JSX is a really useful feature of React. It makes building complex interfaces so much easier. We can easily visualise the would-be DOM structure of our application and build the relations between components much easier. But if you’re a real hardcore developer then you may want to try developing without JSX
createElement
Using JSX elements is equivalent to calling
React.createElement(component, props, ...children)
every single time we want to use one element. So nothing really stops us from rewriting
function App() {
return (
<h1>Hello from Upmostly</h1>
);
}
export default App;
to
import React from 'react'
function App() {
return (
React.createElement('h1', null, 'Hello from Upmostly')
);
}
export default App;
In both cases, the output is exactly the same.
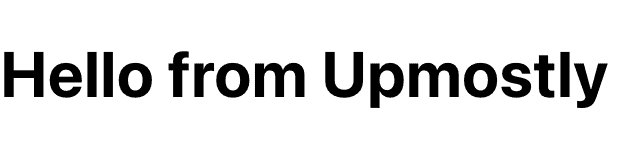
We can also call custom components in the same way. Let’s define a User component that takes some props and simply renders them to the screen.
const User = ({ name, surname, age }) => {
return (
<div>
<h2>Name: {name}</h2>
<h2>Surname: {surname}</h2>
<h2>Age: {age}</h2>
</div>
)
}
export default User;
In App.js we can then use it as follows.
import React from 'react'
import User from './User'
function App() {
return (
React.createElement(User, { name: 'Tommy', surname: 'Smith', age: 23 }, null)
);
}
export default App;
This will then render the below output to the page.
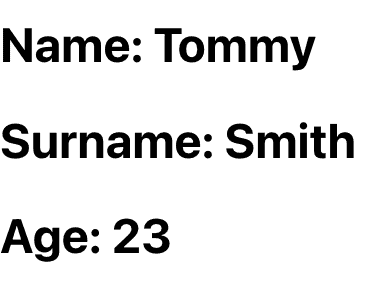
Don’t be getting any crazy ideas with developing in this way. It’s a fun fact to know, but you will probably not need to use createElement for a long long time!
💬 Leave a comment