Fixing NullInjectorError: No provider for HttpClient
This is such a simple error but one that catches me out on every single new project it seems. The full error is : Error: StaticInjectorError[HttpClient]:StaticInjectorError[HttpClient]:NullInjectorError: No provider for HttpClient! Unfortunately it’s not that descriptive, but it’s an easy fix. In your app.module.ts, you need to import the [...]
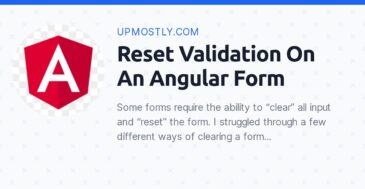
Reset Validation On An Angular Form
Some forms require the ability to “clear” all input and “reset” the form. I struggled through a few different ways of clearing a form before I found a foolproof way that seemed to work in all scenarios I tested. First, what didn’t work. NGForm does have two reset methods : [...]
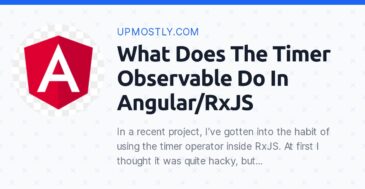
What Does The Timer Observable Do In Angular/RxJS
In a recent project, I’ve gotten into the habit of using the timer operator inside RxJS. At first I thought it was quite hacky, but actually.. It can come in incredible useful in scenarios that you may have previously used setTimeout. That might make it sound even worse! But let [...]
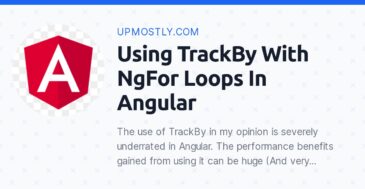
Using TrackBy With NgFor Loops In Angular
The use of TrackBy in my opinion is severely underrated in Angular. The performance benefits gained from using it can be huge (And very noticeable) for such a small code change. But before we dive too deep, let’s talk about how an NgFor loop works in Angular when you don’t [...]
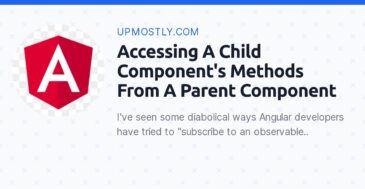
Accessing A Child Component’s Methods From A Parent Component
In some very rare cases, you may need to call a child component’s method directly from a parent component. Generally speaking, this should be seen as only a last resort. Component communication in most cases should be limited to data binding (Both input and output), and and in some cases [...]
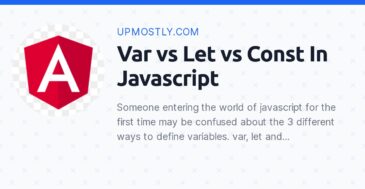
Var vs Let vs Const In Javascript
Someone entering the world of javascript for the first time may be confused about the 3 different ways to define variables. var, let and const all seem to do roughly the same thing, so which one is actually correct to use? And why do we have all 3 in the [...]
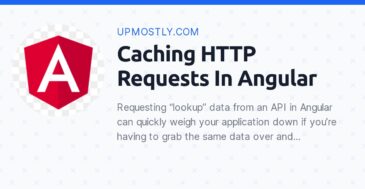
Caching HTTP Requests In Angular
Requesting “lookup” data from an API in Angular can quickly weigh your application down if you’re having to grab the same data over and over. The type of lookup data I’m talking about is say fixed country lists, or a set of user roles, or any other set of data [...]
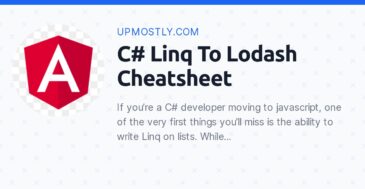
C# Linq To Lodash Cheatsheet
If you’re a C# developer moving to javascript, one of the very first things you’ll miss is the ability to write Linq on lists. While Javascript has a couple of methods to deal with arrays, some of them don’t quite match up to the power of Linq. Luckily, there is [...]
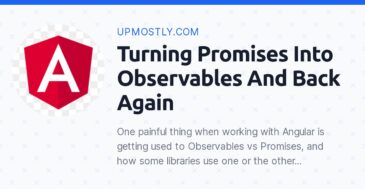
Turning Promises Into Observables And Back Again
One painful thing when working with Angular is getting used to Observables vs Promises, and how some libraries use one or the other exclusively. It can be incredibly frustrating to add a library only to find it wants to force you into using promises, when the rest of your project [...]
