TypeScript
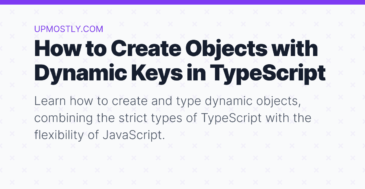
How to Create Objects with Dynamic Keys in TypeScript
Introducing TypeScript into your JavaScript app introduces some rigidity to your code – you lose the complete flexibility of JavaScript, but gain a lot of robustness and reliability in your code. As you learn TypeScript however, you’ll find a lot of ways to quickly build dynamic types – the Record [...]
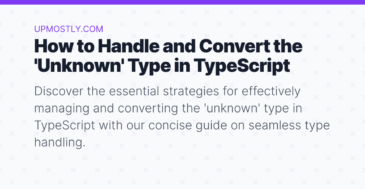
How to Handle and Convert the ‘Unknown’ Type in TypeScript
Hey there, TypeScript enthusiast! Working with TypeScript, chances are you’ve come across the intriguing “unknown” type. It’s like that enigmatic puzzle piece that doesn’t reveal its true nature until you’ve cracked the code. Well, consider this article your ultimate decoder ring, as we dive headfirst into the captivating realm of [...]
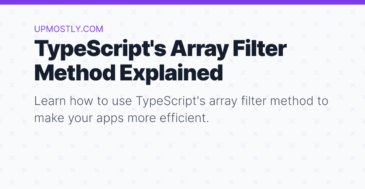
TypeScript’s Array Filter Method Explained
Welcome aboard, TypeScript enthusiasts! Today, we’re diving into the world of array filtering with TypeScript. Filtering arrays is a crucial skill every developer should have in their toolkit, allowing us to effortlessly sift through data, and extract exactly what we need. So, grab your favourite beverage, sit back, and let’s [...]
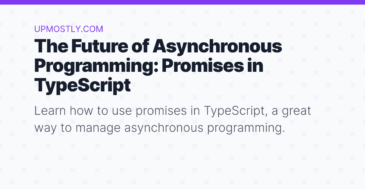
The Future of Asynchronous Programming: Promises in TypeScript
If you’re working with asynchronous code in TypeScript, you’ve probably run into Promises, an elegant solution to handling asynchronous tasks. With their clean syntax and powerful capabilities, promises have become an integral part of modern JavaScript development. TypeScript, being a statically typed superset [...]
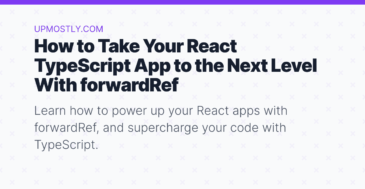
How to Take Your React TypeScript App to the Next Level With forwardRef
If you’re exploring the lesser-used features of React, you might come across forwardRef. It’s a method used to pass refs between parent and children components. In this article, we’ll talk through what refs are, forwardRef, and how to type forwardRef using [...]
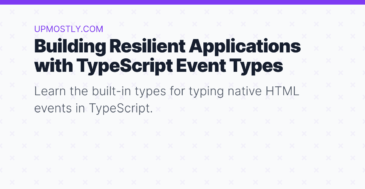
Building Resilient Applications with TypeScript Event Types
Event handling is an essential aspect of modern web development, enabling interactive user interfaces and dynamic content updates. However, ensuring type safety and preventing runtime errors can be a challenge when dealing with events in JavaScript. Luckily, TypeScript provides powerful features for typing event handlers and improving code reliability.
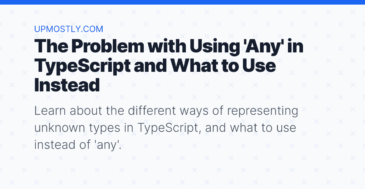
The Problem with Using ‘Any’ in TypeScript and What to Use Instead
If you’ve been using TypeScript for a while, you’re probably familiar with the “any” type. This type is often used as a placeholder for a value whose type is unknown or difficult to specify. While using “any” can make your code more flexible, it [...]
