Introduction
Web development is an ever-evolving landscape, with JavaScript frameworks playing a significant role in modern web applications. As a developer looking to stay relevant and ahead of the curve, mastering these frameworks is essential. In this article, we will provide you with a comprehensive learning path to help you navigate the world of JavaScript frameworks, such as React, Angular, and Vue.js, and set you on the path towards mastery. We’ll cover everything from building a strong foundation in JavaScript to exploring each framework, building real-world projects, and staying current with industry trends. So grab your favorite text editor, a cup of coffee, and let’s dive in!
Building a Strong Foundation in JavaScript
Building a Strong Foundation in JavaScript
Before you can truly master JavaScript frameworks, it’s crucial to have a solid understanding of JavaScript itself. Having a strong foundation in the language will make learning frameworks a smoother experience and enable you to unlock their full potential. In this section, we’ll go over some essential JavaScript concepts and techniques you should master, as well as recommend resources for further learning.
- Variables, Data Types, and Operators: Learn about declaring variables, working with different data types (strings, numbers, booleans, etc.), and using various operators (arithmetic, comparison, logical, etc.).
- Control Structures: Understand how to use conditional statements (if, else, switch), loops (for, while, do-while), and error handling (try, catch, finally).
- Functions: Master the concepts of function declaration, parameters, arguments, return values, and scope. Explore arrow functions and understand the difference between regular functions and arrow functions.
- Objects and Arrays: Dive into the world of objects, properties, and methods, as well as arrays and their manipulation methods (push, pop, splice, etc.).
- Asynchronous JavaScript: Learn about callbacks, Promises, and async/await to manage asynchronous operations effectively.
- DOM Manipulation: Get comfortable with selecting, creating, modifying, and deleting HTML elements using JavaScript, as well as handling events and event listeners.
- ES6+ Features: Familiarize yourself with the latest JavaScript features, such as let and const, template literals, destructuring, default parameters, and more.
Recommended Resources for Learning JavaScript:
- Mozilla Developer Network (MDN) Web Docs: MDN is an excellent resource for learning and referencing JavaScript concepts, providing thorough documentation, guides, and examples.
- Eloquent JavaScript by Marijn Haverbeke: This free online book is a comprehensive guide to JavaScript, covering fundamental concepts and diving into more advanced topics.
- You Don’t Know JS (book series) by Kyle Simpson: This in-depth book series covers a wide range of JavaScript topics, from the basics to advanced concepts, providing a deep understanding of the language.
- FreeCodeCamp: FreeCodeCamp offers an interactive JavaScript curriculum, complete with hands-on coding challenges and projects to help solidify your understanding.
Diving into React: A Beginner’s Guide
React is a popular JavaScript library developed by Facebook for building user interfaces. It’s known for its component-based architecture, which promotes reusability and maintainability. In this section, we’ll introduce you to essential React concepts and walk you through the process of building your first React app.
- React Components: Understand the concept of components, the building blocks of a React application, and learn how to create functional and class components.
- JSX: Familiarize yourself with JSX, a syntax extension that allows you to write HTML-like code within your JavaScript code, making it easier to define and manipulate the structure of your components.
- State and Props: Learn about managing component data using state and props, as well as the differences between them and when to use each.
- Event Handling: Explore handling user interactions, such as clicks or form submissions, by attaching event listeners and defining event handler functions.
- Lifecycle Methods: Get to know the lifecycle methods of class components, which allow you to perform actions at different stages of a component’s life, such as mounting, updating, and unmounting.
- Hooks: Discover React Hooks, a powerful feature introduced in React 16.8 that enables you to use state and lifecycle features in functional components.
- Routing: Understand how to implement client-side routing in your React app using React Router, allowing you to create seamless navigation experiences.
- State Management: Learn about advanced state management solutions, such as Redux or MobX, to handle complex application state and improve performance.
Building Your First React App
- Set up your development environment by installing Node.js and npm (Node Package Manager).
- Install the Create React App CLI tool using
npm install -g create-react-app
. - Create a new React project by running
create-react-app my-first-react-app
(replace “my-first-react-app” with your desired project name). - Navigate to the project directory using
cd my-first-react-app
and start the development server usingnpm start
. - Open your favorite code editor and explore the project structure. Modify the
src/App.js
file to create your first component. - Add interactivity to your component by handling user events and managing state.
- Experiment with additional components, state management, and routing to build a more complex app.