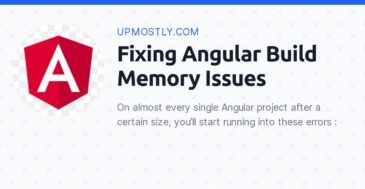
Fixing Angular Build Memory Issues
On almost every single Angular project after a certain size, you’ll start running into these errors : An unhandled exception occurred: Call retries were exceeded Or JavaScript heap out of memory Both of these point to the same issue. That your build process is hitting memory limits and simply crashing.
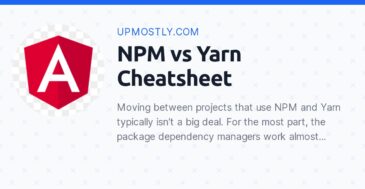
NPM vs Yarn Cheatsheet
Moving between projects that use NPM and Yarn typically isn’t a big deal. For the most part, the package dependency managers work almost identical. But one thing that does tend to trip developers up is the subtle command line changes between the two. Often it’s just the case of swapping [...]
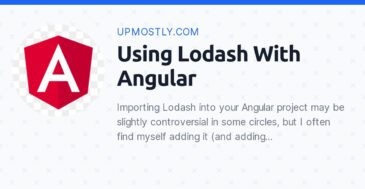
Using Lodash With Angular
Importing Lodash into your Angular project may be slightly controversial in some circles, but I often find myself adding it (and adding the few KB that comes along with it), rather than reinventing the wheel in each project. If you’ve never used it before, Lodash is a javascript [...]
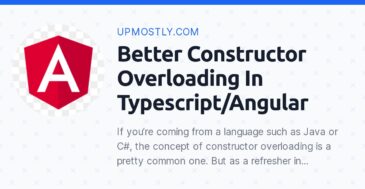
Better Constructor Overloading In Typescript/Angular
If you’re coming from a language such as Java or C#, the concept of constructor overloading is a pretty common one. But as a refresher in say C#, we can overload constructors like so : class MyClass { public MyClass(string value) { this.value = value; } public MyClass(int value) { [...]
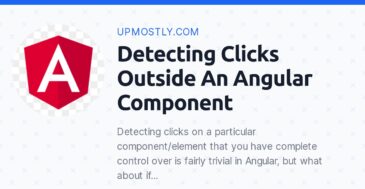
Detecting Clicks Outside An Angular Component
Detecting clicks on a particular component/element that you have complete control over is fairly trivial in Angular, but what about if you want to know when a user clicks somewhere on the page on something that is *not* inside a particular component. It may sound like a weird edgecase but it’s actually [...]
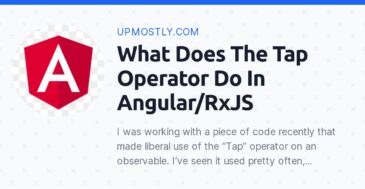
What Does The Tap Operator Do In Angular/RxJS
I was working with a piece of code recently that made liberal use of the “Tap” operator on an observable. I’ve seen it used pretty often, but everytime I try and find documentation to show a junior developer how it works, I always find it a really overcomplicated mess. Tap, [...]
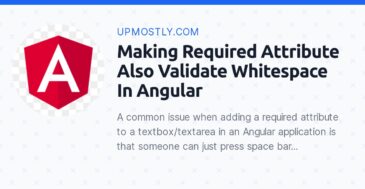
Making Required Attribute Also Validate Whitespace In Angular
A common issue when adding a required attribute to a textbox/textarea in an Angular application is that someone can just press space bar once, and the “required” validation is completed. A space counts as character! The simple way to get around this is to use a regex pattern attribute to [...]
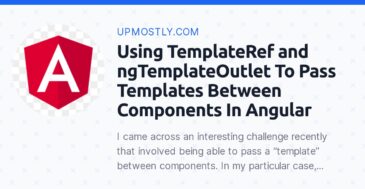
Using TemplateRef and ngTemplateOutlet To Pass Templates Between Components In Angular
I came across an interesting challenge recently that involved being able to pass a “template” between components. In my particular case, I had a “table” component that simply laid out tabular data, nothing too crazy. In most cases I simply wanted the text value of a property to be displayed, [...]
