Introduction
React components are capable of receiving some parameters, these certain parameters are called props in react terminologies, it is not a rule but a convention used by almost all of the react devs.
What is Prop Drilling?
Most of us have encountered the situation where we need to provide the same data on all levels of a component hierarchy, only for that data to be used on the final child component. Let us take the following example
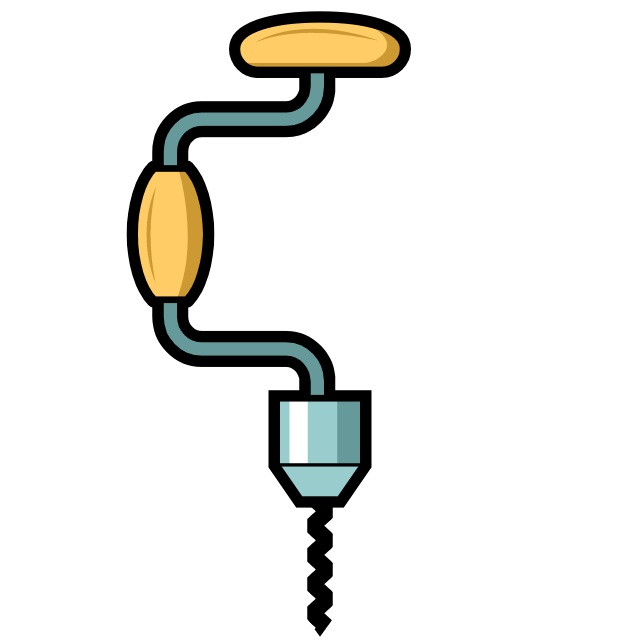
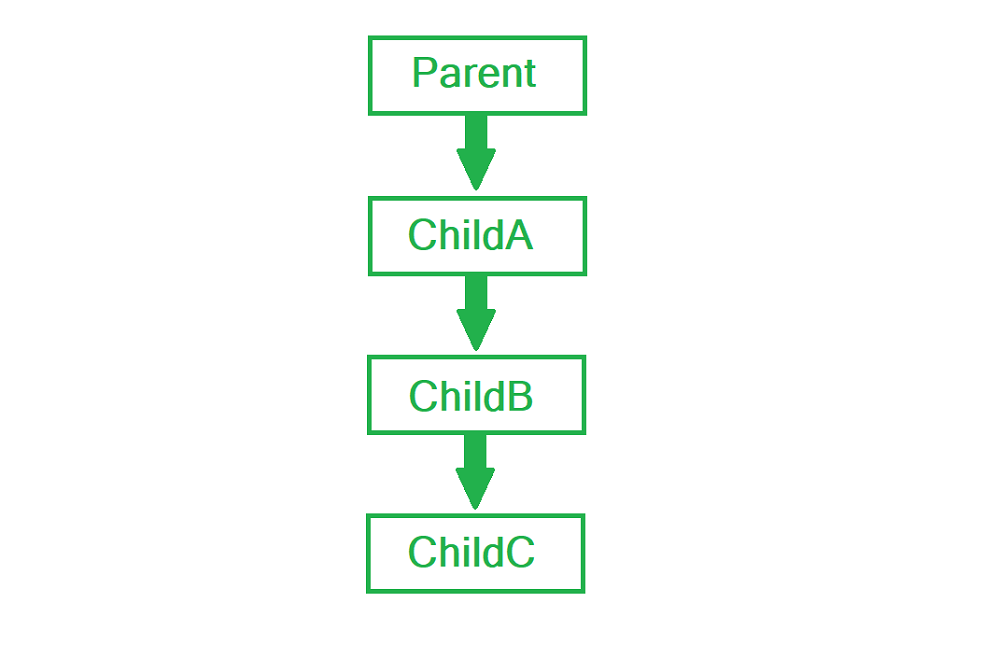
import React, { useState } from "react";
function Parent() {
const [fName, setfName] = useState("firstName");
const [lName, setlName] = useState("LastName");
return (
<>
<div>This is a Parent component</div>
<br />
<ChildA fName={fName} lName={lName} />
</>
);
}
function ChildA({ fName, lName }) {
return (
<>
This is ChildA Component.
<br />
<ChildB fName={fName} lName={lName} />
</>
);
}
function ChildB({ fName, lName }) {
return (
<>
This is ChildB Component.
<br />
<ChildC fName={fName} lName={lName} />
</>
);
}
function ChildC({ fName, lName }) {
return (
<>
This is ChildC component.
<br />
<h3> Data from Parent component is as follows:</h3>
<h4>{fName}</h4>
<h4>{lName}</h4>
</>
);
}
export default Parent;
The Downside of Prop Drilling
Here we are drilling first name and last name from the parent component to the child c component when the component in between them doesn’t need those props. Which ruins data encapsulation as well. With unnecessary data being moved right, left, and centre.
How To Avoid Prop Drilling
- Lift State Up
- Context API
- Some other global state management solutions
- Container pattern
- Compound components
Wrap Up
This one was purely conceptual and I missed the ‘MOMENT OF TRUTH’ too 🫤. But sometimes it is better to learn something so that your code can be more clean, lean, readable, scalable, and self-explanatory. I hope you learned what you were doing wrong in your react application and how you can get around it. I will see you at the next one. This is not a goodbye 🤓. Take Care.
💬 Leave a comment