What Is a Query String?

A query string is a method of passing variables to a URL, in the form of a list of key-value pairs, beginning with “?”, and separating each following “key=value” pair with an “&”. In the URL above we can see we have the variable “variable” with the value “foo”, and the variable “name” and the value “bar” They’re commonly used for things like sending the results of a HTML form to a URL, or sending any small amount of data.
They’re used across the front-end and back-end of a website, and in Next.js there are two ways of defining a component for the front-end, so we’ll go through all 3 scenarios in which you might be trying to access query string parameters.
Working With Query Strings in Next JS
To access query string parameters in Next.js we can use the useRouter hook provided by NextJS. The router object it returns includes the router.query property, containing the parameters we’re looking for. Take a look at this quick example:
import { useRouter } from 'next/router';
export default function Example() {
const router = useRouter();
const { name, location } = router.query;
const query = router.query;
return (
<div>
<h1>
Hi! I'm <span>{name}</span>
</h1>
<h2>Location: {location}</h2>
<h2>Age: {query.age}</h2>
<h2>Title: {query.title}</h2>
</div>
);
}
And what this NextJS page renders from this URL :
http://localhost:3005/example?name=Omari&location=England&age=57
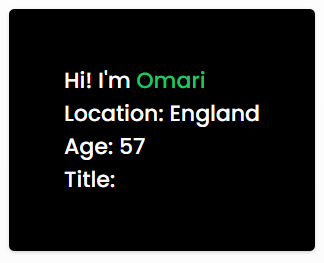
router.query gets populated with the query string parameters from the URL. You can access these like any normal object property with router.query.property, or we can use object destructuring to make our code a little neater. Any value that isn’t provided as a query string parameter will show as undefined, which explains why the title shows as blank. (N.B. If you’re wondering what those weird class names are and where the styling is coming from, check out our page on TailwindCSS here!)
Class Components Alternative
Since useRouter is a hook, it cannot be used inside class components. Functional components are strongly recommended over class components for React, and you can find out more about the differences between them here. In case you still need to use a class component, however, there is a way in the withRouter HOC, or higher-order component. If you’re unfamiliar with those, head here to find out more about them.
Here’s the equivalent code for the above using class components:
import { withRouter } from 'next/router';
import { Component } from 'react';
class ClassComponentExample extends Component {
render() {
const router = this.props.router;
const { name, location, age, title } = router.query;
return (
<div>
<h1>
Hi! I'm <span>{name}</span>
</h1>
<h2>Location: {location}</h2>
<h2>Age: {age}</h2>
<h2>Title: {title}</h2>
</div>
);
}
}
export default withRouter(ClassComponentExample);
The code is mostly similar, however since we can’t use hooks within class components, the HOC withRouter passes the router object to the component as a prop. After that, the code ends up mostly the same!
Query Strings in the Back-End
Next.js also provides a built-in solution for developing a back-end API, and we can define routes for our API by a function that takes a request and response parameter. You can access any query parameters present in the request through the req.query property.
export default function handler(req, res) {
res.status(200).json(req.query);
}
Then we can see the response we get back:

Just like through the front-end with useRouter(), we get an object back containing all of the query parameters provided, which you can then use however you need!
Thanks for reading, hopefully, that all made sense! Feel free to leave a comment below if you have any queries or suggestions, or if you simply want to let me know that you liked this article!
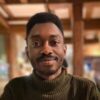
💬 Leave a comment