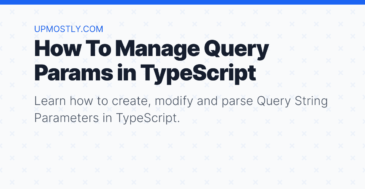
How To Manage Query Params in TypeScript
Have you ever found yourself working on a web application and needing to handle query parameters in a type-safe way? If so, you’re in luck! TypeScript provides powerful tools for working with query parameters that can help you catch bugs at compile time and write more maintainable code. If you’re [...]
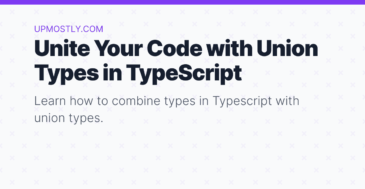
Unite Your Code with Union Types in TypeScript
One of the key features of TypeScript is the powerful ability to use union types. These types allow you to combine multiple types together in powerful ways, making it easier to work with complex data structures and functions. In this article, I’ll explain Union types, and when to use them.
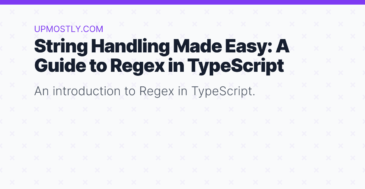
String Handling Made Easy: A Guide to Regex in TypeScript
If you’re working with Typescript and dealing with text processing, you might have heard of a powerful tool called “regex.” Regex, or regular expressions, is a pattern-matching language that allows you to search for specific patterns in text strings. It’s a versatile tool that can be used for a wide [...]
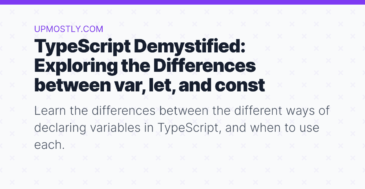
TypeScript Demystified: Exploring the Differences between var, let, and const
If you’re new to TypeScript, you might have noticed that there are three different keywords that you can use to declare variables: var, let, and const. While they might seem interchangeable at first, there are some important differences between them that you should be aware of. In this article, we’ll [...]
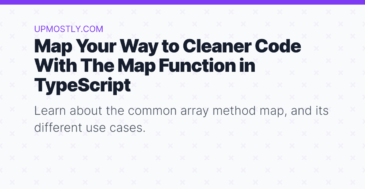
Map Your Way to Cleaner Code With The Map Function in TypeScript
If you’re working with arrays in TypeScript, you’ve probably come across the map() function. The map() function is a powerful tool that allows you to transform each element of an array into a new element, based on a function that you define. Here’s what the function looks like: const array: [...]
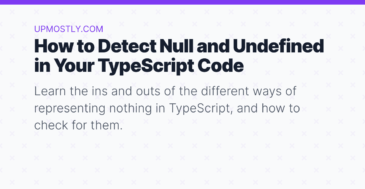
How to Detect Null and Undefined in Your TypeScript Code
Checking for null and undefined is a common task in TypeScript (and JavaScript in general), and there are several ways to do it. In this article, we’ll explore some of the most common techniques for checking null and undefined values in TypeScript. Equals The most straightforward way of checking is [...]
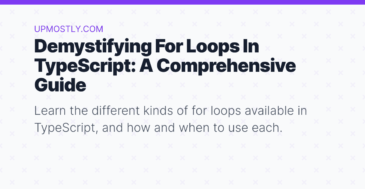
Demystifying For Loops In TypeScript: A Comprehensive Guide
Looping through an array is a common task in programming, and Typescript offers a variety of ways to accomplish this task. If you’re using React, you might be interested in this article on for loops in React. In this article, we’ll explore some of [...]
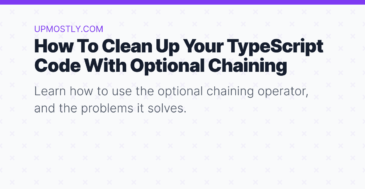
How To Clean Up Your TypeScript Code With Optional Chaining
Optional chaining is a powerful feature introduced in TypeScript 3.7. It lets you safely access properties and methods of an object without having to worry about the object being null or undefined. This can help prevent runtime errors and make code more concise and readable. The Problem One of the [...]
