ESlint is an open-source library that’s commonly used by React developers to enforce rules about maintaining the code standard across the project. It’s fully customizable so you can configure the desired rules yourself.
Linintng your code can help you spot any would be bugs. Given that JavaScript is dynamically typed, it’s really easy for developers to introduce hard to debug runtime errors. ESLint can help spot those dangerous patterns and show warnings about them ahead of execution.
Installation
To install ESLint in your project run the
$ npm install eslint --save-dev
in the root directory of our React project. This will install ESLint as a dev dependency.
The next step is to create the config file. ESLint will read from this file and enforce any rules defined in it. Run the below npm command to generate your .eslintrc.js file
npm init @eslint/config
This command will ask you a couple of questions about your project. Take a look at the screenshot below to see my preferences.
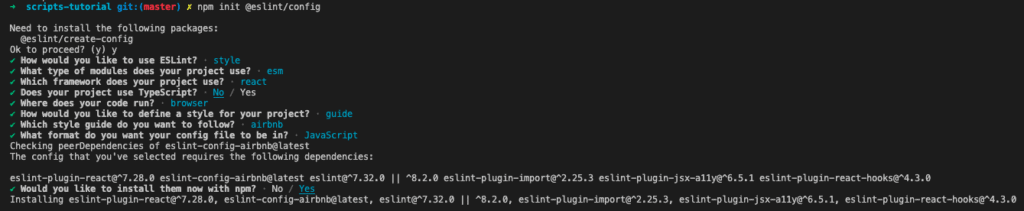
Usage
Let’s now take a look at the generated config file.
module.exports = {
env: {
browser: true,
es2021: true,
},
extends: [
'plugin:react/recommended',
'airbnb',
],
parserOptions: {
ecmaFeatures: {
jsx: true,
},
ecmaVersion: 'latest',
sourceType: 'module',
},
plugins: [
'react',
],
rules: {
},
};
As you can see above we’re extending the Airbnb coding style guide. We can further customise this guide by overwriting the predefined rules with our custom rules.
Let’s now take a look that our App.js where we have a small piece of state, which we then render to the page.
import { useState } from 'react';
import './App.css';
function App() {
const [user, setUser] = useState({name: 'Tommy', surname: 'Smith', age: 12})
return (
<div style={{ margin: '50px' }}>
<h1>Current User:</h1>
<h2>Name: {user.name}</h2>
<h2>Surname: {user.surname}</h2>
<h2>Age: {user.age}</h2>
</div>
);
}
export default App;
The code seems to be fine at the first glance, but when we look at the terminal we can see that the ESLint is not happy about a couple of things and asks us to action those.
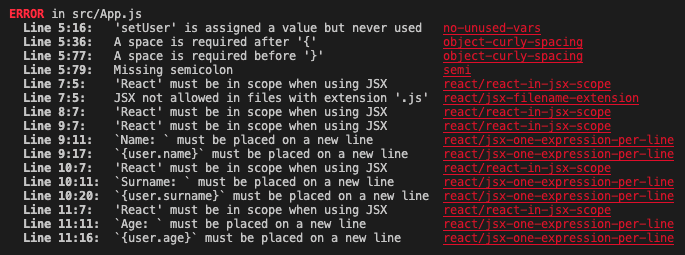
We can also see those in the text editor itself.
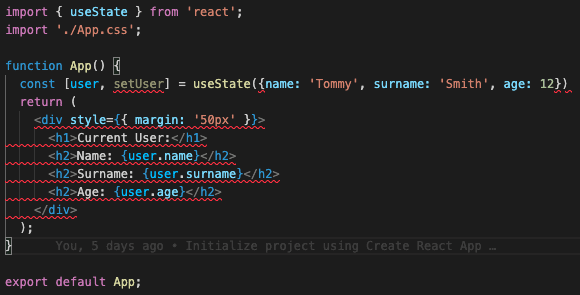
I actioned some of the errors as I think they are generally good coding practices. I temporarily disabled the no-unused-vars error as I want it to be enforced across the whole codebase, but in this particular case, the component is still under development so the rule is temporarily disabled.
import React, { useState } from 'react';
import './App.css';
function App() {
// eslint-disable-next-line no-unused-vars
const [user, setUser] = useState({ name: 'Tommy', surname: 'Smith', age: 12 });
return (
<div style={{ margin: '50px' }}>
<h1>Current User:</h1>
<h2>Name: {user.name}</h2>
<h2>Surname: {user.surname}</h2>
<h2>Age: {user.age}</h2>
</div>
);
}
export default App;
I also don’t want neither react/jsx-one-expression-per-line nor react/jsx-filename-extension enforced at all. I went ahead and disabled them in the codebase altogether.
rules: {
'react/jsx-one-expression-per-line': 'off',
'react/jsx-filename-extension': 'off',
},
All of the console ESLint errors had been actioned at this point. We can now head over to the browser and see that the application compiles successfully.
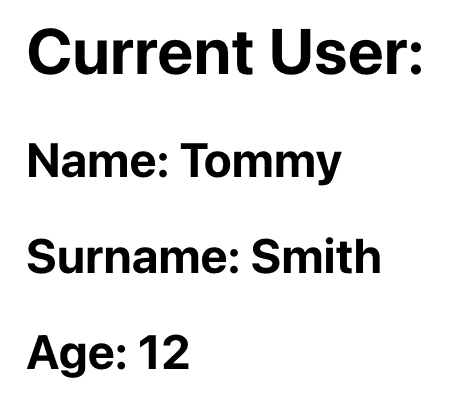
ESLint is a huge library that has hundreds of different rules available. Make sure that you check the official docs to learn more about it.
💬 Leave a comment