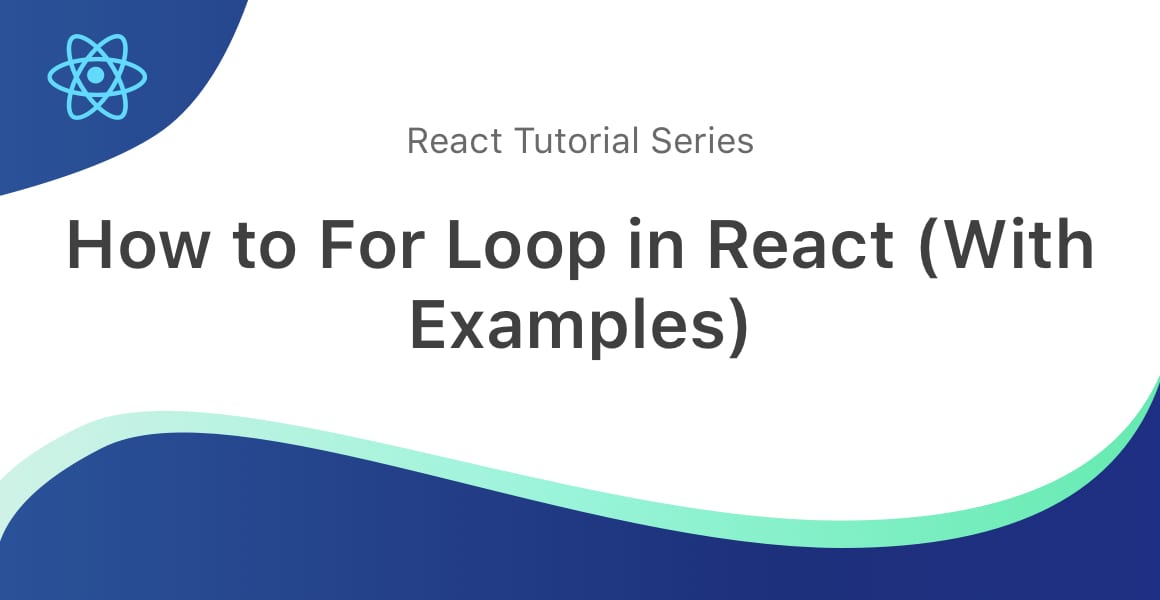
Let’s explore how to write a for loop in React. Although we can write a for loop in React, ES6 provides the more appropriate map function for us to use.
To For Loop or Map in React
As with most things in web development, there are multiple ways to loop, or iterate, through an array in React using JavaScript.
Some of the iterators we have at our disposal in JavaScript are:
- Map (ES6)
- ForEach
- For-of
Out of the three iterators above, our best option to iterate over an array in React inside of JSX is the Map function.
Let’s begin by exploring how we can use the Map iterator to loop through elements in an array and render out some HTML for each of those elements.
Using The Map Function in React
Introduced in ES6, the Map array function is by far my most used method of iterating over an array in React.
Why is it my most commonly used method of looping through arrays in React? Because it’s incredibly versatile, succinct, and easy to read once you wrap your head around it.
Take the example code below:
const names = ['James', 'Paul', 'John', 'George', 'Ringo'];
function App() {
return (
<div>
{names.map(name => (
<li>
{name}
</li>
))}
</div>
);
}
We begin by initializing an array called names and populate it with five strings. You might recognize some of the names in this array.
const names = ['James', 'Paul', 'John', 'George', 'Ringo'];
Then, we define a functional React component named App.
Remember, since the release React 16.8, Class components are no longer necessary. We can add state to functional components, therefore I like to always use functional React components in my tutorials.
function App() {
return (
<div>
...
</div>
);
}
Our functional React component returns a single div element with some JSX inside. JSX is absolutely brilliant. It’s like magic. Why? Because It allows us to write JavaScript inside HTML.
Next, we simply loop through the names array using the brilliantly simple Map Array function. Again, because we’re using JSX, we can have our JavaScript Map function output HTML.
In our case, we loop through the names array and output a set of <li> tags for each element in the array, thus creating a list:
{names.map(name => (
<li>
{name}
</li>
))}
Let’s see all of this code put together:
const names = ['James', 'Paul', 'John', 'George', 'Ringo'];
function App() {
return (
<div>
{names.map(name => (
<li>
{name}
</li>
))}
</div>
);
}
Use a Unique Key for Each Rendered Element in a Map Function
To ensure that each HTML element in the React DOM has a unique identifier, you’re required to provide each rendered element with, well, a unique key.
You do this by providing a key attribute on the HTML element that you are rendering inside of the Map function.
In our example above, we rendered a set of li tags in our Map function. Therefore, we have to add an additional attribute to the li tag, like so:
<div>
{names.map((name, index) => (
<li key={index}>
{name}
</li>
))}
</div>
We use the index of the array element for the unique key. Now, this isn’t the best way of providing a unique key because the index can change if you add or remove elements from the array during runtime.
What I’ve done in the past is to use a third party UUID library to generate unique Ids, and use those as a way of uniquely identifying the elements in the DOM.
Thank you for following along with us and if you are starting out with React don’t forget to check out this article to learn how to create your first React app
💬 Leave a comment