In the ever-evolving world of front-end development, Vue.js has emerged as a popular and versatile JavaScript framework. Its ease of use, powerful features, and excellent performance make it a top choice for developers looking to build scalable and maintainable web applications. One of the core features that sets Vue.js apart is its directives, which offer a simple yet powerful way to manipulate the DOM and add dynamic behavior to your application.
In this comprehensive guide, we’ll dive deep into the world of Vue.js directives, exploring both built-in and custom options. You’ll learn how to harness the power of these handy tools to write cleaner, more efficient code, and we’ll also share tips, best practices, and real-world examples along the way. So, let’s get started!
What are Vue.js Directives?
Vue.js directives are special HTML attributes used to manipulate the DOM (Document Object Model) and modify its behavior based on your application’s data. Directives in Vue.js are prefixed with v-
, making it easy to identify them within your HTML templates. They provide a declarative way to bind your data and logic to the DOM, making your code more readable and maintainable.
Directives are one of the core features of Vue.js, and they play a significant role in simplifying the process of creating dynamic and interactive web applications. By using directives, you can perform various operations, such as conditionally rendering elements, binding attributes, handling events, and looping through data, without the need for verbose JavaScript code.
In Vue.js, directives can have arguments, modifiers, and expressions. Arguments are denoted by a colon (:
) after the directive name, while modifiers are denoted by a dot (.
). Expressions are JavaScript code snippets enclosed in double curly braces ({{ }}
) that determine how the directive should behave.
Here’s a simple example of using a directive in a Vue.js template:
<div id="app">
<p v-if="isVisible">This paragraph is conditionally rendered.</p>
</div>
In this example, the v-if
directive is used to conditionally render the paragraph element based on the value of the isVisible
property in the Vue instance. If isVisible
is true, the paragraph will be displayed; otherwise, it will be removed from the DOM.
Built-in Directives Overview
Vue.js comes with a rich set of built-in directives that enable developers to easily add dynamic behavior to their applications. These directives are simple yet powerful, allowing you to bind data, control the flow of your application, and manipulate the DOM without writing complex JavaScript code. In this section, we’ll provide an overview of some of the most commonly used built-in Vue.js directives and their functionalities.
v-bind
v-bind
is used to bind an attribute or a property on a DOM element to an expression or a data property in your Vue instance. This is particularly useful when you want to dynamically update the value of an attribute based on your application’s state.
<img v-bind:src="imageSource" alt="A dynamic image">
In this example, the src
attribute of the img
element is bound to the imageSource
data property in the Vue instance. When the value of imageSource
changes, the image source will be updated accordingly.
v-model
v-model
is a two-way binding directive that creates a connection between a form input element and a data property in your Vue instance. This enables you to automatically sync the value of the form input with the corresponding data property.
<input type="text" v-model="username" placeholder="Enter your username">
In this example, the username
data property is bound to the input element. Any changes made to the input value will automatically update the username
property, and vice versa.
v-if, v-else, and v-else-if
v-if
, v-else
, and v-else-if
are conditional rendering directives that control the visibility of elements based on the evaluation of an expression.
<p v-if="isLoggedIn">Welcome, {{ username }}!</p>
<p v-else>Login to view your dashboard.</p>
In this example, the first paragraph element will be displayed only if the isLoggedIn
data property is true
. Otherwise, the second paragraph element will be displayed.
v-for
v-for
is a loop directive that enables you to iterate over an array or an object and render elements based on the items in the collection.
<ul>
<li v-for="item in items" :key="item.id">
{{ item.name }}
</li>
</ul>
In this example, the li
element is repeated for each item in the items
array. The :key
attribute is used to provide a unique identifier for each item, which is necessary for Vue’s internal optimizations.
v-on
v-on
is an event handling directive that enables you to listen for DOM events, such as clicks or keyboard input, and trigger methods in your Vue instance.
<button v-on:click="submitForm">Submit</button>
In this example, the submitForm
method in the Vue instance will be called when the button is clicked.
