We have learned how to make the most out of Axios and react query but it is about time we work on the structure side of it to improve our code readability. In this article, we are going to inject react query in a much cleaner way so that everybody can get your code at first glance. Without wasting any time let’s dive straight into it.
Scaffolding
We scaffold our project in the following manner
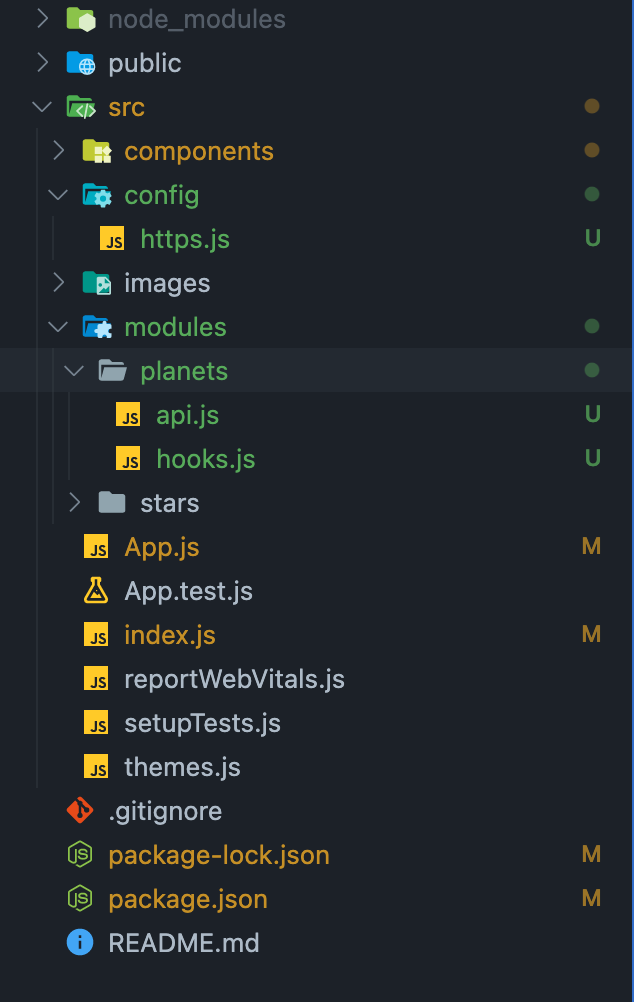
Under config/https.js we have our Axios configuration. And with the modular approach under modules, we have our API and custom hooks serving the single responsibility principle. With our base URL in configs we have our modular route inside modules/planets/api.js as
import { axiosInstance } from "../../config/https";
const fetchPlanets = async () => {
return axiosInstance({
url: "planets",
method: "GET",
}).then(({ data }) => {
return data;
});
};
export { fetchPlanets };
and under modules/planets/hooks.js are our custom hooks which consumes API layer like
import { useQuery } from "@tanstack/react-query";
import { fetchPlanets } from "./api";
const usePlanets = () => {
return useQuery(["get-planets"], fetchPlanets, {
select: (data) => data,
});
};
export { usePlanets };
Consuming Custom Hooks
Now that our custom hook is ready we can consume it in our custom component as
function CustomComponent() {
const [planets, setPlanets] = useState([]);
const { data, isLoading } = usePlanets();
useEffect(() => {
setPlanets(data);
}, [data]);
return (
{isLoading ? (
<InfinitySpin width="500" color="blue" />
) : (
<PlanetList
planets={planets}
classNames={classNames}
/>
)}
)
}
The Big Reveal 🥳🥳
Incoming Bobs your uncle…🤓
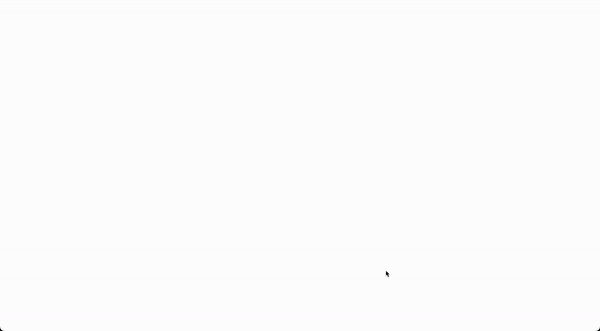
Wrap Up 🧳
This is it from this one, I hope the next time you try to get some data from your server you do it in a cleaner manner and with react query since it is clearly fast and easy to integrate. This is not a goodbye keep scrolling through upmostly and keep learning new things.
💬 Leave a comment