In this article, we are going to learn how to request data from your backend in a certain format through react query. We are going to get data and serve it to our custom components as they require them. Without further ado let’s begin.
Transformer Layer
Let’s take the scenario that you are working on your ReactJS application and requesting payload from your backend with REST and you want the keys in your JSON transformed in a manner that is easy for you to plugin in your component. This is where the transformer layer jumps in. The question then comes in where should we do our transformation? The backend or the frontend? Well, there is no right or wrong here. It depends on the type of your problem. Let’s take an example, you requested a single planet from your backend and this is the response that you got
{
"name": "Yavin IV",
"rotation_period": "24",
"orbital_period": "4818",
"diameter": "10200",
"climate": "temperate, tropical",
"surface_water": "8",
"created": "2014-12-10T11:37:19.144000Z",
"edited": "2014-12-20T20:58:18.421000Z",
"url": "https://swapi.dev/api/planets/3/"
}
but your custom component was expecting something like this
{
"name": "Yavin IV",
"rotationPeriod": "24",
"orbitalPeriod": "4818",
"diameter": "10200",
"climate": "temperate, tropical",
"surfaceWater": "8",
"createdAt": "2014-12-10T11:37:19.144000Z",
"editedAt": "2014-12-20T20:58:18.421000Z",
"link": "https://swapi.dev/api/planets/3/"
}
- rotation_period ➡ rotationPeriod
- orbital_period ➡ orbitalPeriod
- surface_water ➡ surfaceWater
- created ➡ createdAt
- edited ➡ editedAt
- url ➡ link
Where To Achieve This?
The big question is where can we or should we transform it?
- Could be on the backend
- Could be inside the query function
- Could be inside the render of the component
- Or inside the select option in react query
Select With React Query
We will use react query selectors to transform our raw data in any form that our component prefers. We have added transformer.js inside our modular approach.
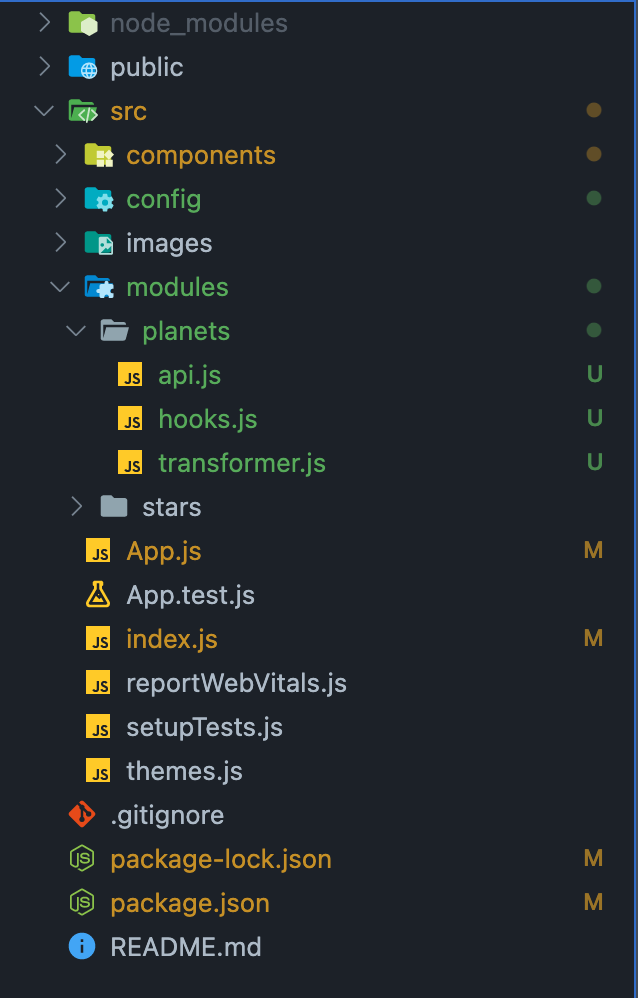
Now coming to the actual transformation
function transformPlanet(planet) {
let {
name,
rotation_period,
orbital_period,
diameter,
climate,
surface_water,
created,
edited,
url,
} = planet;
return {
name,
rotationPeriod: rotation_period,
orbitalPeriod: orbital_period,
diameter,
climate,
surfaceWater: surface_water,
createdAt: created,
editedAt: edited,
url,
};
}
function transformPlanetArray(planets) {
return planets && planets.map((planet) => transformPlanet(planet));
}
export { transformPlanetArray, transformPlanet };
BOBS YOUR UNCLE 🚀🚀
const usePlanets = () => {
return useQuery(["get-planets"], fetchPlanets, {
select: (data) => transformPlanetArray(data),
});
};
WE HAVE DONE IT AGAIN. Good code practice and transformed our incoming data with minimal code while being performance efficient.
Wrap Up
Well this one might have been short but it sure was very technical, I hope I didn’t lose you in the middle and even if you have any questions you can ask us below in the comment box. Don’t hesitate. Here’s to the CLEANER CODE! 📣🥂
💬 Leave a comment