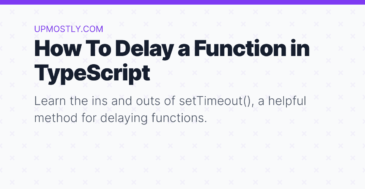
How To Delay a Function in TypeScript
When developing TypeScript applications, you may need to delay the execution of a function for a certain amount of time. Delaying function execution can be useful for a variety of reasons, such as waiting for an animation to complete or for an API request to finish. In this article, I’ll [...]
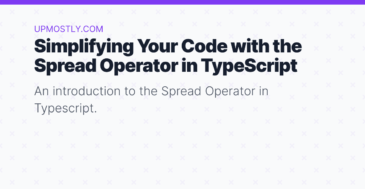
Mastering TypeScript’s Spread Operator for Cleaner, More Flexible Code
The spread operator in TypeScript is a powerful feature that allows you to easily expand the contents of an array or object. This can come in handy when you need to make a copy of an existing object or array, or when you want to [...]
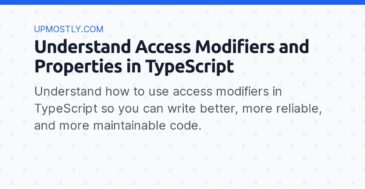
Understand Access Modifiers and Properties in TypeScript
Introduction Access modifiers are a feature of object-oriented programming languages that determine the accessibility or visibility of class members (properties and methods). In TypeScript, there are four access modifiers: public, private, protected, and readonly. These modifiers can be applied to properties, methods, and constructors to control how they can be [...]
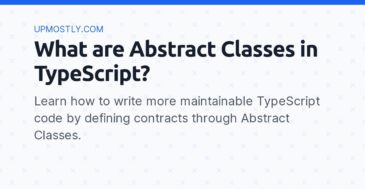
What are Abstract Classes in TypeScript?
Introduction Abstract classes are a powerful feature in TypeScript, a popular typed superset of JavaScript. They provide a way for developers to define the structure of a class without implementing all of its methods. In this article, we’ll go more in-depth to understand why, when, and how we would [...]
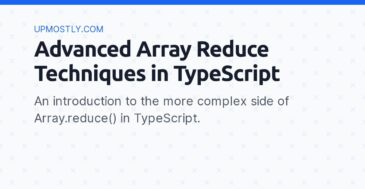
Advanced Array Reduce Techniques in TypeScript
The reducer method is a very useful array method. It lets you process an array item by item, reducing this to one variable. By default, the return type of the reducer is a single item of whatever your array returns. You can change the return type by providing a different [...]
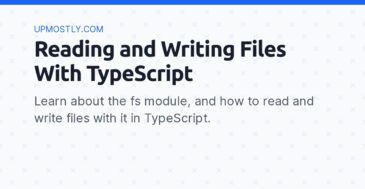
Reading and Writing Files With TypeScript
You can read and write files in TypeScript using the “fs” module, which is a Node module containing methods for file system operations. The module is very versatile and provides a lot of different ways of reading from and writing to files, so in this article, I’ll talk you through [...]
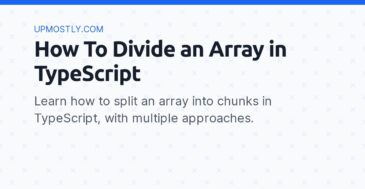
How To Divide an Array in TypeScript
Splitting an array into chunks might be a common problem you’ll run into in your TypeScript apps. In this article I’ll talk you through my approach, how that works, as well as the Lodash approach. Using Reduce One simple approach to this problem is to use [...]
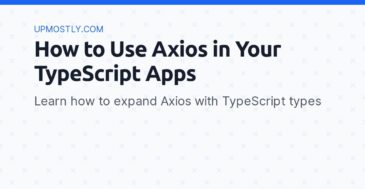
How to Use Axios in Your TypeScript Apps
Axios is a popular library for performing API calls in JavaScript-based apps, including TypeScript. Luckily Axios provides its own TypeScript typings with the library, meaning you don’t need any extra libraries for it to work with TypeScript. Today I’ll talk you through using TypeScript with Axios, by providing a type [...]