Recommended Resources for Learning React (apart from Upmostly of course :P):
- Upmostly (of course 😛)
- Official React Documentation: The official documentation is an excellent resource for understanding React concepts, with guides, tutorials, and API references.
- React for Beginners by Wes Bos: This paid course offers a comprehensive introduction to React, covering essential concepts and building a real-world project.
- Fullstack React: The Complete Guide to ReactJS and Friends: This book provides an in-depth look at React and its ecosystem, including Redux, React Router, and other essential libraries.
- Scrimba: Learn React for free: This interactive platform offers a free course on React, complete with hands-on coding exercises and challenges.
Exploring Angular: A Beginner’s Guide
Angular is a powerful and feature-rich JavaScript framework developed by Google, primarily used for building single-page applications (SPAs). It promotes a modular architecture and provides a robust set of tools and features to streamline web development. In this section, we’ll introduce you to essential Angular concepts and guide you through the process of building your first Angular app.
- Angular Modules: Learn about Angular modules, which are the building blocks of an Angular application, and how they help organize and modularize your code.
- Components and Templates: Understand Angular components, their associated templates, and how to create and use them in your application.
- Data Binding: Familiarize yourself with the different types of data binding in Angular, such as interpolation, property binding, and event binding, to create dynamic and interactive user interfaces.
- Directives: Discover Angular directives, which are used to manipulate the DOM and add custom behaviors to elements.
- Services and Dependency Injection: Learn about Angular services, which are used to encapsulate reusable logic, and the dependency injection mechanism that allows you to easily provide services to components.
- Routing: Explore Angular’s built-in routing module, which enables you to create sophisticated navigation structures for your SPA.
- Forms: Get comfortable with Angular’s form handling capabilities, including both template-driven and reactive forms.
- HTTP Client: Understand how to make HTTP requests and interact with APIs using Angular’s built-in HttpClient module.
Building Your First Angular App
- Set up your development environment by installing Node.js and npm (Node Package Manager).
- Install the Angular CLI using
npm install -g @angular/cli
. - Create a new Angular project by running
ng new my-first-angular-app
(replace “my-first-angular-app” with your desired project name). - Navigate to the project directory using
cd my-first-angular-app
and start the development server usingng serve
. - Open your favorite code editor and explore the project structure. Modify the
src/app/app.component.ts
andsrc/app/app.component.html
files to create your first component. - Add interactivity to your component by implementing data binding and event handling.
- Experiment with additional components, services, routing, and forms to build a more complex app.
Recommended Resources for Learning Angular:
- Upmostly Angular Category
- Official Angular Documentation: The official documentation is an invaluable resource for understanding Angular concepts, with guides, tutorials, and API references.
- Angular – The Complete Guide by Maximilian Schwarzmüller: This paid course provides a comprehensive introduction to Angular, covering all essential concepts and building a real-world project.
- Angular University: Angular University offers a wide range of courses and tutorials on Angular, covering various topics and skill levels.
- Angular Essentials (Angular 2+ with TypeScript) by Codevolution: This YouTube playlist contains a series of free video tutorials that cover the essentials of Angular and TypeScript.
Discovering Vue.js: A Beginner’s Guide
Vue.js is a versatile and approachable JavaScript framework for building user interfaces. Created by Evan You, it has gained popularity due to its simplicity, flexibility, and excellent performance. In this section, we’ll introduce you to essential Vue.js concepts and guide you through the process of building your first Vue.js app.
- Vue Instances: Learn about Vue instances, the core building blocks of a Vue.js application, and how to create and configure them using various options.
- Templates and Data Binding: Understand how Vue templates work and how to use data binding techniques, such as interpolation, v-bind, and v-model, to create dynamic and interactive user interfaces.
- Directives: Discover Vue directives, which are used to manipulate the DOM and add custom behaviors to elements, such as v-if, v-for, v-show, and v-on.
- Components: Learn about Vue components, reusable and self-contained pieces of functionality that can be easily integrated into your application.
- Computed Properties and Watchers: Explore the concepts of computed properties and watchers in Vue, which allow you to create reactive and performant data transformations.
- Lifecycle Hooks: Familiarize yourself with Vue lifecycle hooks, which enable you to perform actions at different stages of a component’s life, such as beforeCreate, created, beforeMount, and mounted.
- Routing: Understand how to implement client-side routing in your Vue.js app using Vue Router, allowing you to create seamless navigation experiences.
