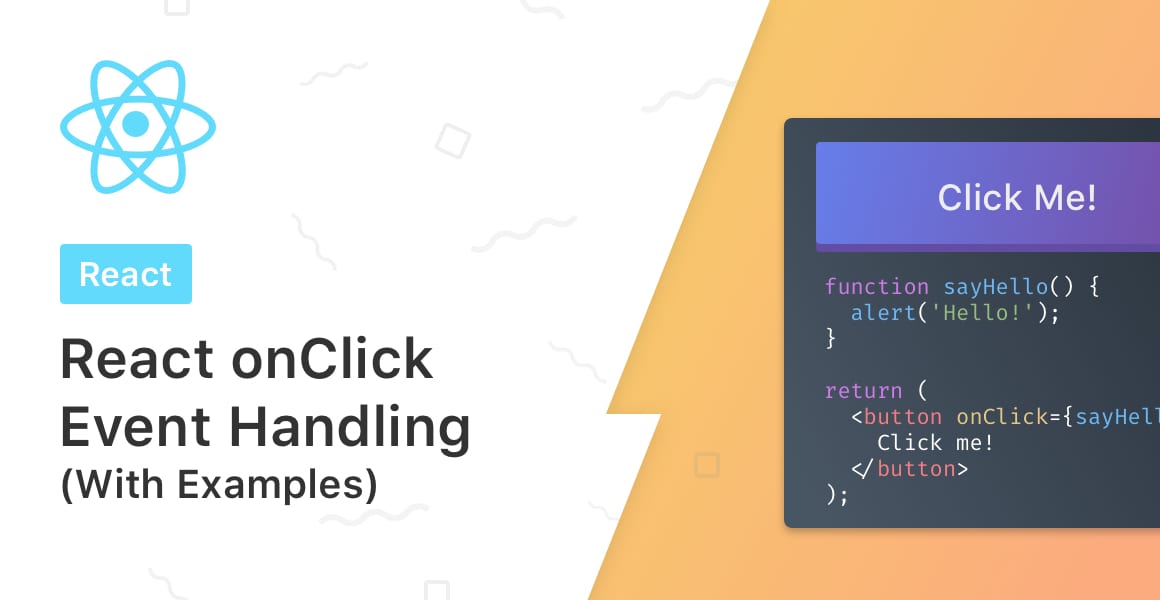
In React, the onClick handler allows you to call a function and perform an action when an element is clicked. onClick is the cornerstone of any React app.
Click on any of the examples below to see code snippets and common uses:
- Call a Function After Clicking a Button
- Call an Inline Function in an onClick Event Handler
- Call Multiple Functions in an onClick Event Handler
- Update the State inside an onClick Event Handler
- Pass a Button Value to an Inline Function
- Pass a Value from an Array to an Inline Function
If you’re new to React, I suggest you read our excellent beginner tutorial: Build a Todo App in React Using Hooks
What is the React onClick Event Handler?
Whenever you need to perform an action after clicking a button, link, or pretty much any element, you’ll use the onClick event handler.
Therefore, the onClick event handler is one of the most powerful and most used tools in your React tool belt.
Let’s look at some examples of how we can use the onClick event handler in React.
Example: Call a Function After Clicking a Button
import React, { Component } from 'react';
class App extends Component {
constructor(props) {
super(props);
this.sayHello = this.sayHello.bind(this);
}
sayHello() {
alert('Hello!');
}
return (
<button onClick={this.sayHello}>
Click me!
</button>
);
}
export default App;
import React from 'react';
function App() {
function sayHello() {
alert('Hello!');
}
return (
<button onClick={sayHello}>
Click me!
</button>
);
}
export default App;
The simple App component above has one function called sayHello(), and a single button.
The button inside the React component has an onClick event handler attached to it, pointing to our sayHello() function. Doing so will trigger the function every time you click the button.
How do we trigger the sayHello() function? By passing in the name of the function you want to call inside the curly braces, after the event handler:
<button onClick={sayHello}>Click</button>
Common Mistakes
Notice how we only pass the name of the function to the event handler and not the name followed by parentheses. Parentheses after a function name executes the function.
In other words, if we passed in the function with parentheses, the function will execute every time the component renders.
We do not want to execute a function inside of the event handler.
✅ Good
<button onClick={sayHello}>
Click me!