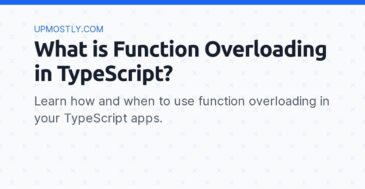
What is Function Overloading in TypeScript?
Function overloading is an OOP feature. It lets you define multiple functions with the same name, but different implementations. TypeScript has semi-support for function overloading; it allows you to to define multiple signatures for the same function, but only one implementation. This can still be useful, however, and in this [...]
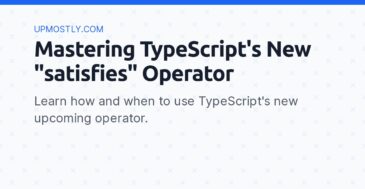
Mastering TypeScript’s New “satisfies” Operator
TypeScript 4.9 beta is introducing a new operator, the “satisfies” operator. The short version is that this operator lets you ensure a variable matches a type, while keeping the most specific version of that type. You can check out the discussion on the feature [...]
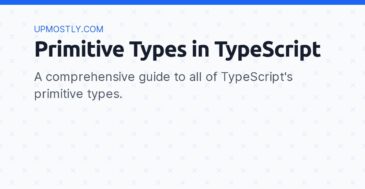
Primitive Types in TypeScript
TypeScript provides some useful primitive types that you’ll be using every day. In this article, I’ll explain them, what they’re for, and the TypeScript-specific primitives. number The number type works as you expect in TypeScript, covering all the ways JavaScript allows you to represent [...]
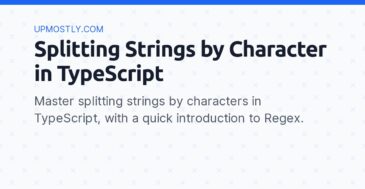
Splitting Strings by Character in TypeScript
There are a few scenarios you might want to be splitting strings in TypeScript, whether it’s splitting a paragraph by newline characters, splitting a list by commas, etc The .split() Function Let’s explore the .split() function using a file just containing some randomly generated [...]
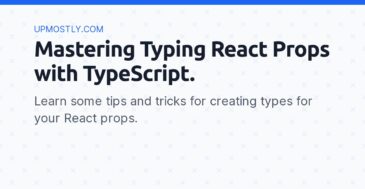
Mastering Typing React Props with TypeScript
TypeScript is a highly useful extension to JavaScript, that helps your code to be sound and robust. In this article, I’ll help you cover typing your React props in TypeScript, as well as some helpful common types to help you out. Interfaces vs [...]
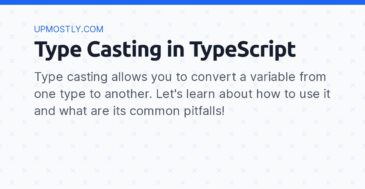
What is Type Casting in TypeScript?
Introduction As you might know, TypeScript gives us plenty of beautiful features that highly enhance the development experience, such as: Strong Static Typing supportSupport for major IDEsError highlighting at compile timeGreat integration with other tooling to provide hints Microsoft has continuously supported it back since 2012. Edge Scenario of Strong [...]
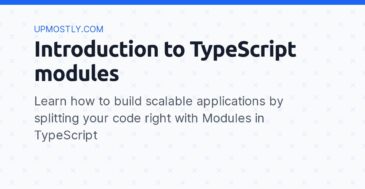
Introduction to TypeScript Modules
Keeping all code in one file is rarely a good practice, and we know this is especially true when working with React. However, if you have not yet worked with React, don’t worry, as the topic we’ll discuss about today is not directly [...]
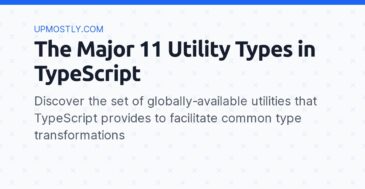
The Major 11 Utility Types in TypeScript
TypeScript provides a lot of utilities to help us write better, more scalable, better-structured, and overall safer applications. One of these utilities comes under the form of Utility Types, which we’ll discuss in this article. The concept of Utility Types in TypeScript is tightly tied with that of Generics, so [...]
