It’s pretty common in an Angular project to add the ability to “listen” for router events. That is, know that a user is about to (or already has) navigated to a new page. The most common reason is to push pageview events to analytics platforms (think Google Analytics or Azure Application Insights), but I’ve also used them to hook into whether a user is navigating away from a page (And stop them from losing their work), or to hide toaster/error messages if a user has moved away from a particular page.
In anycase, it’s actually fairly trivial to subscribe to router events.
The first thing to do is to inject the Router object into your service/component like so :
constructor(private router: Router)
Then to subscribe, we simply subscribe to a filtered list of navigation events like so :
this.router.events.pipe(filter(event => event instanceof NavigationEnd)).subscribe((event: NavigationEnd) => {
//Do something with the NavigationEnd event object.
});
Notice that we are filtering only on NavigationEnd events, this is because the events stream from the router is *all* Router events. The main events you want to subscribe to are listed below (Although there are a couple more, I personally never really use them).
- NavigationStart – Triggered when a navigation starts
- NavigationEnd – Triggered when navigation ends successfully
- NavigationError – Triggered when navigation ends with an error
- NavigationCancel – Triggered when a navigation is cancelled (Often because a router guard returned false)
- GuardsCheckStart – Triggered before guards are run as part of the routing
- GuardsCheckEnd – Triggered at the end of guards running as part of the routing
The most important ones I’ve used are NavigationStart, to determine if a user is about to navigate away, and NavigationEnd when a user has successfully browsed to a new page. It’s also important to keep in mind NavigationError, as NavigationEnd will only trigger if a user was successfully able to visit a page, but you may be missing analytics of failed pages if you ignore NavigationError.
And that’s it! Now you can subscribe to all sorts of different router events with just a couple of lines of code!
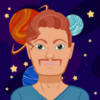
💬 Leave a comment