Some forms require the ability to “clear” all input and “reset” the form. I struggled through a few different ways of clearing a form before I found a foolproof way that seemed to work in all scenarios I tested.
First, what didn’t work. NGForm does have two reset methods :
this.myForm.reset();
And
this.myForm.resetForm();
Both of these “appeared” to work, but I found it completely broke data binding at times where values got reset to null and lost their data binding. I personally use Template Driven Forms rather than Reactive Forms, so it may work in different scenarios, but for me, this really broke data binding.
If you use Template Driven Forms like me, then the best way I found to actually wipe values in the form was to first reset the underlying two way data binding object. E.g. If I’m binding to a “Person” object then I first do new Person() etc.
Then I can run the following code which only seems to reset the validity of controls rather than fiddling with the underlying data:
Object.keys(this.myForm.controls).forEach((key) => {
const control = this.myForm.controls[key];
control.markAsPristine();
control.markAsUntouched();
});
This runs through every control and resets the validity without affecting binding. This seemed to work the best for me. An alternative I found would be to change the call to setErrors()
Object.keys(this.myForm.controls).forEach((key) => {
const control = this.myForm.controls[key];
control.setErrors(null);
});
Again, the first iteration worked for me, but try both if it doesn’t for you.
Adding An NGForm Extension Method
Now rather than copy and pasting this everywhere, instead I created an extension. I created a file called ng-form.extensions.ts with the following content :
import { NgForm } from '@angular/forms';
declare module "@angular/forms/" {
interface NgForm {
resetValidation(this:Form) : void;
}
}
NgForm.prototype.resetValidation = function(this:NgForm) {
Object.keys(this.controls).forEach((key) => {
const control = this.controls[key];
control.markAsPristine();
control.markAsUntouched();
});
}
Then in my component, I have to import this extension file like so :
import 'src/app/_core/extensions/ng-form.extensions'
And I then simply reset the form like so :
this.myForm.resetValidation();
Much cleaner!
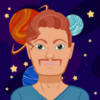
💬 Leave a comment