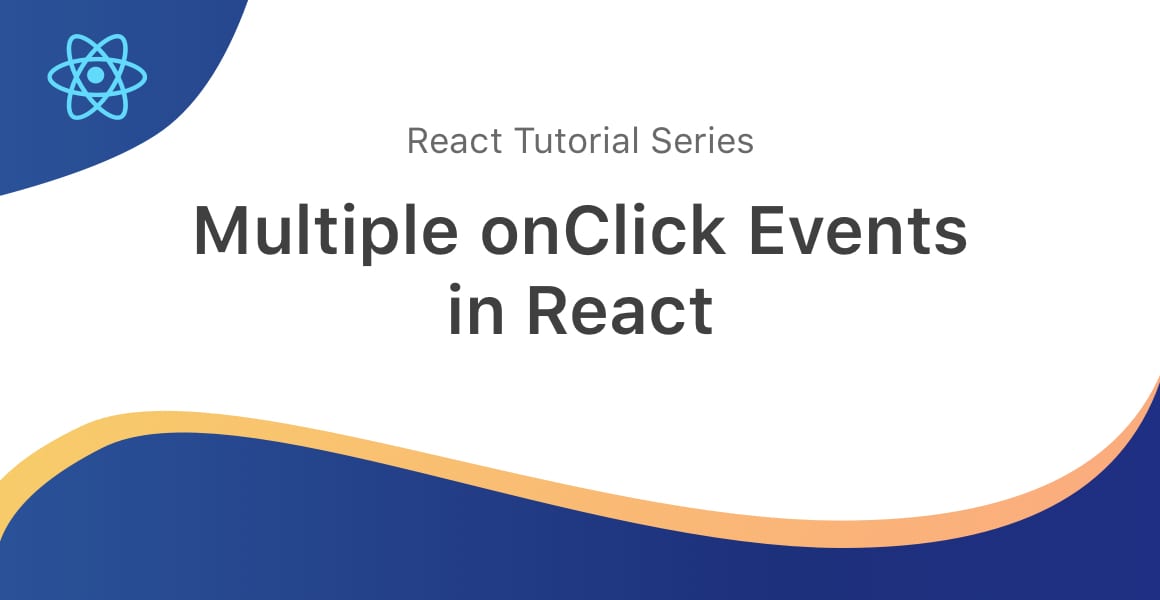
There are several ways to call muliple onClick events in React, each with its own pros and cons. Let’s explore each one and learn how they work in React!
- Call a Function onClick in React
- Call Multiple Functions onClick in React
- Write Logic Inside of onClick Event Handler in React
Calling multiple functions after clicking a button is a very common thing for a React developer to want to perform.
As a result, React provides the onClick event handler to attach to a UI element to detect when it is clicked.
- Call a separate, single function and include all of your actions inside of that function (Best option).
- Use an inline function inside of the onClick event handler and call multiple functions onClick.
- Write your logic inside of an inline function that’s inside of the onClick event handler (Worst option).
The first way to perform multiple onClick events in React is to write your logic in a single function, and then call that function inside of the onClick event handler.
To learn more about the onClick event handler in React, check out my tutorial on React onClick Event Handling with Examples.
The second way to is to call multiple functions inside of the onClick event handler in React.
The third option, is to write your logic inside of an inline function that’s inside of the onClick event handler. This is the least recommended way to solve this problem. I’m only including it here so that you know all the ways of making multiple actions inside of an onClick event handler.
Each solution has its own pros and cons. Let’s take a look at code samples for all three, and the reasons why you’d want to perform each one over the other.
Call a Function onClick in React
✔ Good
The first solution to perform multiple onClick events in React is to include all of your actions inside of a function and then call that single function from the onClick event handler.
Let’s explore how to do that in a React Component:
import React from 'react';
function App() {
function greeting() {
console.log('hello!');
waveHello();
}
function waveHello() {
console.log('wave');
}
return (
<div>
<button
onClick={greeting}>
I'm a button
</button>
</div>
);
}
export default App;
There are several reasons why you’d want to perform multiple onClick events using this method.
- Your code is easier to read and understand
- Logic code is seperate from view code
- The React Component’s onClick handler is not re-compiled when the Component re-renders (unlike with inline functions)
It’s extremely important to remember the second point, that logic code should be separated from view code.
De-coupling our view code from the logic code is extremely important as our UI changes but the logic does not. Therefore, we won’t need to re-write our components as often if logic code is not closely coupled with the view code.
Call Multiple Functions onClick in React
This isn’t the best option out of the three, but there may be situations where it is necessary.
import React from 'react';
function App() {
function greeting() {
console.log('hello!');
}
function waveHello() {
console.log('wave');
}
return (
<div>
<button
onClick={() => {
greeting();
waveHello();
}}>
I'm a button
</button>
</div>
);
}
export default App;
The example above may look similar to the first solution, but it’s very different. Take a look at the button onClick event handler. We use an inline function inside of the onClick to call multiple functions.
Write Logic Inside of onClick Event Handler in React
❌ Bad
Your view and logic code should be de-coupled as much as possible. This promotes cleaner, modular code, and allows your View library to be switched out if necessary.
I’m only showing you how to do this so you are aware that writing code like this in React is a bad idea.
import React from 'react';
function App() {
return (
<div>
<button
onClick={() => {
console.log('hello!');
console.log('wave');
}}>
I'm a button
</button>
</div>
);
}
export default App;
Well, there you have it. Three solutions to the same problem. Don’t you just love web development?
💬 Leave a comment