Fiber is a reconciliation algorithm used in the popular JavaScript library React to efficiently update a web application’s user interface (UI). It was introduced in version 16.0 of React in 2017 and has significantly improved the performance of React applications.
What is reconciliation in React?
In React, when the state of a component changes, the component needs to update its UI to reflect the new state. This process of updating the UI is called reconciliation.
React uses a Virtual DOM (VDOM) to perform reconciliation, which is used to compare a component’s current and previous states.
What is the Virtual DOM?
The VDOM is a lightweight in-memory representation of the actual DOM.
When the state of a component changes, React compares the VDOM of the last and current states and calculates the minimum number of DOM operations required to update the actual DOM to match the current VDOM.
The Catch
This helps reduce the number of DOM manipulations and improve the application’s performance. However, there are specific scenarios where the reconciliation process can become inefficient.
For example, suppose a component has many elements that need to be updated. In that case, the reconciliation process can take a long time and cause the UI to become unresponsive – This is where Fiber comes in.
What is Fiber?
Fiber is a new reconciliation algorithm introduced in React 16.0 that aims to improve the performance of React applications by making the reconciliation process more efficient.
It does that by allowing the reconciliation process to be broken down into smaller chunks and scheduled over multiple frames rather than being completed in a single frame.
Fiber divides the reconciliation work into smaller units called “fibers“.
What is a Fiber?
Each Fiber represents a single element in the VDOM tree, and the reconciliation process is performed on each Fiber individually.
This allows React to prioritize the reconciliation of certain fibers over others, depending on the importance of the updates.
For example, suppose a component has many elements that need to be updated. In that case, React can prioritize the reconciliation of the elements that are visible to the user while deferring the reconciliation of the other elements until later.
This helps ensure that the UI remains responsive even when there are a large number of updates.
Fiber in Asynchronous Programming
Fiber also introduces a new concept called “suspense”. Suspense allows React components to “wait” for a specific condition to be met before rendering.
This can improve the performance of applications that rely on asynchronous data, such as fetching data from a server.
How does Fiber work?
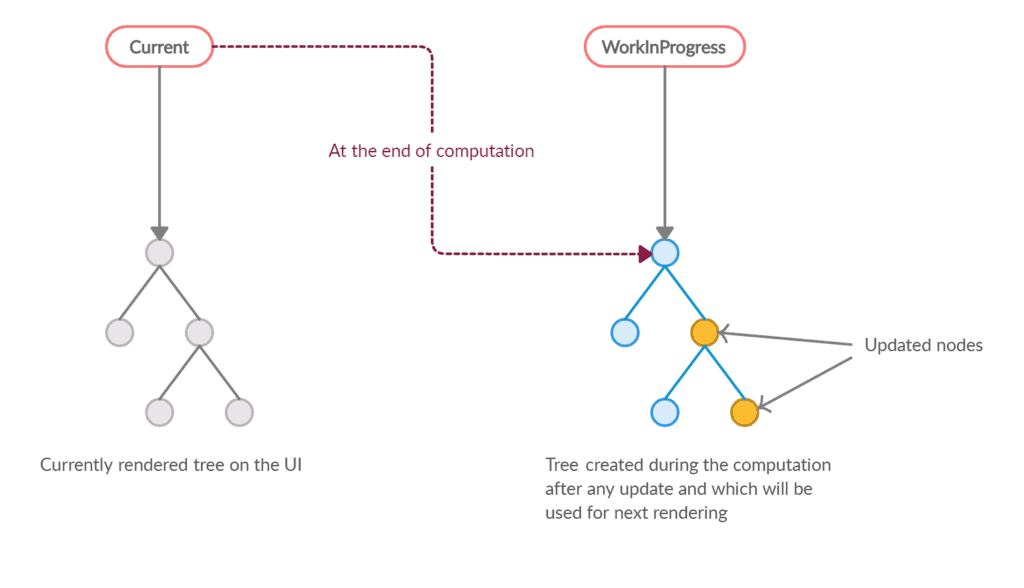
Fiber works by maintaining a linked list of fibers called the “fiber tree“.
Each Fiber in the tree represents a single element in the VDOM tree and contains information about the element, such as its type, props, and state.
When a component’s state changes, React begins the reconciliation process by creating a new fiber tree based on the current state of the component. It then compares the new fiber tree to the previous fiber tree to determine the minimum number of changes required to update the actual DOM.
During the reconciliation process, React traverses the fiber tree and updates each Fiber individually. It does this by starting at the root fiber and working its way down the tree, updating each Fiber in a depth-first order.
As it traverses the fiber tree, React can pause the reconciliation process at any point and schedule the remaining work for the next frame. This allows React to prioritize the reconciliation of certain fibers over others and ensure that the UI remains responsive.
Benefits of Fiber
Fiber brings several benefits to React applications, including:
Improved Performance
As mentioned earlier, Fiber allows the reconciliation process to be broken down into smaller chunks and scheduled over multiple frames, which helps improve React applications’ performance, especially when a large number of elements need to be updated.
Better user experience
By prioritizing the reconciliation of certain fibers over others, Fiber helps ensure that the UI remains responsive, even when there are a large number of updates. This leads to a better user experience.
Asynchronous rendering
The introduction of Suspense in Fiber allows React components to “wait” for a specific condition to be met before rendering, which can be used to improve the performance of applications that rely on asynchronous data.
If you’d like to know more about Suspense in React, which is related to the Asynchronous rendering mentioned earlier, I’d recommend checking the article we’ve written here.
Better concurrency
Fiber allows React to interrupt the reconciliation process at any point and schedule the remaining work for the next frame. This helps improve the concurrency of React applications, as it allows React to perform updates in parallel with other tasks.
Summary
In summary, Fiber is a reconciliation algorithm used in React to improve the performance of web applications.
It does this by allowing the reconciliation process to be broken down into smaller chunks and scheduled over multiple frames and by introducing the concept of suspense, which allows React components to “wait” for a specific condition to be met before rendering.
Fiber brings several benefits to React applications, including improved performance, a better user experience, asynchronous rendering, and better concurrency.
In case you have any suggestions or questions in regards to anything I’ve discussed in this article, or you wish to leave any kind of feedback, feel free to drop a comment below, and we’ll discuss further.
Cheers!
💬 Leave a comment