When developing TypeScript applications, you may need to delay the execution of a function for a certain amount of time. Delaying function execution can be useful for a variety of reasons, such as waiting for an animation to complete or for an API request to finish. In this article, I’ll show you how to delay function execution in TypeScript, step-by-step.
We also have an article covering how to implement this in React here.
setTimeout()
To delay a function in TypeScript, you can use setTimeout(), which accepts a callback function and a delay time in milliseconds, after which it will call your function. Here’s the signature for it:
const timeoutId = setTimeout(function callbackFunction() {}, delayMs)
We also get back an id which can be used to cancel the timeout:
clearTimeout(timeoutId);
Then we can use them like this:
console.log('Ill print first');
setTimeout(() => {
console.log('Ill print third after a second');
}, 1000);
console.log('Ill print second');
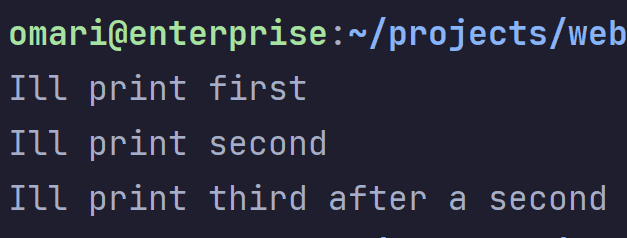
And cancelling a timeout is also very simple. You might use this in something like React, where you might need to cancel the process before it finishes executing:
const firstTimeout = setTimeout(() => {
console.log('I hope no-one cancels this timeout!');
}, 1000);
setTimeout(() => {
console.log('Cancelling the first timeout');
clearTimeout(firstTimeout);
}, 500);

Here the first function never gets ran, since the timeout gets cancelled before it ever gets to execute.
You might notice some possibly unexpected behaviour here; the function gets ran after our delay, but nothing will wait for it. Code after the timeout won’t be delayed. In some situations, this might be fine, but if you are looking for that behaviour, there’s still a way to get around this.
Promise
By wrapping a promise around setTimeout, we can await it, and thus delay all of the code until that timeout finishes. Here’s the promise-ified setTimeout():
function delay(ms: number) {
return new Promise((resolve) => setTimeout(resolve, ms));
}
And here’s how to use it:
console.log('Ill run first');
await delay(1000);
console.log('Ill run second');
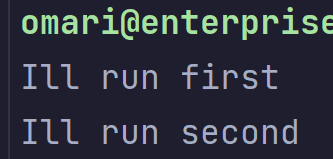
With my implementation, there is no callback function, but we can easily create a version which still calls a callback function when the timer runs out:
function setTimeoutPromise(callback: () => void, ms: number) {
return new Promise((resolve) => setTimeout(resolve, ms)).then(callback);
}
And use it like this:
console.log('Ill run first');
await setTimeoutPromise(() => console.log('Ill run second'), 1000);
console.log('Ill run third');
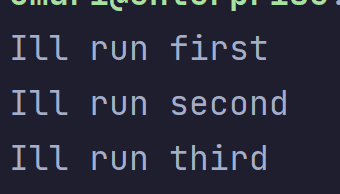
Which option you want to use is up to you, and both act very similarly.
Conclusion
So there you have it! Now you know how to delay function execution in TypeScript like a pro.
By following the simple steps we’ve outlined, you can easily add delays to your functions and create more dynamic and interactive applications. Whether you’re waiting for an API request to complete or simply want to add a pause between animations, this technique will come in handy time and time again. If you liked this article, or if you’re having any troubles, feel free to leave a comment below!
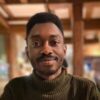
💬 Leave a comment