Styling is a crucial part of developing any modern web application. The days of plain HTML websites are long gone. Now websites need to have beautiful designs in order to appeal to the users and seem trustworthy. Let’s take a look at how you can style your React applications.
The below article doesn’t present a complete list of all the ways how to style React apps, but just a selected bunch of them that I personally find useful.
CSS files
The most obvious and simple way to style the React apps is by importing CSS files. This is actually the default way of doing things and if you used create-react-app to set up your project then you should have it all ready and working. Let’s take a look at your App.js and App.css files.
h1 {
color: pink;
}
.App {
text-align: center;
}
.App-logo {
height: 40vmin;
pointer-events: none;
}
@media (prefers-reduced-motion: no-preference) {
.App-logo {
animation: App-logo-spin infinite 20s linear;
}
}
.App-header {
background-color: #282c34;
min-height: 100vh;
display: flex;
flex-direction: column;
align-items: center;
justify-content: center;
font-size: calc(10px + 2vmin);
color: white;
}
.App-link {
color: #61dafb;
}
@keyframes App-logo-spin {
from {
transform: rotate(0deg);
}
to {
transform: rotate(360deg);
}
}
In the above code snippet taken from App.css, you can see that we are using the regular CSS to style the React application. Just like in any other web application, make sure that the selectors are actually targeting relevant elements and voila! You have just styled your React app.
To prove my point I have added a custom code styling the h1 element at the top of the file. It matched the h1 element that I had added in the JSX of App.js
import logo from './logo.svg';
import './App.css';
function App() {
return (
<div className="App">
<header className="App-header">
<h1>Hello from Upmostly</h1>
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
);
}
export default App;
Let’s take a look to see if it applied the relevant styles.
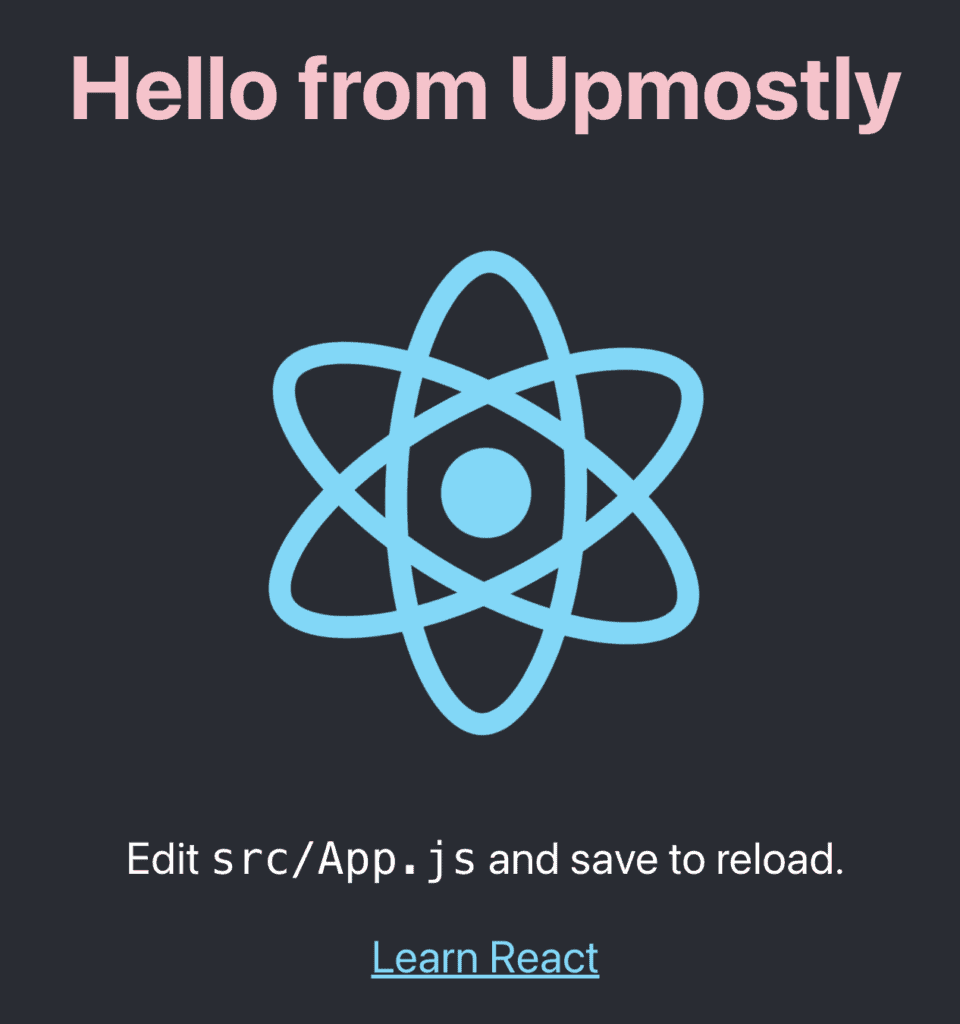
Looks like it did! All the magic happens because of the
import ‘./App.css’;
statement at the top of App.js. This line of code is responsible for importing the stylesheets. Now comment it out and see what happens.