These are just a few of the many built-in directives provided by Vue.js. By understanding and utilizing these directives, you can create more dynamic and interactive applications with minimal code. In the following sections, we’ll dive deeper into each directive and explore their practical use cases.
Creating Custom Directives
While Vue.js provides a set of powerful built-in directives, you might encounter situations where you need a custom directive to perform a specific task or handle a unique scenario. Vue.js allows you to create custom directives, giving you the flexibility to extend the functionality of the framework according to your needs. In this section, we’ll discuss the process of creating custom directives and explore some practical examples.
Defining a Custom Directive
To create a custom directive, you need to define it using the Vue.directive()
method, which takes two arguments: the directive’s name and an object containing the directive’s options. The name of the custom directive should be in kebab-case, and the options object can have several properties, such as bind
, update
, and unbind
, which are lifecycle hooks of the directive.
Here’s a simple example of creating a custom directive called v-focus
:
Vue.directive('focus', {
inserted: function(el) {
el.focus();
}
});
In this example, we define a custom directive named focus
that sets the focus on an input element when it’s inserted into the DOM. The inserted
lifecycle hook is called when the element is inserted into the DOM, and we use it to call the focus()
method on the element.
Using a Custom Directive
To use a custom directive in your Vue.js application, simply add the directive to the appropriate DOM element using the v-
prefix followed by the directive’s name, like this:
<input type="text" v-focus>
When this input element is inserted into the DOM, our custom v-focus
directive will automatically set the focus on the element.
Practical Example: Auto-growing Textarea
Let’s create a custom directive called v-auto-grow
that automatically adjusts the height of a textarea element based on its content. This can be useful in applications where users may input variable amounts of text.
Vue.directive('auto-grow', {
bind: function(el) {
el.addEventListener('input', autoGrow);
},
unbind: function(el) {
el.removeEventListener('input', autoGrow);
}
});
function autoGrow(event) {
const textarea = event.target;
textarea.style.height = 'auto';
textarea.style.height = textarea.scrollHeight + 'px';
}
In this example, we define a custom directive named auto-grow
with bind
and unbind
lifecycle hooks. In the bind
hook, we add an event listener to the textarea element for the input
event, which calls the autoGrow
function. In the unbind
hook, we remove the event listener when the directive is unbound from the element.
The autoGrow
function adjusts the textarea’s height based on its scroll height, allowing the textarea to grow or shrink automatically as the user types.
To use our custom v-auto-grow
directive, simply add it to a textarea element:
<textarea v-auto-grow placeholder="Type your message here"></textarea>
With this custom directive, the textarea element will now automatically adjust its height based on its content, providing a more user-friendly experience.
Tips and Best Practices for Using Directives
When working with directives in Vue.js, it’s essential to follow best practices and adhere to some guidelines to ensure that your code is efficient, maintainable, and easy to understand.
1. Use Shorthand Syntax
Vue.js provides shorthand syntax for commonly used directives like v-bind
and v-on
. Using the shorthand syntax can make your code more concise and easier to read.
For v-bind
, you can use the :
prefix instead of writing out v-bind
:
<!-- Long syntax -->
<img v-bind:src="imageSource" alt="A dynamic image">
<!-- Shorthand syntax -->
<img :src="imageSource" alt="A dynamic image">
For v-on
, you can use the @
prefix instead of writing out v-on
:
<!-- Long syntax -->
<button v-on:click="submitForm">Submit</button>
<!-- Shorthand syntax -->
<button @click="submitForm">Submit</button>
2. Use Computed Properties for Complex Expressions
Directives can accept complex expressions, but it’s generally recommended to use computed properties for any expression that requires more than a simple data property lookup or operation. Computed properties make your code more readable and maintainable by abstracting complex logic away from your template.
computed: {
fullName: function() {
return this.firstName + ' ' + this.lastName;
}
}
<p>Welcome, {{ fullName }}!</p>
3. Be Mindful of Directive Order
The order in which directives are applied to an element can sometimes matter, especially when you have multiple directives that depend on each other. For instance, if you use both v-for
and v-if
on the same element, be aware that v-for
has a higher priority than v-if
. This means the v-if
condition will be checked for each item in the loop, rather than the loop being skipped entirely if the v-if
condition is false.
