If you know what API keys are then you also know why is it important for us to protect them from being stolen. This can cause a lot of issues. Every developer should know how to hide their API keys.
The most common way of exposing your keys to the public is by hard coding them in your project source code and later committing to a remote repository such as GitHub or GitLab. Using dotenv files can help you deal with this issue in a more efficient way.
It’s important to point out that although the method described in this article is a step in the right direction in terms of security. It’s not the most secure way of hiding your keys. Environment variables in React are embedded in the build, so any user can access them. A better solution would be to store them on the server.
Environment variable
We’ll hide our sensitive information by storing it in the .env or dotenv file. It’s a text config file that helps us control the constants across our projects.
You can create one in your by simply creating a new file and calling it.
.env
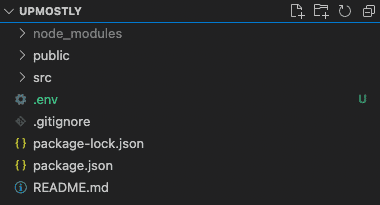
Example
Let’s take a look at the below application that makes a simple request that uses the API key.
import axios from 'axios';
import { useEffect } from 'react';
const veryPrivateKey = 'fdt43b24d34c43v256bvra4tsds4v3bv'
function App() {
const fetchData = () => {
const response = axios.get(`https://test.endpoint/key=${veryPrivateKey}`)
...
}
useEffect(() => {
fetchData()
})
return (
<div>
</div>
);
}
export default App;
In the above code snippet I have defined the veryPrivateKey as a constant in our application. We’re then using it in the fetchData function that makes a dummy GET request and uses our veryPrivateKey as a query param.
If I now went ahead and pushed my changes to a remote repo I would expose my veryPrivateKey to the public. Someone could then steal it and use it maliciously.
To prevent that, I will more that API Key into the .env file
REACT_APP_VERY_PRIVATE_KEY=fdt43b24d34c43v256bvra4tsds4v3bv
Notice that the variable name is written in the SCREAMING_SNAKE_CASE and has the prefix REACT_APP. The prefix is very important as any variable without it will be ignored.
Now you can simply use your variable by accessing it on process.env.<variable-name>
import axios from 'axios';
import { useEffect } from 'react';
function App() {
const fetchData = () => {
const response = axios.get(`https://test.endpoint/key=${process.env.REACT_APP_VERY_PRIVATE_KEY}`)
...
}
useEffect(() => {
fetchData()
})
return (
<div>
</div>
);
}
export default App;
The very last step would be to add the .env file to .gitignore to make sure that you will never commit it by accident.
💬 Leave a comment