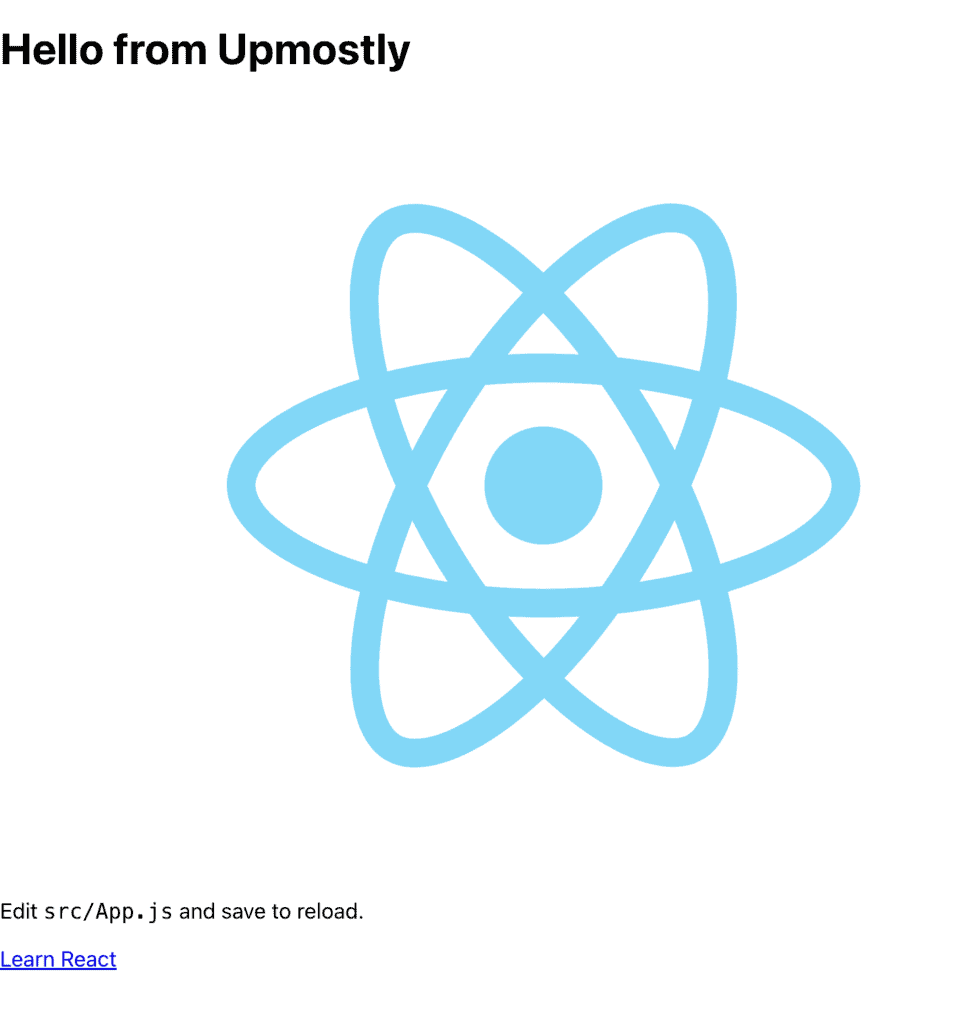
It doesn’t look quite the same, does it?
