Have you ever found yourself working on a web application and needing to handle query parameters in a type-safe way? If so, you’re in luck! TypeScript provides powerful tools for working with query parameters that can help you catch bugs at compile time and write more maintainable code. If you’re using Next.js, you might also be interested in this article. In this article, we’ll explore how to use TypeScript to work with query parameters and discuss some best practices for handling them in your web applications. So grab a cup of coffee, get comfy, and let’s dive in!
qs
One option to approach this is to use the package “qs”, which you can install with:
npm install --save qs
npm i --save-dev @types/qs
This package provides a set of utility functions for parsing and formatting query strings.
One thing the package does not do however, is extract the params from a URL, but we can do this very simply:
const url = 'https://upmostly.com/wp-admin/post.php?post=17693&action=edit';
const [, params] = url.split('?');
Splitting the URL by the question mark means we get an array with two items; everything before the params, and everything after. We can then use destructuring to directly get everything after, without even storing the URL part we don’t need.
Parsing the parameters we’ve extracted is easy:
const parsedParams = qs.parse(params);
console.log(parsedParams); //{ post: '17693', action: 'edit' }
qs also supports the reverse; creating query params from TypeScript:
const myParams = qs.stringify({ post: '17693', action: 'edit' }); //post=17693&action=edit
URLSearchParams
If you want to avoid using an external package, you can also do this using the built-in URLSearchParams API.
Let’s rewrite the previous solution to use these:
const url = new URL(
'https://upmostly.com/wp-admin/post.php?post=17693&action=edit'
);
const params = url.searchParams;
console.log(params); //URLSearchParams { 'post' => '17693', 'action' => 'edit' }
One important thing to note here is that instead of an object, you’ll get back a URLSearchParams.
You can easily iterate through this:
for (const [key, value] of params) {
console.log(key, value);
}
Or convert it to an array of entries using:
const paramsArray = [...params];
//[ [ 'post', '17693' ], [ 'action', 'edit' ] ]
Or just the keys or values:
const keys = [...params.keys()];
const values = [...params.values()];
console.log(keys, values); //[ 'post', 'action' ] [ '17693', 'edit' ]
Constructing Query Params
We’ve covered parsing query parameters using URLSearchParams, but how do we create them?
The URLSearchParams constructor provides this functionality:
const newParams = new URLSearchParams({ post: '17693', action: 'edit' });
const paramsString = newParams.toString();
console.log(paramsString); //post=17693&action=edit
Then to construct our URL back together, you can do it manually:
const url = 'https://upmostly.com?' + paramsString;
Or through the URL API if you plan to do more modifications to the URL:
const newUrl = new URL('https://upmostly.com?'+ paramsString);
Conclusion
Thanks for reading! I hope with the help of this article, you have all the knowledge needed to create, modify and read query string parameters in TypeScript. Which approach you use is up to you. The API provided by qs is a little simpler, at the cost of an extra package. URLSearchParams however is a great native option, with some extra functionality.
If you liked this article, or if you have any questions, leave a comment below!
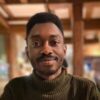
💬 Leave a comment