We have previously talked about why we want to use react query with our server-side state and this time we are here to walk the walk. In this article, we are going to learn how easy it is to get up and running with react query with your application.
Why React Query?
- Allows you to update data only when it is required or even manually
- Know when your data is out of date
- Managing server-side seamlessly
- Easiest strategy which can be the most difficult most of the time, Caching
- Easy lazy loading
- Pagination support
- No boilerplate code
- Ready to be consumed
- Better performance of your application
React Query in Action
Following our Axios with reactJS introduction guide, we try to communicate with our server using react query and axios. We separate our REST API into a separate layers to have a single responsibility and make it look like this.
const fetchPlanet = async () => {
return axiosInstance({
url: "planets/1/",
method: "GET",
}).then(({ data }) => data);
};
And using react query to pass this function as an argument, an argument which is required by react query which returns back a promise.
const { isLoading, error, data } = useQuery(["repoData"], fetchPlanet);
BOOOOOM 🚀🚀🚀. That was fast right? And look at how much boilerplate code we just skipped and have everything we need in just one response from our query. We can further use isLoading, error and data however we want in our component.
if (isLoading) return "Loading...";
if (error) return "An error has occurred: " + error.message;
return (
<div>
<h1>Name: {data.name}</h1>
<h1>Climate: {data.climate}</h1>
<h1>Diameter: {data.diameter}</h1>
<h1>Gravity: {data.gravity}</h1>
<h1>Terrain: {data.terrain}</h1>
<h1>Population: {data.population}</h1>
<h1>Orbital Period: {data.orbital_period}</h1>v
</div>
Cleaner Code All the Way
Once you are done with this minimalistic code you realize the power of Axios and react query in combination. It’s basically a killer duo 🥵🥵.
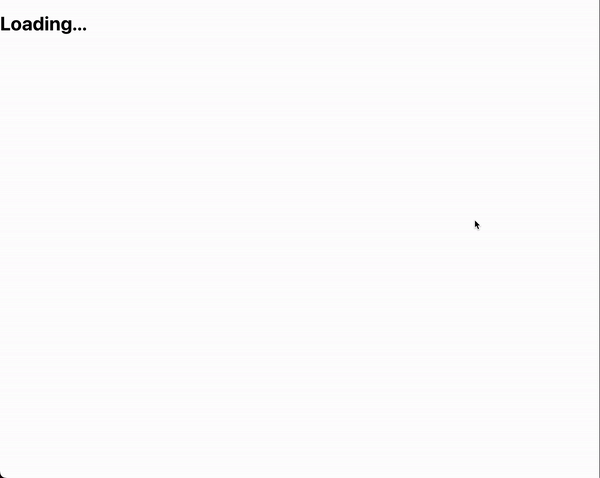
Wrap Up
In this article, we learned how to efficiently communicate with our server in less code following best practices. I hope you managed to keep up with this one because react query is although hard to grasp, but once you get going there is no stopping you.
Reference
💬 Leave a comment