In this article, we are going to make our react hook forms perform even better with minimal code. We are going to inject validations so that we can check our data and inform the user accordingly with a suitable error message, with a bare minimum code.
Why Validation?
There are two solid reasons why you’d want to apply validation in your client-side forms.
- You get the accurate data, one that you are expecting
- Make the experience better for your end-user with suitable error messages.
Examples
Let’s say you want the user’s phone number but in a certain format maybe it should start with ‘+’, to make that happen you’d need some sort of mechanism to check what the user submitted and tell them how to enter it if it was wrong.
Maybe you want to reassure for your sanity that the user enters a valid email address, by valid we mean that it is a valid email format not that your system actually checks if it exists. (Duhhhh 🙄).
Or to force a user to not skip a field that is required. These are some things you can check with form validation.
Implementation
One way you could make validation work is define your custom hook and validate your data in there before submitting it.
Another way could be to define rules when you register your DOM element with react hook forms. As
<input
placeholder="Enter your password"
hidden
{...register("password", { required: true })}
/>
This looks tidy but there is an even better way to do validation. One of my favorites and a very powerful combination is react hook forms with Yup. You can do wonders with this combination with minimum code. Let’s see it in action.
npm i @hookform/resolvers yup
Once you have yup and @hookform/resolvers installed we can starting constructing the schema of our form.
const schema = yup.object({
// email is required with email format
email: yup.string().email().required(),
// phone number needs to match the regex expression
phoneNumber: yup
.string()
.matches(
/^((\\+[1-9]{1,4}[ \\-]*)|(\\([0-9]{2,3}\\)[ \\-]*)|([0-9]{2,4})[ \\-]*)*?[0-9]{3,4}?[ \\-]*[0-9]{3,4}?$/,
"Enter a valid phone number"
),
firstName: yup.string().required(),
lastName: yup.string().required(),
referral: yup.string(),
// password is required with minimum length of 8
password: yup.string().min(8).required(),
});
You can see yup is doing the majority part of our job, we are just instructing it how to do it. We have just defined the regex for the phone number and that is it. Now it is time to bind our yup schema with react hook forms, that’s a one-liner as well 🤯.
import { yupResolver } from "@hookform/resolvers/yup";
... rest of your component
const {
register,
handleSubmit,
watch,
formState: { errors },
} = useForm({
resolver: yupResolver(schema),
});
THAT IS IT. TRUST ME.
MOMENT OF TRUTH 🚀🚀
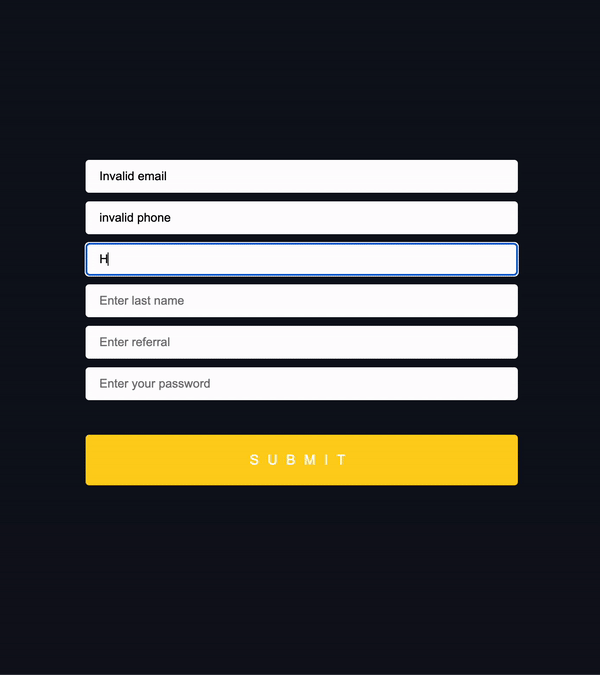
Wrap Up
Honestly, I think this one was a banger 💥💥. Such clean forms, quick validation, easy to integrate, and ready in no time. I think it is really about finding the right combination. Anyway, I hope you learned a lot from this one and I’m not letting you go since this is not a goodbye. See you at the next one. Take care.
💬 Leave a comment