If you’re building web apps in Next.js, you’ve probably had to iterate through an array at some point. This means you’ll have run into the issue of keys; unique ids for each element. In this article, we’ll talk about keys, when to use them, and how to create them.
What are keys?
The key prop is a commonly used attribute in Next.js and React applications. It is used to help identify each unique component within a list of components, and it plays an essential role in optimizing performance.
When rendering a list of components, React needs to be able to identify each item in the list so that it can efficiently update the UI when changes occur. The key
prop provides React with a way to identify each item and keep track of its state.
It should be a string or a number, and it should be unique among all the siblings in the list. This means that no two items in the list should have the same key.
When a list is updated, React will compare the new list with the old list to determine which items have been added, removed, or updated. By using the key prop, React can quickly identify which items have changed and update only those items, rather than re-rendering the entire list.
What not to do
You might see a straightforward solution to this. We have an array, so we have index keys, so use their indexes. There are some scenarios where this might be fine, but there are also a lot of situations where this will cause problems. The keys will be unique, there won’t be any repeats, but if you change your array, they might change which item they refer to.
Let’s think about it with some code:
const children = {
0: <div>Hello</div>,
1: <div>World</div>
}
We have our children, with our keys on the left, but now I want to change the message to say Hello Upmostly. So, let’s modify our array:
const children = {
0: <div>Hello</div>,
1: <div>Upmostly</div>
}
So React sees the first key is the same, so we don’t need to re-render, and the second key is the same, so we don’t need to re-render. But that’s wrong, right? Since we used the same key for two different items, React can’t tell that our item has changed.
To sum it up, the main two reasons are:
- Performance: When you use array indexes as keys, you can run into performance issues if you add or remove items from the beginning of the array. React will re-render all the items in the list, even if they haven’t changed, because it thinks the keys have changed.
- Stability: Array indexes are not stable. If you sort, filter, or otherwise modify the array, the indexes will change. This can lead to confusing bugs if your components rely on the keys for state or props.
So what should we do instead?
Unique Ids
The easiest solution is to look at the data in your array and use any unique properties as keys. This could be an ID that comes from a database, a hash of the item’s properties, or any other value that is guaranteed to be unique.
type User = {
userId: string;
name: string;
}
In that case the solution is straightforward:
export function Keys() {
const [users, setUsers] = useState<User[]>();
return (
<ul>
{users?.map((u) => (
<li key={u.userId}>{u.name}</li>
))}
</ul>
);
}
In some cases you might not have any unique ids. The best solution here then is to create one, e.g. with a uuid, which you can install with:
npm install --save uuid
Let’s imagine in our previous example we didn’t have the userId, just the names:
const users = ['Adam', 'Brian', 'Ruby'];
We can transform this to get some unique ids using uuid:
const uniqueUsers: User[] = users.map((name) => ({ userId: uuidv4(), name }));
Conclusion
So to sum it up, you probably shouldn’t use array indexes as keys, unless you know your data will never change, even if it’s the same items in a different order. To provide keys for your elements, use any unique id properties it might have, and if it doesn’t have one, then you can generate one with something like a UUID. If you liked this article, or if you’re having any difficulties, feel free to leave a comment below!
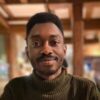
💬 Leave a comment