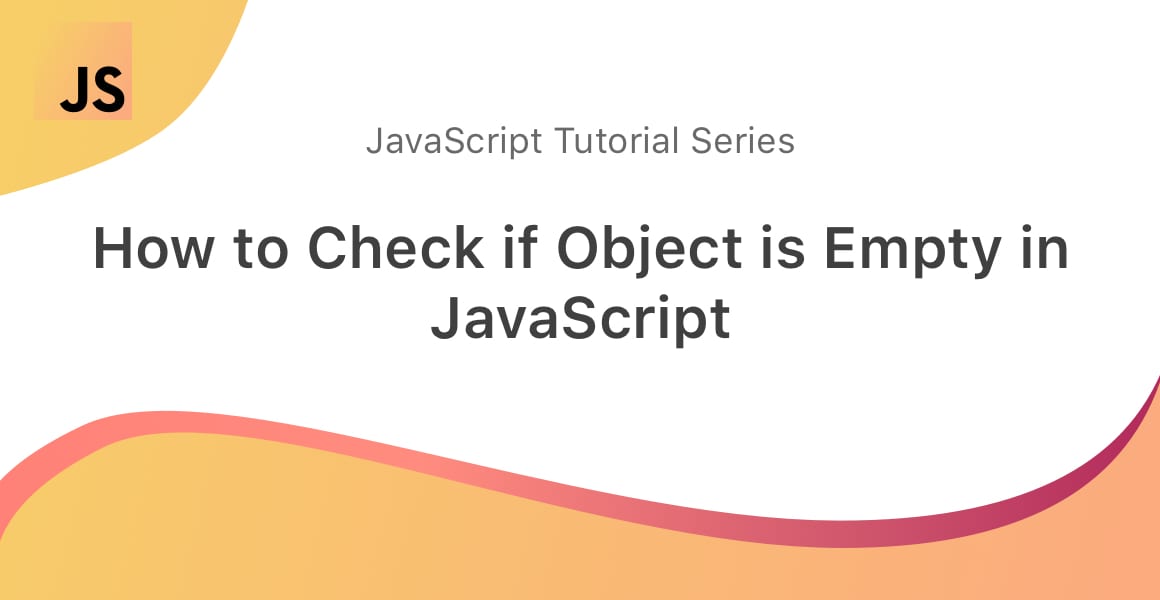
I’ll show you how to check if an object is empty in JavaScript. Checking if an object is empty is not as simple as checking if an array is empty.
The surprising fact is, it’s not as obvious as you might think to check if an object is empty in JavaScript.
Let’s take the following empty sample object named person, below:
const person = {}
A plain and simple JavaScript object, initialized without any keys or values. Empty.
There are multiple ways to check if the person object is empty, in JavaScript, depending on which version you are using.
ES6 is the most common version of JavaScript today, so let’s start there. ES6 provides us with the handy Object.keys function:
Object.keys(person).length
The Object.keys function returns an array containing enumerable properties of the object inside of the parentheses. In our case, the array for the person object will be empty, which is why we then check the length of the array.
Simple, effective, concise. Let’s see it in action!
const person = {}
if (Object.keys(person).length === 0) {
// is empty
} else {
// is not empty
}
The above works for empty objects like our person object, but it won’t work for new Date() objects. Object.keys(new Date()).length === 0 will always return true.
If you are using ES7 (the newer version of ECMAScript), you can use the following function:
Object.entries(obj).length === 0
Similarly, we can use the Object.entries function like so:
const person = {}
if (Object.entries(person).length === 0) {
// is empty
} else {
// is not empty
}
It’s exactly the same as Object.keys, however if you’re using ECMAScript 7 or higher, you’ll need to use Object.entries.
If you want to explore more JavaScript tutorials, why not check out my tutorial on how to remove a character from a string.
💬 Leave a comment