RXJS 7 was recently released to much fan fare, but buried deep inside the release was the deprecation of the “toPromise()” method on the Observable type.
For example, this sort of code :
let myPromise = myObservable.toPromise();
Now gives you the following warning :
@deprecated — Replaced with firstValueFrom and lastValueFrom . Will be removed in v8
That last point is the most important thing here. Not only is it deprecated in RxJS 7, but come version 8, it will be completely gone. That’s big, because often libraries deprecate methods but keep them around for backwards compatibility, but not this time!
Luckily, there is a simple work around that doesn’t require much of a change at all to how you were already using toPromise().
Using FirstValueFrom and LastValueFrom
FirstValueFrom and LastValueFrom are new utility methods inside RxJS 7 that more or less give you the same functionality as toPromise(), just in a different format. To use them, simply import from rxjs and give them a whirl!
import { firstValueFrom } from 'rxjs';
let myPromise = firstValueFrom(myObservable);
firstValueFrom does exactly what it says on the tin, it waits for the very first result from the observable, returns it as a promise, and unsubscribes from the subject.
lastValueFrom however, waits until “complete” is called on the subject, and then returns the last value it received. It’s important to note that if your subject never calls complete, then your promise will never be resolved.
Why?
The obvious question would be, why? Why remove toPromise() in favor of adding static “helper” methods. There’s actually a simple reason.
When using toPromise(), if the Observable completed without ever emitting any value at all, the Promise would resolve with a value of undefined. However, when you were using this in code, it was impossible to tell if the promise itself returned undefined, or it returned nothing. This has been the case ever since RxJS was released.
To be able to differentiate between no value, first value, or last value from the Observable, it was decided a static method, off the Observable prototype was a better solution than changing the behaviour of the toPromise() method all together.
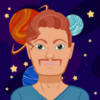
💬 Leave a comment