You may be wondering how to link to an external page in a NextJS application? It’s actually quite simple to do that, but you may be a little bit confused with the built-in Link components. In this article, I will show you how to add a link that’s pointing to an external URL.
Link component
In NextJS we have access to a built-in Link component. You might have thought that you can use this component to implement a redirection to an external website. Well, as a matter of fact, you actually cannot do that.
Link is meant to be used for handling navigation within the scope of your application, so you can use it to link between different pages. If you want to learn more about Link and its use cases then make sure that you familiarise yourself with the Routing in NextJS.
Well, to be completely honest you can use the Link to handle this functionality, but you really shouldn’t be doing that. Technically the below code snippet will work as expected.
import Link from 'next/link'
export default function Home() {
return (
<div>
<Link href="https://en.wikipedia.org/wiki/Next.js">NextJS wikipedia</Link>
</div>
)
}
External link
Since this will be an external Link we can simply use the anchor tag to handle this functionality. This won’t be any different web application. Just like in the example below.
export default function Home() {
return (
<div>
<a href="https://en.wikipedia.org/wiki/Next.js">NextJS wikipedia</a>
</div>
)
}
You may also notice that Link doesn’t support all of the properties of the regular anchor tag. For example, you don’t have an easy way to open the link in the new tab by providing the target property.
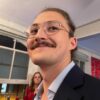
💬 Leave a comment