Sometimes when writing React components you don’t know ahead what are going to be its children ahead of time. In this case, you would really benefit from knowing how to use the children prop. Let’s take a quick look at how we can apply this pattern in React applications.
Application
In Users.js I have written a simple component that simply renders an h1 element.
const Users = () => {
return (
<div>
<h1>This is a parent component</h1>
</div>
)
}
export default Users;
In App.js I’m importing this element at instantly calling it in the return statement.
import Users from './Users'
const App = () => {
return (
<div style={{ margin: '50px' }}>
<Users/>
</div>
)
}
export default App;
This application simply renders the hardcoded statement to the page like in the below screenshot.

We can use the containment to contain another component within it and render it with more flexibility.
import Users from './Users'
const App = () => {
return (
<div style={{ margin: '50px' }}>
<Users>
<h3>This is a children component</h3>
</Users>
</div>
)
}
export default App;
The only change that I have implemented is that I have nested and h3 element within the Users component. To do that I had to rewrite the code slightly. I removed the self-closing tag and instead added a corresponding closing tag.
Now we can access this nested element from the Users component by using the children property. Let’s render this h3 element to the page.
const Users = ({ children }) => {
return (
<div>
<h1>This is a parent component</h1>
{children}
</div>
)
}
export default Users
As you can see we have destructured the props inline and used the children prop to render it to the page. We used the curly braces to tell the JSX that anything passed between them should be interpreted as JS code.
If you head back to the web page you can see that the h3 element indeed renders to the page.
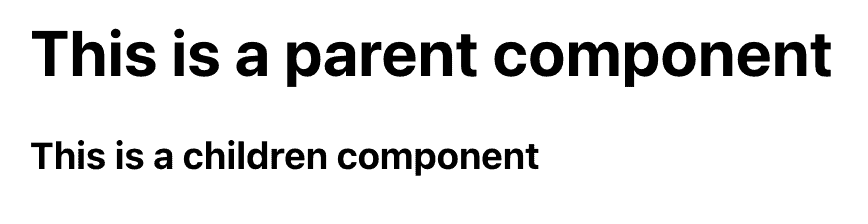
You can also inspect the elements tab in dev tools to make sure that no extra element were added when rendering.
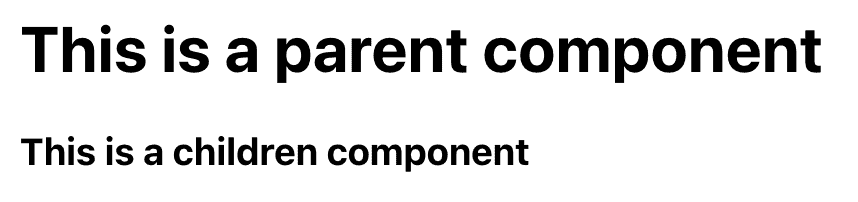
Multiple children
You may be wondering if there is a way to render multiple children like elements to the page within the same component and the answer is yes. It’s actually quite simple.
In this case, you will need to find custom names for those and use them just like you would use regular props. I will use the simple example of name and surname.
import Users from './User'
const App = () => {
return (
<div style={{ margin: '50px' }}>
<Users
name={<h3>Mike</h3>}
surname={<h3>Tyson</h3>}
/>
</div>
)
}
export default App;
const User = ({ name, surname }) => {
return (
<div>
<h1>Current User</h1>
{name}
{surname}
</div>
)
}
export default User
And below you can see the page output and the DOM structure.
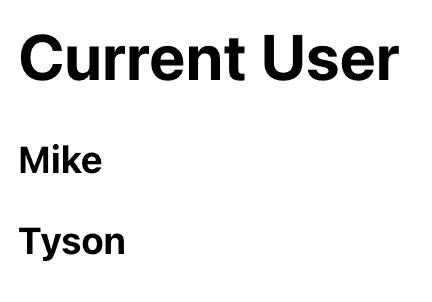
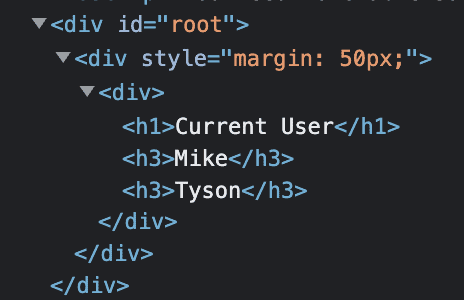
Containment is a great technique to use in your React components. It’s a common practice in well-architected projects and can improve the quality of your code.
💬 Leave a comment