In this article, we are going to learn how to cleanly set up Axios for code readability and reusability. We have previously learned how to set up Axios in our reactJS application. So without wasting any time let’s dive straight into it.π€Ώπ€Ώ
Introduction
As we learned how to send HTTPS requests and receive responses when we communicate with our server for data and had to use the deconstructed data from the response every time. What if I told you Axios provides you with a built-in mechanism to handle all of this hassle in a much cleaner way? π§
Interceptors In Axios
With Axios interceptors, you can now intercept and hook into requests and responses before they are treated by the then() or catch() block. Let’s see them in action by making https.js under config directory like this
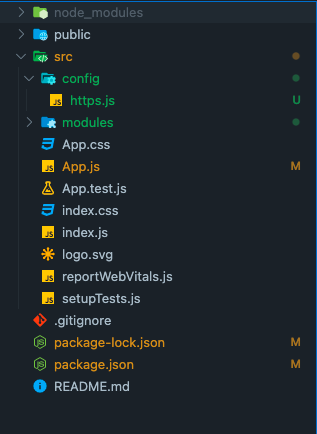
And now writing the code to use interceptors in Axios
import axios from "axios";
export const axiosInstance = axios.create({
baseURL: "https://swapi.dev/api/",
});
axiosInstance.interceptors.request.use(
function (config) {
// Do something before request is sent
return config;
},
function (error) {
// Do something with request error
return Promise.reject(error);
}
);
axiosInstance.interceptors.response.use(
function (response) {
return response;
},
function (error) {
return Promise.reject(error);
}
);
Here we have created interceptors for our Axios instance which handles the requests and responses which makes our code reusable as well as readable.
Interceptors also allow us to add or customise our headers in the request by doing something like
axiosInstance.interceptors.request.use(
async (config) => {
const token = # Your token goes over here;
if (token) {
config.headers.accessToken = token;
}
return config;
},
function (error) {
return Promise.reject(error);
}
);
Bobs Your Uncle π
We already knew how to use Axios in our reactJS application, in this one covered how to make the most out of Axios by using interceptors.
Reference
https://axios-http.com/docs/interceptors
π¬ Leave a comment