Your website can feel more contemporary and provide a better user experience by using Vue Transitions and Animations. Fortunately for developers, setting up a Vue animation only takes a few minutes.
After reading this tutorial, you’ll be able to use Vue’s transition element, know how to use it to make various animations within this framework, and understand how to incorporate them into any code you’re working on.
First, we’ll take a look at Vue Transition; and then, we’ll develop our CSS animation styles. Last but not least, we’ll demonstrate how to combine Vue animations with JS webhooks in order to handle more complex animations.
Let’s get started!
What is Vue Transition and why is it important?
The majority of people believe that transitions are merely decorative. However, a well-designed transition can accomplish a number of different things:
- Draw the attention of your users and direct it.
- Make crucial information clear.
- While on your website, suggest a natural flow and orient users while they’re navigating in it.
- Assist you in developing a more credible brand image.
All of these ideas will assist in enhancing the UX on your website, as well as improving your CRO and retention rates. So, you can say animations and transitions are kind of a big deal.
Sounds good? Let’s learn how to use transitions in Vue now that we are aware of how useful they can be for your website.
Enhancing Your Project Using Vue Transition
VueJS provides a few ways to implement transitions out of the box in order to cater to a wide range of developers:
- CSS style for animation transitions
- JavaScript hooks for DOM modification
- Including external CSS/JS libraries
Each of them has varying degrees of difficulty depending on your prior knowledge. For example, transition/animation styling is something you’ll like using if you have more HTML/CSS familiarity.
On the other hand, manually modifying the DOM is the way to go if you are switching from React or in case you’re a vanilla JavaScript lover and love tinkering with DOM manipulation on your own.
To begin with, we’ll concentrate on handling a single element with CSS for the time being. But don’t worry, we’ll cover more complicated topics later on.
The Transition element, the key to Vue transitions
Vue’s transition element is a wrapper that enables you to give your elements transition behavior. In essence, it adds different functionalities to the elements you want to animate, allowing you to style them later during various transitional phases.
When working with Transition, we can use 6 different classes out of the box:
- “v-enter-from” & “v-leave-from”: This class is deleted once the transition begins. Their mission is to jumpstart an animation.
- “v-enter-active” & and “v-leave-active”. These refer to the transition’s active state.
- “v-enter-to” & “v-leave-to”. These mark the transition end state.
How to use Vue Transition
Now that we’re familiar with the transition classes, it’s pretty simple to write your own template code for a Vue Transition. Simply choose the element you wish to animate, and then enclose it in a <transition> component.
In this illustration, we’ll make a button that instructs an <h2> element to show up after we click it.
<template>
<div>
<h1>Vue Transition Example</h1>
<button @click="show = !show">Show Header</button>
<transition name="show">
<h2 v-if="show" class="h2-show">Our Transition Works!</h2>
</transition>
</div>
</template>
<script>
export default {
data() {
return {
show: false,
};
},
};
</script>
Pretty simple, right? Now just a few more styles need to be added to make the transitions functional.
Per Vue documentation’s sample styling, here’s what we have to do in our <style> section:
<style>
.show-enter-active,
.show-leave-active {
transition: opacity 0.8s ease-in;
}
.show-enter-from,
.show-leave-to {
opacity: 0;
}
</style>
See how the prefix “show” appearns in every one of our classes? That comes from the transition name we’ve specified before!
What does this code do right now? Because it connects classes with comparable states, it is actually quite simple to understand.
According to these styles, once you click the button the opacity attribute will change gradually over 0.8s. Here’s the result:
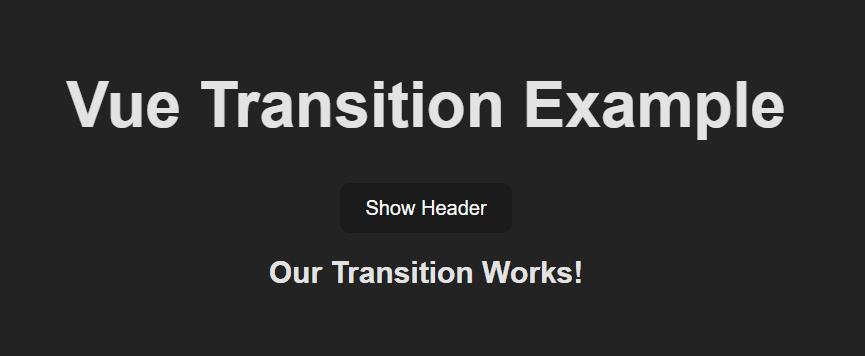
Simple, wasn’t it?
By the way, you can utilize CSS animations in addition to CSS transitions in the same way.
JS Hooks and Custom Class Names
By including any one of these six classes to our element, we may also replace any of the default names:
- “enter-from-class”
- “enter-active-class”
- “enter-to-class”
- “leave-from-class”
- “leave-active-class”
- “leave-to-class”
When incorporating third party libraries into your code, or if you’re building your own animation libraries, this is extremely helpful.
In addition to that, the transition element emits JS hooks that we may intercept and use to implement our animations in JavaScript rather than CSS. The hooks readily available are:
- “before-enter” & “before-leave”
- “enter” & “leave”
- “after-enter” & “after-leave”
- “enter-cancelled” & “leave-cancelled”
When these hooks trigger, we can very easily “catch them” in our JavaScript and then add any animation we want.
Let’s set up a slide animation on a heading element in the main App component as an example. The functions that alter the text’s color will be combined with the enter and after-enter hooks:
<template>
<transition appear name="move" @enter="enter" @after-enter="afterEnter">
<h1>Changing color with Transition Hooks</h1>
</transition>
</template>
<script>
export default {
methods: {
enter(el) {
el.style.color = "blue";
},
afterEnter(el) {
el.style.color = "red";
},
},
};
</script>
<style>
.move-enter-from,
.move-leave-to {
opacity: 0;
transform: translateY(-50px);
}
.move-enter-active,
.move-leave-active {
transition: all 2s ease-out;
}
</style>
In this case, our header will begin on the page as blue and change to red once the animation ends. Notice that we’re combining both the Transition element we saw before with two webhooks in order to create a more complex animation.
As you can see, creating transitions in Vue is very easy thanks to its built-in classes and webhooks. In this tutorial we have shown you how to do it by using CSS classes and JS webhooks.
If you want to learn more about how to use Vue, take a look at our tutorials. You will find all the information you need to create amazing websites and applications. Thanks for reading!
💬 Leave a comment