If you’re working with Typescript and dealing with text processing, you might have heard of a powerful tool called “regex.” Regex, or regular expressions, is a pattern-matching language that allows you to search for specific patterns in text strings. It’s a versatile tool that can be used for a wide range of text processing tasks, from validating user input to extracting data from large text files. In Typescript, you can use the built-in RegExp class to work with regular expressions.
What is Regex?
Regular expressions are expressions that can be used to describe a set of rules or patterns that match specific text strings or characters. This can be useful for tasks such as searching for and extracting specific information from large text files, or validating user input to ensure it meets a specific pattern or format.
Let’s take a look at an example Regex pattern:
/0x[A-F0-9]+/gi
Looks like a bunch of gibberish, right? But underneath each symbol has a meaning. This regex pattern is equivalent to “A string starting with ‘0x’, then followed by at least 1 character which is either A-F or 0-9”, aka a hexadecimal string! There are a lot of different Regex characters, and you can check out the full list here.
Simple
We’ll start off simple. Let’s imagine I want some regex to remove my name from a message. Firstly we need some regex to describe “text that is ‘Omari'”:
const nameRegex = /Omari/gi;
Pretty simple. The characters you see at the end are option flags, g is for global, meaning “check for all matches, not just the first”.i is for case insensitive, meaning ignore capitalisation.
Then let’s use our Regex:
const message = 'Hello Omari, your name is Omari right? Great name, Omari!';
const anonymized = message.replace(nameRegex, '[NAME REMOVED]');
console.log(anonymized);
The TypeScript string class includes a .replace method, which accepts a regex pattern as a parameter. And here’s our output:

Intermediate
Let’s get a little more advanced. Regex is used a lot for pattern-matching filenames. Let’s take a look at this mock project directory:
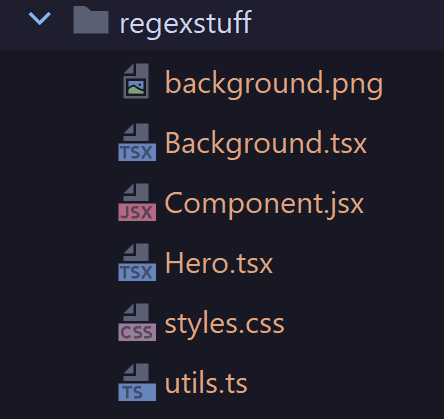
And let’s write some Regex to be able to get every TypeScript file from this folder, that’s every file that ends in “.ts” or “.tsx”.
Firstly we’ll get our folder in code:
const files = fs.readdirSync('./regexstuff');
console.log(files);
/*
[
'Background.tsx',
'Component.jsx',
'Hero.tsx',
'background.png',
'styles.css',
'utils.ts'
]
*/
Then we need some way of checking if a string matches a Regex pattern, thankfully we can use the .test() method on Regex patterns:
const regex = //?
const tsFiles = files.filter((f) => regex.test(f));
console.log(tsFiles);
Then we need to fill in our Regex. Here’s my solution:
const regex = /.*\.tsx?/gi;
Let’s break this down:
- . – Any character except for newline characters
- * – Any number of the preceding characters
- “.ts”, including a backslash to escape the “.”, so it’s interpreted as a full stop.
- x?, meaning 0 or 1 “x” character
- gi, our flags:
- g – Global, meaning to search for any matches in our string
- i – Case insensitive again
So our whole Regex means “Any number of any characters, followed by .ts, and optionally an x”
Advanced
This time we’ll do something a little more difficult. Let’s try and use regex to extract query string parameters from our URL, and even split them into variable names and values. If you’re not sure what query string parameters are, they’re a method of passing variables to a URL, in the form of a list of key-value pairs, beginning with “?”, and separating each following “key=value” pair with an “&”.
For example, here’s the query string parameters passed to the very post you’re reading right now:

What we want for our end result is to extract the parameters, and have them grouped together in key value pairs.
Here’s the code:
const querystring = /(?<=\?|&)(\w+)=(\w+)/gi;
const matches = [...url.matchAll(querystring)].map((m) => [m[1], m[2]]);
console.log(matches);
First let’s explain our regex:
- (?<=\?|&)
- (?<=) is a positive lookbehind. It’s used to check for whatever else is in the brackets, without including it in the match. In our case, we don’t want to include the ? or & in a match, but we do know a variable will follow it.
- \? | & is simple, it means ? or &. We also have to escape our question mark, so it’s interpreted as a literal question mark.
- (\w+)
- \w means any alphanumeric character
- + means 1 or more of them
- = – We’re looking for an equals between our two matches
- Then we have another w+ to match the next word
Then the matchAll method gives us back an iterator, which we can spread into an array. We can then use map to extract the information we want from each match, and our end result is:

Conclusion
To wrap it up, Regex is a robust feature in TypeScript that allows you to effectively handle and validate strings in your code. With the knowledge of regex syntax and TypeScript’s built-in support, you can simplify your string manipulation and reduce your code’s complexity.
Thanks for taking the time to read this article on regex in TypeScript. If you liked this article, or if you need any help, leave a comment below. I hope it’s been informative and I’ve helped you broaden your understanding of this crucial concept!
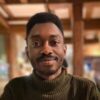
💬 Leave a comment