</button>
❌ Bad
<button onClick={sayHello()}>
Click me!
</button>
Example: Call an Inline Function in an onClick Event Handler
An inline function is a function which is defined inside of the onClick handler when the React Component renders.
It’s created on render because the function definition is inside of the onClick handler, which is inside of the component render method (or return, in the case of functional React components).
You’d use an inline function to pass in additional arguments to a function, such as a value from a loop, or the target button’s value. Let’s take a look at an example:
import React from 'react';
function App() {
return (
<button onClick={() => alert('hello'))}>
Click me!
</button>
);
}
export default App;
Common Mistakes
A common mistake when using inline functions is not including parentheses after the function name.
Unlike calling a function that’s not inline, one that’s defined inside of the component’s body, we need to execute the function if it’s inline.
✅ Good
<button onClick={() => functionName())}>
Click me!
</button>
❌ Bad
<button onClick={() => functionName}>
Click me!
</button>
Example: Call Multiple Functions in an onClick Event Handler
You may find yourself wanting to call multiple functions after clicking a button. For example, updating a component’s state and simultaneously showing an alert.
There are multiple ways to do this:
- Running a block of code inside the onClick handler
- Calling multiple functions inside the onClick handler
Let’s start with defining a block of code inside of the onClick handler itself:
<button onClick={() => {
const name = 'James';
alert('Hello, ', name);
}}>
Click me!
</button>
As the example above shows, it is possible to define a block of code inside of the onClick handler. However, depending on the amount of code that you want to run, the block scope can be difficult to read.
Similarly, calling multiple functions inside of the onClick handler is also possible:
<button onClick={() => {
this.sayHello();
this.setState({ name: "James"});
}}>
Click me!
</button>
Again, the code above is not as readable as it could be if we just called the sayHello function and set the state inside of that. However, there may be instances when you need to do the above.
Example: Updating the State in an onClick Event Handler
A very common use of an inline function inside of an onClick event handler in React is to update a component’s state.
You’ll do this when you want to update the state with the button’s value, or using a value from a loop, for example. Let’s take a look at how we can update the state inside of an onClick event handler:
import React, { Component } from 'react';
class App extends Component {
constructor(props) {
super(props);
this.state = {
count: 0,
};
}
return (
<button onClick={() => this.setState({ count: 1})}>
Click me!
</button>
);
}
export default App;
import React, { useState } from 'react';
function App() {
const [count, setCount] = useState(0);
return (
<button onClick={() => setCount(1)}>
Click me!
</button>
);
}
export default App;
Continue learning about updating state in React components through the onClick event handler in Simplifying React State and the useState Hook.
Example: Pass a Button Value to an Inline Function
Another common use of an inline function is to pass in a button’s value as an argument. This is also very common when using input elements and the onChange event handler.
Take a look at the example below. Notice the value e that’s returned from the onClick event handler:
import React from 'react';
function App() {
return (
<button value="hello!" onClick={e => alert(e.target.value)}>
Click me!
</button>
);
}
export default App;
The value e is what’s called a SyntheticEvent. Every event handler is passed a SyntheticEvent, which is an object that contains useful metadata related to the event, such as the target element’s value.
The code above accesses just that, the target element’s value, and shows it inside a browser alert. The e.target.value is originating from the value attribute that’s on the actual button element.
Take some time to explore the SyntheticEvent further as there are more useful values inside of it. You could start by console logging e out and clicking through the object.
Example: Pass a Value from an Array to an Inline Function
The final example we’ll explore is how to pass in a value from an array to an inline function that’s inside of the onClick event handler.
One common use of arrays in React is to render multiple components per item in the array.
For example, an admin dashboard which displays a list of users and provides admins with the ability to edit or delete users:
import React from 'react';
function UserList() {
const users = ['James', 'Nora', 'Matthew', 'Joe', 'Susan'];
function deleteUserWithName(name) {
...
}
return (
<ul>
{users.map(name => (
<li>
<button onClick={() => deleteUserWithName(name)}>
Click me!
</button>
</li>
))}
</ul>
);
}
export default UserList;
The code above first defines a new array called users with a list of first names. Then, it loops through those names, rendering a list and button element for each value inside the array.
Finally, it passes in the name from the array as an argument of the deleteUserWithName function.
💬 Leave a comment