A common issue when adding a required attribute to a textbox/textarea in an Angular application is that someone can just press space bar once, and the “required” validation is completed. A space counts as character!
The simple way to get around this is to use a regex pattern attribute to validate that there is more than just a space. Something like :
<input type="text" pattern="^(?!\s*$).+" />
But this quickly gets annoying to copy and paste everywhere. A better solution if you need to validate multiple fields the same way is to create a nice directive. Here’s one I prepared earlier :
import { Directive } from '@angular/core';
import { NG_VALIDATORS, Validator, ValidationErrors, AbstractControl } from '@angular/forms';
@Directive({
selector: '[noWhitespace]',
providers: [{ provide: NG_VALIDATORS, useExisting: NoWhitespaceDirective, multi: true }]
})
export class NoWhitespaceDirective implements Validator {
constructor() { }
validate(control: AbstractControl): ValidationErrors {
if(!control.value || control.value.trim() == '')
{
return {'required' : true };
}
return null;
}
}
Simply place this on any text input/area control like so :
<input type="text" noWhitespace />
And you will now validate that the control has a value *and* that the value is not just whitespace. Easy!
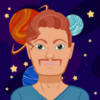
💬 Leave a comment