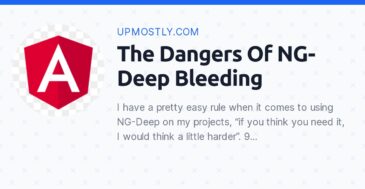
The Dangers Of NG-Deep Bleeding
I have a pretty easy rule when it comes to using NG-Deep on my projects, “if you think you need it, I would think a little harder”. 9 times out of 10 when I see people using the ng-deep modifier, it’s because they want a “quick fix” without really thinking [...]
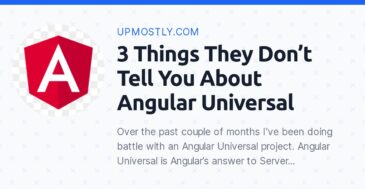
3 Things They Don’t Tell You About Angular Universal
Over the past couple of months I’ve been doing battle with an Angular Universal project. Angular Universal is Angular’s answer to Server Side Rendering or SSR for short. Why use SSR? In most cases it’s for SEO purposes but in some cases it also gives “perceived” performance. For [...]
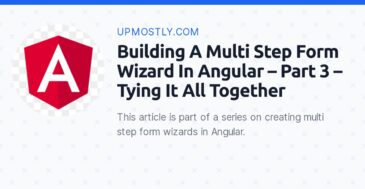
Building A Multi Step Form Wizard In Angular – Part 3 – Tying It All Together
This article is part of a series on creating multi step form wizards in Angular. Part 1 – Basic SetupPart 2 – Building Our FormsPart 3 – Tying It All Together We are on the home stretch with our multi-step form, infact [...]
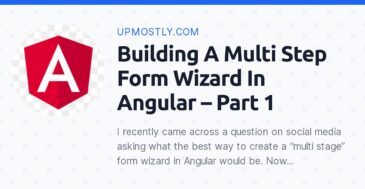
Building A Multi Step Form Wizard In Angular – Part 1
This article is part of a series on creating multi step form wizards in Angular. Part 1 – Basic SetupPart 2 – Building Our FormsPart 3 – Tying It All Together I recently came across a question on social media asking what [...]
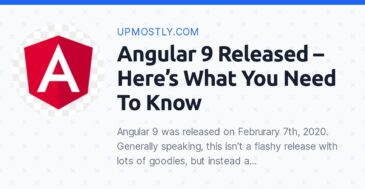
Angular 9 Released – Here’s What You Need To Know
Angular 9 was released on Februrary 7th, 2020. Generally speaking, this isn’t a flashy release with lots of goodies, but instead a release that gives a huge (needed) update to plenty of behind the scene components. Angular Ivy Angular Ivy is the name given to Angular’s new compilation and rendering [...]
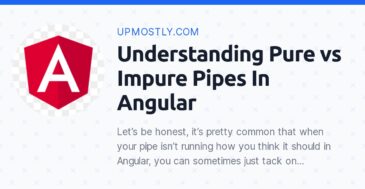
Understanding Pure vs Impure Pipes In Angular
Let’s be honest, it’s pretty common that when your pipe isn’t running how you think it should in Angular, you can sometimes just tack on the “pure : false” tag like so @Pipe({ name: 'myPipe', pure : false }) And you just hope for the best. But this actually has [...]
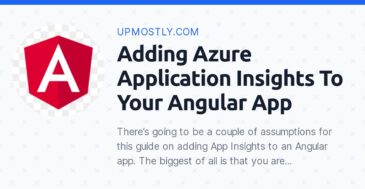
Adding Azure Application Insights To Your Angular App
There’s going to be a couple of assumptions for this guide on adding App Insights to an Angular app. The biggest of all is that you are familiar with the Application Insights product itself. Maybe you’ve used in an another app before, maybe in a C# backend application or maybe [...]
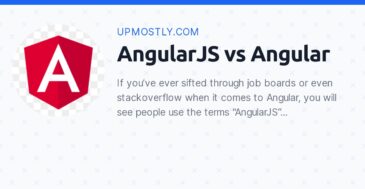
AngularJS vs Angular
If you’ve ever sifted through job boards or even stackoverflow when it comes to Angular, you will see people use the terms “AngularJS” and “Angular” interchangeably. Or atleast that’s what it can sometimes appear to be. But AngularJS and Angular actually refer to two very different versions of Angular. While [...]
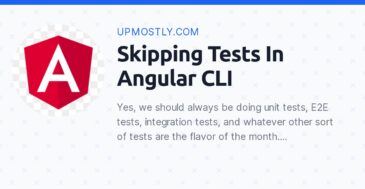
Skipping Tests In Angular CLI
Yes, we should always be doing unit tests, E2E tests, integration tests, and whatever other sort of tests are the flavor of the month. But you might also find yourself working on a proof of concept that tests are just going to be overkill on. Even so, each time you [...]
