JSX expressions can be either self-closing or be closed by a corresponding ‘closing’ tag. Anything that goes between those opening and closing tags is referred to as children. Let’s take a look at how JSX children work and how you can improve your code quality by following a couple of patterns.
Strings
The first and most obvious use case for passing children is to render the strings to the page. This is the basic use case for most of the built-in HTML elements.
function App() {
return (
<div style={{ margin: '50px' }}>
Hello World
</div>
);
}
export default App;
It renders the below to the screen.
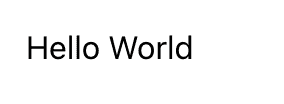
JSX as children
You can provide other JSX elements as children. This will start generating the tree-like component structure that you are surely familiar with.
function App() {
return (
<div style={{ margin: '50px' }}>
<div>Hello World</div>
<h1>Hello from Upmostly!</h1>
</div>
);
}
export default App;
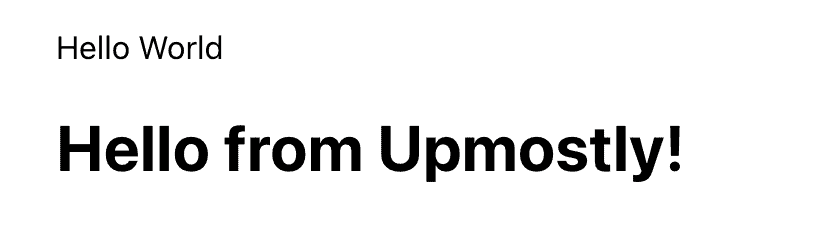
JS Expressions
You can also put any JS expressions you want as children. This is a really useful feature as it can help with making your return statements more dynamic. Let’s take a look at the below example.
function App() {
return (
<div style={{ margin: '50px' }}>
<ul>
{[1,2,3,4,5].map(num => <li key={num}>{num}</li>)}
</ul>
</div>
);
}
export default App;
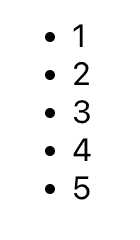
In the return statement of our component, we’re iteration over the array of numbers 1 through 5. We’re mapping those into li elements and nest them as children of the ul element.
We can also use template literals in the same way!
function App() {
const webisiteName = 'Upmostly'
return (
<div style={{ margin: '50px' }}>
<h1>{`Welcome to ${webisiteName}!`}</h1>
</div>
);
}
export default App;
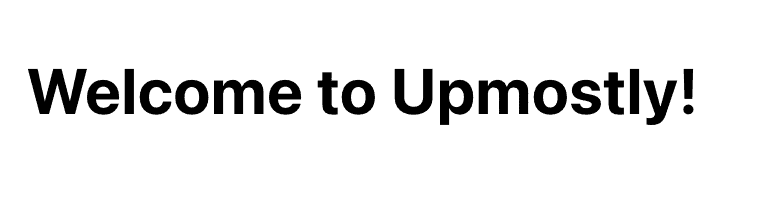
Ternaries
By far my favourite way use case of JS expressions in JSX. It gives you a lot of freedom when it comes to conditional rendering. If the expression evaluates to true it’s entered into the page. If it evaluates to false, null or undefined then it will be simply ignored.
function App() {
return (
<div style={{ margin: '50px' }}>
{true && <h1>This expression renders</h1>}
{false && <h1>This expression doesn't render</h1>}
{15 < 18 && <h1>15 is less than 18</h1>}
{15 > 18 && <h1>15 is NOT less than 18</h1>}
</div>
);
}
export default App;
Which renders the below output
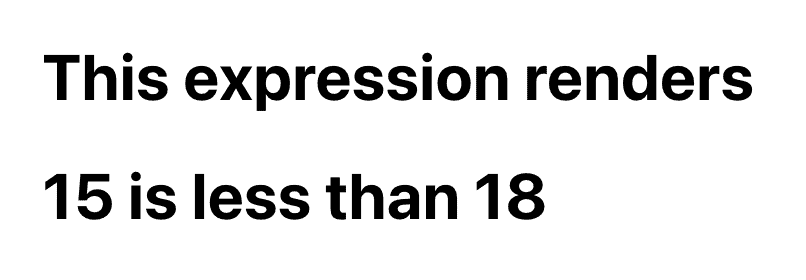
💬 Leave a comment