To avoid potential issues, it’s recommended to use a computed property to filter the items before looping through them with v-for
.
4. Avoid Overusing Custom Directives
While custom directives can be powerful, they should be used sparingly and only when necessary. In many cases, using Vue.js components may be a better choice, as they provide a more flexible and reusable solution. Use custom directives when you need low-level DOM manipulation or access to lifecycle hooks that components don’t offer.
5. Keep Directives Focused and Single-Purpose
Directives should be focused on a single purpose and have a clear, well-defined responsibility. Avoid creating multi-purpose directives that handle multiple concerns, as this can make your code harder to understand and maintain. By keeping your directives focused, you’ll ensure that they are reusable, modular, and easy to reason about.
By following these tips and best practices, you’ll be able to write cleaner, more efficient, and maintainable Vue.js applications that make the most of the powerful features that directives have to offer.
Common Use Cases and Examples
Directives in Vue.js can be used in various scenarios to make your applications more dynamic, interactive, and user-friendly.
Example 1: Dynamic CSS Classes
One common use case for directives is to apply dynamic CSS classes to elements based on the application’s state. The v-bind
directive can be used to bind the class
attribute of an element to a computed property or an object that determines the classes to apply.
<!-- Using a computed property -->
<div :class="boxClass">...</div>
codecomputed: {
boxClass: function() {
return this.isActive ? 'box-active' : 'box-inactive';
}
}
In this example, we use the v-bind
directive to bind the class
attribute of a div
element to the boxClass
computed property, which returns either 'box-active'
or 'box-inactive'
based on the value of the isActive
data property.
Example 2: Dynamic Form Validation
Directives can be used to implement dynamic form validation by conditionally applying styles or displaying error messages based on user input. The v-model
directive is particularly useful in this context, as it provides two-way binding between form elements and data properties.
<form @submit.prevent="submitForm">
<div :class="{ 'error': usernameError }">
<input type="text" v-model="username" @input="validateUsername" placeholder="Enter your username">
<p v-if="usernameError" class="error-message">Username is required.</p>
</div>
<!-- More form fields and a submit button... -->
</form>
codedata: {
username: '',
usernameError: false
},
methods: {
validateUsername: function() {
this.usernameError = !this.username.trim();
},
submitForm: function() {
this.validateUsername();
if (!this.usernameError) {
// Submit the form...
}
}
}
In this example, we use the v-model
directive to bind the username
data property to the input element, and the v-if
directive to conditionally display an error message when the usernameError
property is true. We also use the v-bind
directive to apply the 'error'
CSS class to the input’s container element when there’s a validation error.
Example 3: Conditional Rendering of Components
Sometimes, you may want to conditionally render components based on the user’s role or the application’s state. Directives like v-if
, v-else
, and v-else-if
can be used to achieve this functionality.
<admin-dashboard v-if="userRole === 'admin'"></admin-dashboard>
<user-dashboard v-else></user-dashboard>
In this example, we use the v-if
and v-else
directives to conditionally render either the admin-dashboard
or user-dashboard
components based on the value of the userRole
data property.
These examples demonstrate the versatility and power of directives in Vue.js applications. By understanding and applying directives in various scenarios, you can create more dynamic, interactive, and user-friendly web applications that cater to your users’ needs.
Closing Thoughts
Directives are an essential aspect of Vue.js, providing developers with a powerful toolset to create dynamic, interactive, and user-friendly web applications. By mastering built-in directives, creating custom ones when needed, and adhering to best practices, you can harness the full potential of Vue.js and build exceptional web experiences for your users. Remember to keep directives focused, make use of computed properties, and leverage the versatility of directives to solve a wide range of problems.
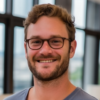
💬 Leave a comment