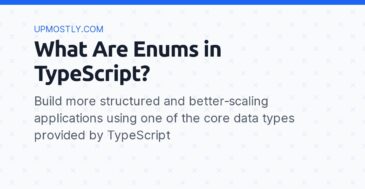
What Are Enums in TypeScript?
Introduction Sometimes rigid structure can allow us to build robust and solid foundations for modern applications, and TypeScript’s Enums will enable us to do precisely that. Since version 2.4 of TypeScript, we have been able to use Enums, a data type provided to us with configurability and “flexible rigidity” in mind. What are [...]
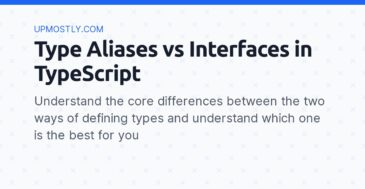
Type Aliases vs Interfaces in TypeScript
Introduction TypeScript gives us a handful of tools to ensure the best developer experience and application-level sustainability; two of these tools are Type Aliases and Interfaces. We’ve previously written articles on both of these ways of defining new named types; if you haven’t already checked those articles out, be sure [...]
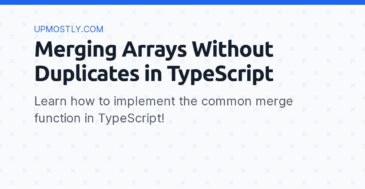
Merging Arrays Without Duplicates in TypeScript
Merging arrays is a common task you’ll face in most programming languages, especially merging arrays while only adding unique values. In this article, I’ll talk you through a few approaches to this in TypeScript, as well as the unique TypeScript quirks you might face. If you’re trying to solve this [...]
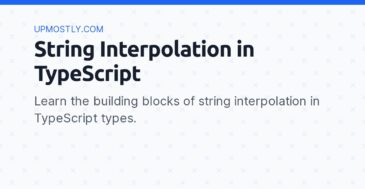
String Interpolation in TypeScript
TypeScript string literal types are a great tool that let you define a type as a literal string value. They’re also really useful when combined with type unions, commonly used as a sort of alternative to enums, when you just want to deal with strings. Here’s a simple example of [...]
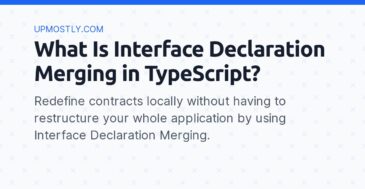
What Is Interface Declaration Merging in TypeScript?
If you aren’t already familiar with the concept of interfaces or what they are in TypeScript, be sure to check out this article first, as Interface Declaration Merging is linked directly to them. Otherwise, we shall proceed with the rest of the article. What [...]
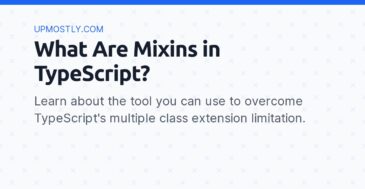
What Are Mixins in TypeScript?
If you’ve worked with TypeScript extensively, you would know that we cannot inherit or extend from more than one class at a time. However, this inconvenience is something we can get around by using something called Mixins. So, what exactly are Mixins? Introduction to TypeScript’s Mixins As mentioned prior, Mixins [...]
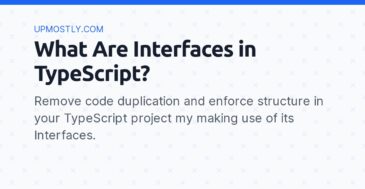
What Are Interfaces in TypeScript?
Similar to Type Aliases, TypeScript’s Interfaces provide us a medium to avoid code duplication across our TypeScript codebases. If we were to look at the alternative way of defining types, we would find ourselves defining and redefining types inline each time we want to [...]
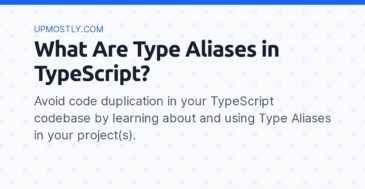
What Are Type Aliases in TypeScript?
We may find ourselves defining the same Type in different places, which is not ideal, considering all the bad things we often hear about code duplication, but what can we do? The answer to that is Type Aliases. TypeScript allows us to reuse certain types by defining them the same [...]
