There are a few scenarios you might want to be splitting strings in TypeScript, whether it’s splitting a paragraph by newline characters, splitting a list by commas, etc
The .split() Function
Let’s explore the .split() function using a file just containing some randomly generated emails.
We can really easily load these emails into TypeScript using the fs module.
const emailFile = fs.readFileSync('./emails.csv', { encoding: 'utf8' });
And just for a sample of what our randomly generated email file looks like:
uglyKeri10@free.fr,gorgeousKayla@wanadoo.fr,pricklyRussell@yahoo.ca,livelyBrian@aim.com,condemnedHannah@aliceadsl.fr... etc
With the .split() function, splitting these by the comma is very simple:
const emails = emailFile.split(',');
console.log(emails);
[
'uglyKeri10@free.fr',
'gorgeousKayla@wanadoo.fr',
'pricklyRussell@yahoo.ca',
'livelyBrian@aim.com',
,...
]
The split function splits the string by the given separator, and we get back our list of email addresses in an array.
Splitting by Newline Characters
If our email list was split by newline characters, splitting the list by newlines is a little more complicated, but still quite straightforward.
The key difference is different operating systems use different characters to mark a new line. If you’re on Windows, your text files will probably use “/r/n”. Linux uses “\n”, and on the off chance you’re on an old Mac, they will use “\r”.
The service I’ve used to randomly generate my emails uses “\r\n”. Since I know this, we could just change our code to:
const emails2 = emailFileNewlines.split('\r\n');
However, if you’re unsure of where your text file is going to come from, it’s very easy to change your code to cover any possibility. The .split() function also accepts a regex statement as a parameter to split by, instead of a string.
If you’re not sure what Regex is, Regex, or Regular Expressions, are a way of defining patterns to search for in text, e.g. “numbers that end in a 5”, or “sentences that mention TypeScript”. Regex is an extremely powerful tool, so if you’re unfamiliar with it, I’d recommend reading up on it. RegExr is a great starting point for playing around with Regex.
Here’s my full code for this approach:
const emailFileNewlines = fs.readFileSync('./emails-newline.txt', {
encoding: 'utf8',
});
const emails2 = emailFileNewlines.split(/\r\n|\r|\n/);
Our Regex might look confusing, but it’s very simple. This just reads as “\r\n” or “\r” or “\n”, covering all of our options.
Printing the result again, we get exactly what we expect!
[
'realStacy@hotmail.com',
'Martinhelpful@voila.fr',
'Davidcreepy@web.de',
'elegantJason66@earthlink.net',
'deadRichard@yahoo.fr',...
]
Regex
With Regex, you can also have a more complex approach to this. Our code above matches the newlines/commas, and gives you the text around them. If you’re looking for something more complex, we can do something more elaborate with Regex.
Here’s an example using Regex to get all the gmail emails from our list:
const emails3 = emailFileNewlines.match(/.*@gmail.com(?=(\r\n|\r|\n))/g);
Breaking this down we get:
- We start with “.*”. The . means “any character”, while the * means “any amount of that character”. This just matches any amount of any character before our next string.
- @gmail.com just means we want it to be followed by @gmail.com
- (?=foo) is the positive lookahead character. This means we want our match to end with whatever is inside the brackets, but not include it in the match. In this case, this means our regex from before, which is just matching any newline character we might run into.
Then if we run this, we get:
[ 'Nataliegorgeous@gmail.com', 'thoughtlessNikki7@gmail.com' ]
The two gmail emails we have in our list!
Conclusion
Thanks for reading this article on splitting strings in TypeScript. Hopefully, I’ve helped you out with these simple examples, and you can expand them for your own use cases. If you’re having any troubles, or if you simply just liked this article, feel free to leave a comment below!
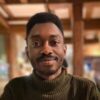
💬 Leave a comment