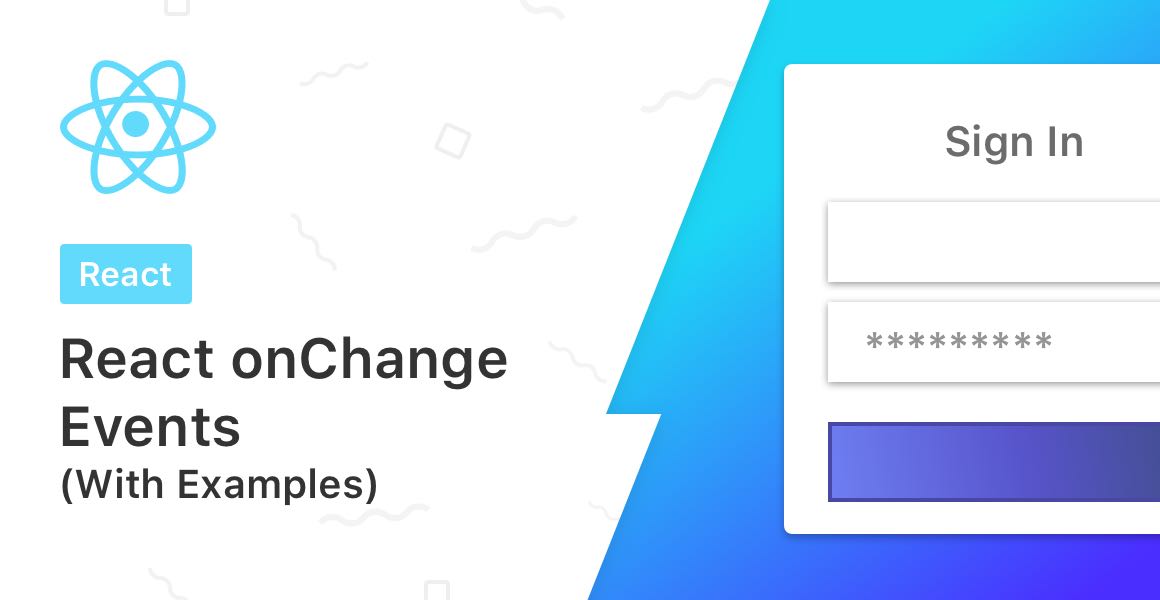
React provides us with some really useful utilities. One of them is the normalized event system that it provides. Today we are going to look at one of events — The onChange event. The onChange event in React detects when the value of an input element changes. Let’s dive into some common examples of how to use onChange in React.
- Add an onChange Handler to an Input
- Pass an Input Value to a Function in a React Component
- Storing an Input Value Inside of State
What is the onChange Event Handler?
JavaScript allows us to listen to an input’s change in value by providing the attribute onchange. React’s version of the onchange event handler is the same, but camel-cased.
If you’re using forms inside of a React component, it’s a good idea to understand how the onChange event handler works with forms, state, and how you can pass the value to a function.
Event handlers are an important part of React. Learn more about other Event handlers such as the onClick, onDrag, onHover or the onKeyPress event.
Example: Add an onChange Handler to an Input
Let’s begin with a simple, but extremely important example: how to add an onChange event handler to an input, checkbox, or select element in a React component:
...
return (
<input name="firstName" onChange={handleChange} />
);
...
The above code displays a single input field which, when typed in, passes its current value to the handleChange function. As mentioned before, JavaScript’s native onchange syntax is written in all lowercase, however we use camel-case for our event handler names in React.
Example: Pass an Input Value from the onChange Event in a React Component
Let’s expand on the previous example by declaring a new function inside of our React component for the onChange handler to pass a value to. We’ll call it handleChange, and have it log the input’s current value to the console:
import React from 'react';
function App() {
function handleChange(event) {
console.log(event.target.value);
}
return (
<input name="firstName" onChange={handleChange} />
);
}
export default App;
An onChange event handler returns a Synthetic Event object which contains useful meta data such as the target input’s id, name, and current value.
We can access the target input’s value inside of the handleChange by accessing e.target.value. Therefore, to log the name of the input field, we can log e.target.name.
Log the whole event object to the console and click through it to see what other useful information it provides.
The example above was of a functional component. If you’re using a Class component, you will have to bind the onChange event handler to the context of this. To learn more about the differences between Functional components and Class-based components check out this guide.
Example: Saving an Input Value Inside of State using onChange in a React Component
The final example we’ll explore today is how to store an input’s current value inside of a state value. We are going to use the useState hook provided to us by React. You can learn more about the useState hook here.
This is extremely useful for keeping track of the current input fields values, and displaying them on the UI.
import React from 'react';
function App() {
const [firstName, setFirstName] = useState('');
return (
<input value={firstName} name="firstName" onChange={e => setFirstName(e.target.value)} />
);
}
export default App;
So basically, what we did here is attach the setFirstName setter function to the onChange event handler which passes us the value of the input under e.target.value which is then set to the firstName which sets the value of the input and the cycle continues… Thank you for following along and if you are new to React don’t forget to check out this tutorial on how to create your first React app
💬 Leave a comment