Have you ever wondered if there is an easy way to use CSS with JS in Svelte? This is a pattern that’s very often used when working with other JS libraries, like React where you can use the styled-components or Classnames. The same is possible with Svelte, but it’s not as obvious to realise at a first glance. Let me show you how you can accomplish that!
Emotion
The first step is to install the emotion package in our app via npm. You can do this by running the below command
$ npm i emotion
in the root directory of your application. Now we can go ahead and write the styles.
Implementation
I have created a new styles.js file in which I’m importing using the emotion package to define and export new styles to be used in my application.
import { css } from '@emotion/css'
const color = 'purple'
export const wrapper = css`
display: flex;
flex-direction: column;
align-items: center;
`
export const title = css`
color: ${color};
`
export const paragraph = css`
font-size: 20px;
`
As you can see we are also using the JS variable to demonstrate how we can use it with the CSS.
In App.svelte, we have written a simple application that will consume those styles.
<script>
import { wrapper, title, paragraph } from "./styles.js";
</script>
<div class={wrapper}>
<h1 class={title}>Hello Upmostly!</h1>
<p class={paragraph}>
Hey there! Come over to <a href="https://upmostly.com/">Upmostly.com</a> to check
out many interesting articles about web development
</p>
</div>
As you can see we are importing the previously defined style classes in the script tag and then injecting them into the class tags of the relevant HTML elements.
Below you inspect the output of our application. You can see that all of our styles were applied just like we expected them to.

Now go ahead and try to incorporate this approach in your application!
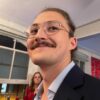
💬 Leave a comment