Looping through an array is a common task in programming, and Typescript offers a variety of ways to accomplish this task. If you’re using React, you might be interested in this article on for loops in React.
In this article, we’ll explore some of the different approaches to looping through an array in Typescript, and highlight their unique features and benefits.
For Loops
The traditional for loop is one of the most commonly used looping constructs in programming, and it works just as well in Typescript. With a for loop, you can iterate through each element of an array and perform some operation on each element.
const myArray = [1, 2, 3, 4, 5];
for (let i = 0; i < myArray.length; i++) {
console.log(i, myArray[i]);
}
The benefits of for loops are speed; they’ve existed for so long that they’re highly optimised in most programming languages, as well as versatility. You have complete control over the iteration, when it ends, and how it iterates. This means you can do complex things like:
Iterate through a linked list:
type LinkedListNode = { val: number; next: LinkedListNode | null };
const node: LinkedListNode;
for (
let curr: LinkedListNode = node;
curr.next === null;
curr = curr.next ?? curr
) {
console.log(curr.val);
}
For Each
Arrays in TypeScript have many different methods attached to them, one of them being the forEach() method. With forEach, you provide an iterator function which TypeScript calls for each element in the array, providing it with the index, the element and the whole array as parameters.
myArray.forEach((value, i, array) => console.log(i, value));
It’s a little more concise than the for loop, which you may prefer in a lot of situations.
Additionally, since it accepts a function as a parameter, this means you can abstract out the iteration logic into a separate function, into something like:
for (let i = 0; i < myArray.length; i++) {
//some big block of code
}
//put it into a function
myArray.forEach(someBigFn);
For Of
The for of loop is a newer addition to Javascript, and is available in Typescript as well. With a for of loop, you can iterate through each element of an array without needing to worry about the index, just like the for each loop.
for (const element of myArray) {
console.log(element);
}
One important thing to note here is that with the for of loop, you don’t have access to the array index.
For In
For In is a special loop used to iterate through object keys. It’s identical to the for of syntax, just swapping the “of” for an “in”:
const myObj = { foo: 1, bar: 'Hi!' };
for (const key in myObj) {
console.log(key, myObj[key as keyof typeof myObj]);
}
One small note here is that you’ll need to cast the key to something that can be used to index your object.
And of course, the same thing can be accomplished using a for of loop:
for (const [key, value] of Object.entries(myObj)) {
console.log(key, value);
}
Which one to use?
For most situations, you can feel free to pick whichever option you prefer. The for loop can be a great option if you prefer a simpler, iterative approach, while forEach lends well to a more functional style of programming. forEach is slower than the declarative approaches, however, so you might prefer to use “for..of” loop if you need the simpler syntax, but require more speed.
To sum it up:
For Loop
- Fast
- Simple
- Can be used for more complex iteration
- Everything is manual, so slightly more error-prone
forEach
- Shorter syntax
- Functional style
- Can’t break or continue
For Of
- Faster than forEach
- Shorter syntax than for loop
For In
- Useful for object keys
- (Only useful for object keys)
If you’re not sure which one to use, I tend to start off with a for loop, and then see if the code can be shortened with one of the alternate options.
Conclusion
Thanks for reading! There are a lot of different ways to loop through an array in Typescript, each with its own strengths and weaknesses. Depending on your specific use case, you may find that one approach works better than another.
Whether you choose a traditional for loop, a concise for each loop, a modern for of loop, a flexible while loop, or a do-while loop, you can rest assured that you have the tools you need to efficiently and effectively iterate through your array!
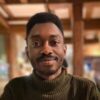
💬 Leave a comment