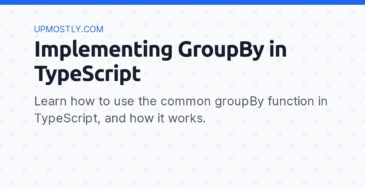
Implementing GroupBy in TypeScript
The groupBy function is generally used to group arrays together by a certain condition. You can easily go for a built-in solution, such as lodash’s groupBy function, or implement it yourself in TypeScript. Lodash _.groupBy Lodash is a library that contains a large selection of utility functions, [...]
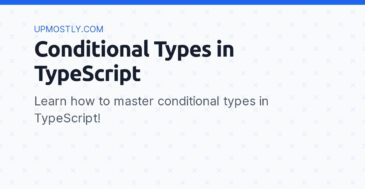
Conditional Types in TypeScript
Conditional types in TypeScript help you to create types depending on another type, by checking if that type satisfies some condition. In their simplest form, they take the format of: MyType extends SomeType ? IfTrueType : IfFalseType; Here’s a simple example, checking if a type can be [...]
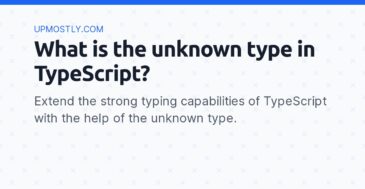
What is the unknown type in TypeScript?
Introduction Not so long ago, in version 3.0, TypeScript introduced a new `unknown` type to its toolkit, but what does this type do, and more specifically, when should we use it? This article will look at this “new” type to better understand its goal. We’ll also consistently compare it to [...]
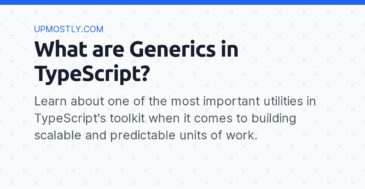
What are Generics in TypeScript?
One of the most important things when writing scalable applications is ensuring that the components that make up those applications are scalable and, thus, reusable by themselves. When working with TypeScript, one of the utilities that ensure units of work are reusable and scalable is Generics. Introduction to TypeScript’s Generics [...]
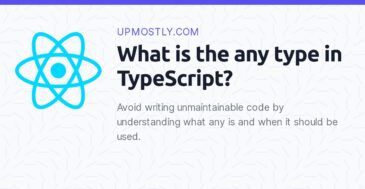
What is the any type in TypeScript?
When working with TypeScript or migrating an already existing codebase over to TypeScript, it is crucial to know how to handle typing properly. One tricky typing scenario to get right is when we decide whether or not to use any for a particular scenario. In this article, we’ll discuss about [...]
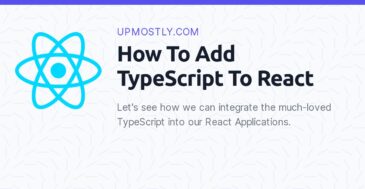
How To Add TypeScript To React
As JSX projects get increasingly complex, we often find ourselves reverse-engineering whole chunks of code to fix or change existing functionality. JavaScript doesn’t really help in that regard, especially when trying to figure out what specific code snippets do unless they have been run first. Intro to TypeScript Suppose you [...]
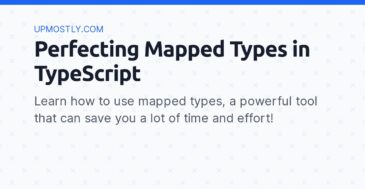
Perfecting Mapped Types in TypeScript
Mapped Types in TypeScript let you transform one type to another, by transforming the keys from one type to another. TypeScript offers a lot of flexibility with this, allowing you to modify the name of types, perform string interpolation on keys and more. In [...]
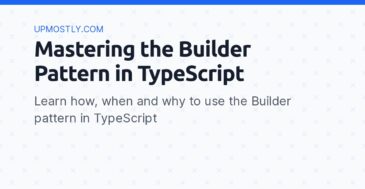
Mastering the Builder Pattern in TypeScript
The builder pattern in TypeScript is used to give a more flexible solution to creating complex objects, by breaking the construction up into separate steps. An example of this is the Promise class, which you may be familiar [...]