- State Management: Learn about Vuex, a state management library for Vue.js applications, to handle complex application state and improve performance.
Building Your First Vue.js App
- Set up your development environment by installing Node.js and npm (Node Package Manager).
- Install the Vue CLI using
npm install -g @vue/cli
. - Create a new Vue.js project by running
vue create my-first-vue-app
(replace “my-first-vue-app” with your desired project name). - Navigate to the project directory using
cd my-first-vue-app
and start the development server usingnpm run serve
. - Open your favorite code editor and explore the project structure. Modify the
src/components/HelloWorld.vue
file to create your first component. - Add interactivity to your component by implementing data binding, directives, and event handling.
- Experiment with additional components, computed properties, watchers, routing, and state management to build a more complex app.
Recommended Resources for Learning Vue.js:
- Upmostly Vue Category
- Official Vue.js Documentation: The official documentation is an excellent resource for understanding Vue.js concepts, with guides, tutorials, and API references.
- Vue.js: Getting Started by Maximilian Schwarzmüller: This paid course provides a comprehensive introduction to Vue.js, covering all essential concepts and building a real-world project.
- VueSchool: VueSchool offers a wide range of courses and tutorials on Vue.js, covering various topics and skill levels.
- The Vue.js Master Class by Vue Mastery: This online course covers Vue.js in depth, teaching you how to build a real-world application from scratch.
Venturing into Other JavaScript Frameworks and Libraries
While React, Angular, and Vue.js are some of the most popular JavaScript frameworks, the web development ecosystem is vast and ever-evolving. As you continue to grow as a developer, it’s essential to keep exploring new frameworks, libraries, and tools. In this section, we’ll introduce you to a few more notable JavaScript frameworks and libraries to consider diving into next.
- Svelte: Svelte is a unique, compile-time framework that differs from traditional frameworks like React or Vue. It compiles your code into efficient, vanilla JavaScript, resulting in smaller bundles and better performance. Learn the basics of Svelte and explore its unique approach to web development.
- Next.js: Next.js is a powerful framework built on top of React, focusing on server-rendered React applications. It offers features like automatic code-splitting, static exporting, and built-in CSS and TypeScript support. Understand the benefits of server-rendered applications and learn how to build them using Next.js.
- Nuxt.js: Nuxt.js is a Vue.js-based framework for building server-rendered applications and static websites. It provides a high-level, opinionated structure and simplifies the development process. Explore the world of server-rendered Vue applications by learning Nuxt.js.
- Gatsby: Gatsby is a modern, static-site generator that uses React, GraphQL, and Webpack to build blazing-fast websites. Discover the advantages of static websites, learn how to work with Gatsby’s plugin ecosystem, and create high-performance websites.
- Ember.js: Ember.js is an opinionated, full-featured JavaScript framework for building ambitious web applications. It promotes conventions over configurations and provides a structured approach to development. Learn the fundamentals of Ember.js and explore its conventions, components, and routing system.
Expanding Your Skill Set
As you venture into new frameworks and libraries, consider the following tips to enhance your learning experience:
- Follow Tutorials and Courses: Look for high-quality tutorials, courses, and articles to learn the basics of new frameworks and libraries. Official documentation and community-created resources can be great starting points.
- Build Projects: Apply what you’ve learned by building small projects, experimenting with different features and techniques, and gradually increasing the complexity of your projects.
- Join Communities: Participate in online forums, social media groups, and local meetups related to the frameworks and libraries you’re learning. Engage in discussions, ask questions, and share your knowledge with others.
- Stay Current: Follow industry news, blogs, and podcasts to stay informed about new developments, best practices, and emerging trends in the world of web development.
- Contribute to Open Source: As you gain experience, consider contributing to open-source projects within the frameworks and libraries you’re learning. This will not only help you improve your skills but also give back to the community.
Closing Thoughts
Mastering JavaScript frameworks and libraries is a valuable skill in today’s web development landscape. By exploring popular frameworks like React, Angular, and Vue.js, along with other notable options such as Svelte, Next.js, and Gatsby, you’ll be well-equipped to tackle a wide range of projects and challenges. Remember to stay curious, keep learning, and enjoy the journey. As you continue to grow as a developer, don’t forget to give back to the community by sharing your knowledge and contributing to open-source projects.
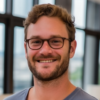
💬 Leave a comment