Importing Lodash into your Angular project may be slightly controversial in some circles, but I often find myself adding it (and adding the few KB that comes along with it), rather than reinventing the wheel in each project. If you’ve never used it before, Lodash is a javascript library that provides handy data manipulation extension methods for arrays/collections. If you’ve used C# LINQ, you’ll probably be familiar with many of the methods like OrderBy, GroupBy, Join etc. It’s also important to note that it pays to check if the default Array type in Javascript has what you need as there is a little bit of overlap (For example Find exists on both an array and Lodash).
But that’s not all! One of the most common use cases for Lodash is actually manipulating objects that aren’t arrays. For example, an extremely common use-case for me is using the _.clone() method which copies all the values of an object into a new object that’s safe for editing. This is extremely common for me when I’m doing two way data binding on a form that a user can “cancel”, so I still have the original object in tact.
In anycase, this post isn’t a pros and cons guide to using Lodash, it’s about adding it to your Angular project, so let’s get on and do that!
Adding Lodash To Angular
The first thing you want to do is add the Lodash package to your project using NPM/Yarn.
NPM
npm i lodash --save
Yarn
yarn add lodash
This adds Lodash to our project and at this point is ready for use, but it’s just the raw library. What we also need is the type definitions to give us some nice strongly typed defintions inside Typescript. For that we need to install one more package.
NPM
npm i --save-dev @types/lodash
Yarn
yarn add @types/lodash --dev
Note that we only add the type definitions as a dev dependency as it is not required at runtime, only while you are developing your project.
Anywhere in your project, you should now be able to import Lodash like so :
import * as _ from 'lodash';
let myItem = {};
let clonedItem = _.clone(myItem);
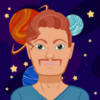
💬 Leave a comment