When working with TypeScript or migrating an already existing codebase over to TypeScript, it is crucial to know how to handle typing properly.
One tricky typing scenario to get right is when we decide whether or not to use any
for a particular scenario.
In this article, we’ll discuss about the any
type; what it is, how it works, and, more importantly, when you should use it or avoid it.
What is the any type?
In some situations, not all type of information is available, or its declaration would take an inappropriate amount of effort. These may occur for values from code written without TypeScript or a 3rd party library. In these cases, we might want to opt out of type-checking.
Unlike unknown
, variables of type any
allow you to access arbitrary properties, even ones that donβt exist. These properties include functions, and TypeScript will not check their existence or type:
let looselyTypedVar: any = 4;
// It's ok as ifItExists might exist at runtime
looselyTyped.ifItExists();
// toFixed exists (but the compiler doesn't check)
looselyTyped.toFixed();
let strictlyTyped: unknown = 4;
strictlyTyped.toFixed();
// Will give us an error: "Object is of type 'unknown'".
The any
type will propagate over through objects:
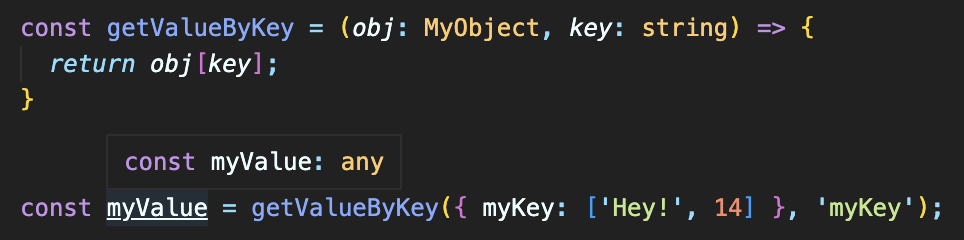
any
type propagationWhen should you use any
?
It does seem like any
is highly convenient when working with TypeScript, which is true; however, the issue is: what do we use TypeScript for then?
You see, it’s easy to try to avoid complex types when there’s the option to use any
, but that should be the exception rather than the role.
So, then, when should we use any
?
- When dealing with highly complex types
- When dealing with any external API data (That cannot be otherwise typed out)
Those are the only two scenarios where I would say it’s acceptable to use any
; otherwise, it only does chip away at the benefits that have come with the adoption of TS in the first place.
Alternatives to any
You might think there are other scenarios where any would be helpful or OK to use, but in my last two years of working with TypeScript, I wouldn’t say there are.
Apart from those two scenarios (The first being a bit more subjective), I’ve found the unknown
type also to do the job just as fine while proving to be safer in the meanwhile.
You can check out more about the unknown
type in this nice article I’ve read.
Other Mentions
It’s also helpful to know that the any
type is the default for types that have not been resolved or typed yet.
That isn’t particularly good, considering how easy it is to forget about correctly typing a variable, function parameter, or the return type for a function.
A solution to this rather common issue is to turn off the implicit any
assignment and only allow for manual any
assignment to any piece of code that can be typed, meaning that any
is fine as long as it’s been manually set so by someone.
There is a rule that’s called noImplicitAny
and which can be found and/or added inside a tsconfig.json
file like this:

any
typeAfter enabling the rule we can see an error message appearing when trying to use an untyped parameter:
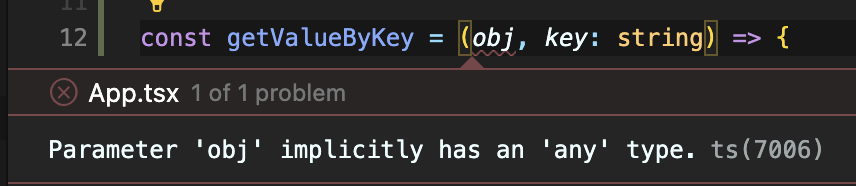
noImplicitAny
rule enabled – Untyped Function Argument ErrorSummary
I hope you’ve enjoyed the read and have gotten a bit more insight into what the any
type is, what it is used for, as well as why and when we should use it (Or better said, not use it), as well as my thoughts and other suggestions on the subject.
Let me know if you have any feedback for this article by leaving a comment in the comment section below.
Cheers!
π¬ Leave a comment