Styles are essential to any modern web page. They regulate the sizes, shapes, colors, and features of UI elements on-screen.
While we can add styles in a CSS file (and then reference it in our React code), React gives us the option to add these styles directly into elements using inline styles.
In this tutorial we’ll go over inline styles with React in JSX, which allow us to code in styles in a higher-level, more intuitive way.
First, we’ll set up a standard web app using create-react-app. Then we’ll create a new file, called Element.js, and reference it in App.js:
function Element( props ) {
return(
<div>
{props.name}
</div>
)
}
export { Element }
Now, we’ll clear the boilerplate code in App.js and add a couple references to the Element we just created:
import { Element } from './Element'
function App() {
return (
<div>
<Element name="Element 1"/>
<Element name="Element 2"/>
</div>
);
}
export default App;
To completely clear any external styles, we’ll get rid of the CSS import in Index.js:
import React from 'react';
import ReactDOM from 'react-dom';
import App from './App';
ReactDOM.render(
<React.StrictMode>
<App />
</React.StrictMode>,
document.getElementById('root')
);
Now, when we run the code, we get the following web page in the browser:
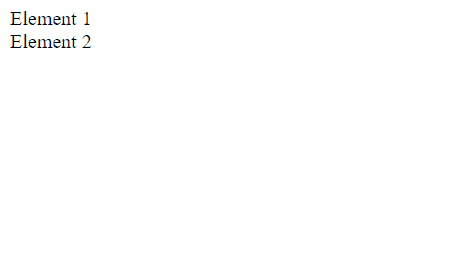
The elements are styled with HTML’s standard settings. We’ll now use inline styles to add a modern look.
First, we’ll demonstrate how to use inline styles to change color. We’ll set the text color of all elements to red in Element.js:
function Element( props ) {
return(
<div style={{color: 'red'}}>
{props.name}
</div>
)
}
export { Element }
The result is:
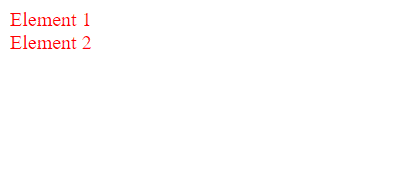
In the above code, we used the “style” attribute in the <div> JSX element to add inline styles. Then, we used a double curly brace before specifying the styles to use, which in this case was just a setting of color to red.
The double braces are important, as each set of curly braces does something different. The outer set specifies that we are using a variable; since the return portion is written in JSX, our code won’t know that we are setting the attribute to a variable unless we use curly braces around it.
The inner set of curly braces specifies a JavaScript object. JavaScript objects are mappings between keys and values; in this case, we are mapping the key color to the value “red”.
Notice also that we applied the inline style to a JSX <div> rather than a JSX React element. The “style” attribute is a special attribute in React which applies only to HTML JSX tags.
Now, let’s add a fuller set of styles to Element.js:
function Element( props ) {
return(
<div style={{
backgroundColor: 'gray',
fontFamily: 'Arial, Helvetica, sans-serif',
width: 'max-content',
padding: '10px',
margin: '5px',
border: '1px solid black',
borderRadius: '5px'
}}>
{props.name}
</div>
)
}
export { Element }
We get the following in our browser:
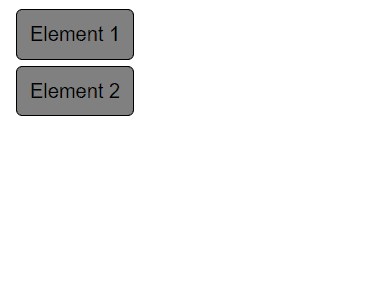
The code structure is exactly the same, but with more styles within the double curly brace, separated from each other with commas.
Notice how we put different styles on different lines, adding the newline after the comma. This is a good programming practice, allowing us to see clearly all the styles that are being applied. The alternative is to have a very long set of styles all on one line, which is not very legible.
What if we wanted to be able to specify different background colors for our elements? Since we can’t use the style attribute directly on our React JSX elements, we have to use a workaround via props.
First, we’ll add a new “backgroundColor” attribute in each of our elements in App.js:
import { Element } from './Element'
function App() {
return (
<div>
<Element name="Element 1" backgroundColor="red"/>
<Element name="Element 2" backgroundColor="green"/>
<Element name="Element 3"/>
</div>
);
}
export default App;
Now, we’ll rewrite Element.js to utilize the new attribute via props:
function Element( props ) {
var backgroundColor;
if ( props.backgroundColor ) {
backgroundColor = props.backgroundColor;
} else {
backgroundColor = 'gray';
}
return(
<div style={{
backgroundColor: backgroundColor,
fontFamily: 'Arial, Helvetica, sans-serif',
width: 'max-content',
padding: '10px',
margin: '5px',
border: '1px solid black',
borderRadius: '5px'
}}>
{props.name}
</div>
)
}
export { Element }
In the above code, we add an if/else statement that will try to use “props.backgroundColor” to set the variable “backgroundColor”. If there is no “props.backgroundColor”, the value of “backgroundColor” defaults to “gray”.
Then, we set the “backgroundColor” style in our return statement to the variable “backgroundColor”. Notice that we don’t have to add curly braces to it this time around, since the set of curly braces that we added before wraps around our variable reference.
We get the following output in our browser:
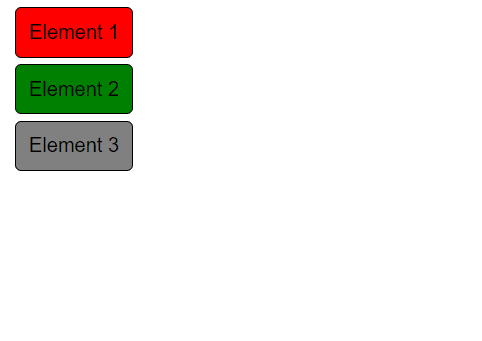
Now we can set the colors of specific elements!
And that’s it! Have fun using React’s inline styling!
💬 Leave a comment