I was recently banging my head against the wall for a very annoying error that only occurred on my build server, but not locally. The error in question was when a component referenced an external style sheet, I was getting the following error :
Couldn’t Resolve Resource ./foo.css Relative To foo.component.ts
I’m going to go through a tonne of solutions here, any one of them could be your solution so don’t dismiss any of them until you’ve given it a go. The basis for this error is that whatever build tool you are using (Gulp or Webpack), can’t find your style file. In some rare cases, this also applies to your component not being able to find your template html, and all of the following solutions still apply.
You Are Using Inline Styles
If you are building templates using inline styles, then often it can be a bit of a copy paste error when you end up with something like so :
@Component({
selector : 'app-header',
templateUrl : './header.component.html',
styleUrls : ['h1 { float:left }']
})
And this may look very obvious but it’s happened to me before! If you are looking to use inline styles, you should change that styleUrls to be styles like so :
@Component({
selector : 'app-header',
templateUrl : './header.component.html',
styles : ['h1 { float:left }']
})
You Are Not Using Any Styling At All
The next solution is actually similar to the previous. Imagine that you don’t want to be using any styling at all, so you simply delete the style file and blank out the URL like so :
@Component({
selector : 'app-header',
templateUrl : './header.component.html',
styleUrls : ['']
})
This will still in some cases (Depending on your build pipeline) blow up. If you are intending to not use any styles at all, just completely remove the styleUrls all together like so :
@Component({
selector : 'app-header',
templateUrl : './header.component.html'
})
Relative vs Absolute Paths
I mostly see this in projects that move from things like Gulp to Webpack or vice versa. Even more so again when people are copy and pasting code from other projects. In some cases, Gulp for example likes absolute paths like so from the very root of your project.
@Component({
selector : 'app-header',
templateUrl : './core/layout/header.component.html',
styleUrls : ['./core/layout/header.component.css']
})
Whereas WebPack prefers relative paths from where the component.ts file is located. So if the css file is in the same folder we can change everything to be relative :
@Component({
selector : 'app-header',
templateUrl : './header.component.html',
styleUrls : ['./header.component.css']
})
However, again, this is totally dependant on what build tool you are using. The majority of projects will be using Webpack and therefore require relative paths, but just incase, and if you aren’t sure, try the absolute URL style yourself just to rule it out.
Case Sensitivity
This one has caught me out plenty of times as a Windows developer who typically has build agents running Linux. Windows is case-insensitive for file paths. Therefore this will build completely fine on a Windows machine :
@Component({
selector : 'app-header',
templateUrl : './header.component.html',
styleUrls : ['./hEaDeR.component.css']
})
However it will blow up when building on a Linux machine. The solution is obviously pretty simple, either rename the styleUrls to the correct casing *or* rename the file in Source Control (Which in of itself can be difficult on Windows). Either way, don’t discredit a small casing issue completely blowing things up.
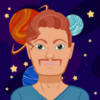
💬 Leave a comment