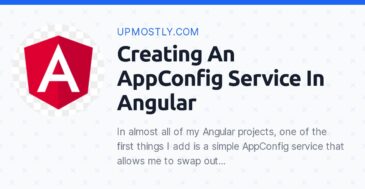
Creating An AppConfig Service In Angular
In almost all of my Angular projects, one of the first things I add is a simple AppConfig service that allows me to swap out configurations on the fly for different environments. In most cases, this is so that I can set a different base API endpoint for my different [...]
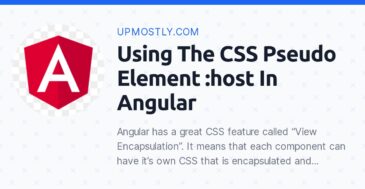
Using The CSS Pseudo Element :host In Angular
Angular has a great CSS feature called “View Encapsulation”. It means that each component can have it’s own CSS that is encapsulated and applied to only that particular component/view. This does away with having to have great big long CSS declarations to try and narrow down the element you want [...]
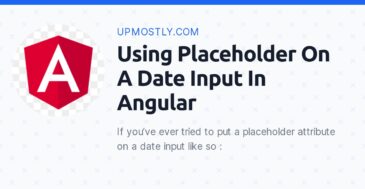
Using Placeholder On A Date Input In Angular
If you’ve ever tried to put a placeholder attribute on a date input like so : <input type="text" name="myDateField" placeholder="Enter the date here" /> You know it doesn’t work. Instead what you end up with is the field being pre-populated with “dd/MM/yyyy” which is incredibly annoying! But there is actually [...]
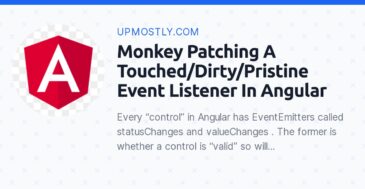
Monkey Patching A Touched/Dirty/Pristine Event Listener In Angular
Every “control” in Angular has EventEmitters called statusChanges and valueChanges . The former is whether a control is “valid” so will emit an event every time a control changes from invalid to valid or vice versa. valueChanges is when the actual control’s “value” changes. In most cases this is going to be a form [...]
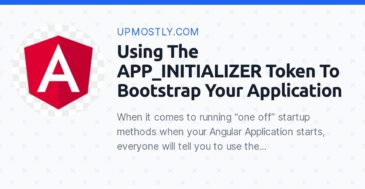
Using The APP_INITIALIZER Token To Bootstrap Your Application
When it comes to running “one off” startup methods when your Angular Application starts, everyone will tell you to use the “APP_INITIALIZER” token. That’s all well and good to be told that, but when you actually check the documentation, it’s a little sparse… Admittedly there is a bit more on [...]
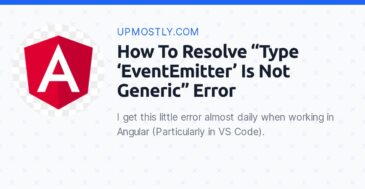
How To Resolve “Type ‘EventEmitter’ Is Not Generic” Error
I get this little error almost daily when working in Angular (Particularly in VS Code). Type 'EventEmitter' is not generic. The first time I got the issue, it drove me mad as I was copy and pasting code from another file where it was working just fine, yet in my [...]
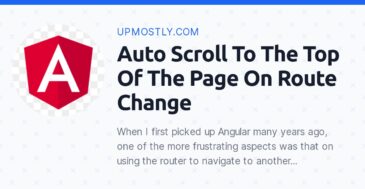
Auto Scroll To The Top Of The Page On Route Change
When I first picked up Angular many years ago, one of the more frustrating aspects was that on using the router to navigate to another page, the scroll position was kept. This meant you were taken to the new page, but you would be scrolled halfway down the page (Or [...]
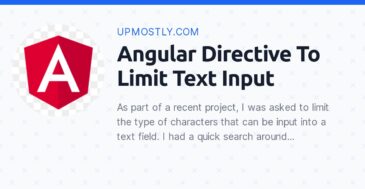
Angular Directive To Limit Text Input
As part of a recent project, I was asked to limit the type of characters that can be input into a text field. I had a quick search around but every single time I thought I had found a solution, it was some form of using the pattern attribute to [...]