Inline styling
This technique is particularly useful when trying to implement really tiny styling changes here and there. Let’s undo the changes that we implemented in App.css to have a fresh start. Let’s say that this time we want to style that h1 element to be red. We can easily accomplish it by using the style property.
import logo from './logo.svg';
import './App.css';
function App() {
return (
<div className="App">
<header className="App-header">
<h1 style={{ color: 'red' }}>Hello from Upmostly</h1>
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
);
}
export default App;
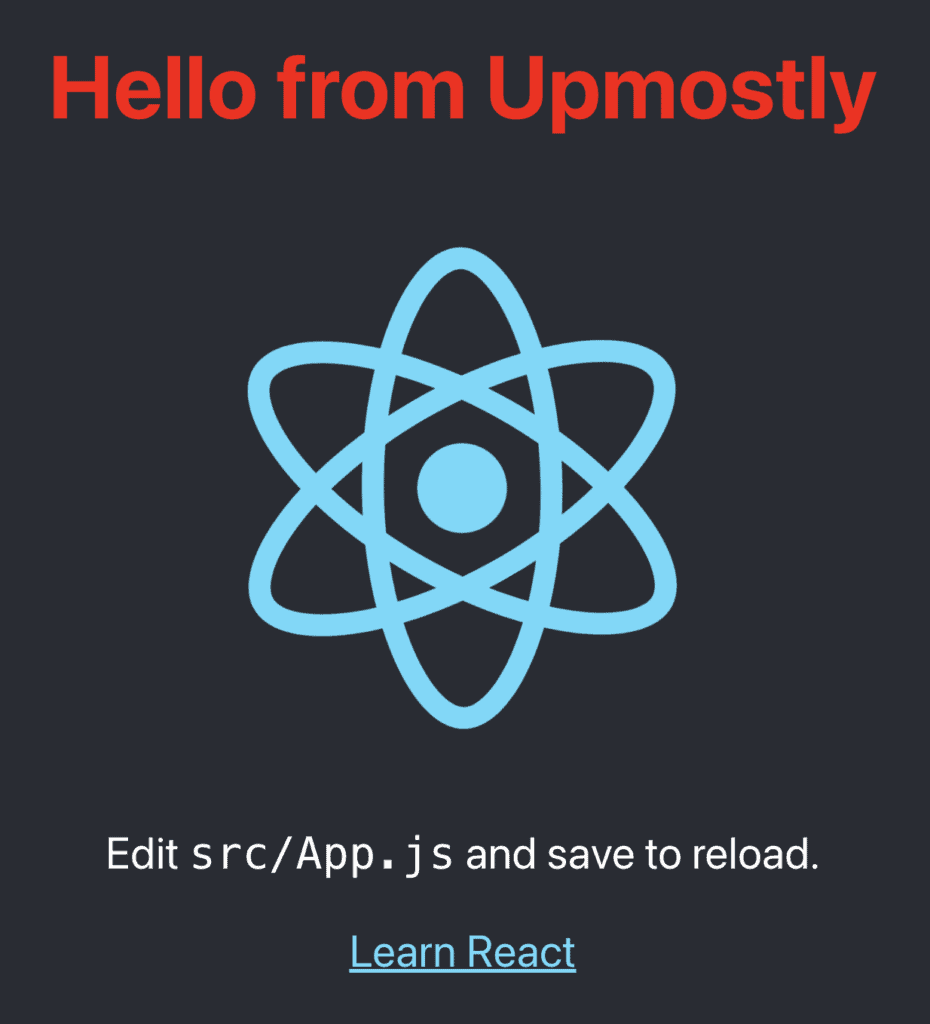
It’s important to point out that the style property doesn’t accept the regular CSS code. Instead, it expects an object, which properties are camelCased names of the CSS properties. The values are to be provided as strings. For more accurate reference please check out the official docs on style by React.
Styled Components
Have you ever heard of styled-components? If not then your whole world is about to change! It’s my favourite way to style React code. You’re gonna see why!
PS. For a more detailed explanation of styled-components please check out Osman’s article. It covers the library in more detail.
You can install styled-components by running
$ npm install –save styled-components
in the root directory of your React application.
Then we can simply define our new YellowHeader component and start using it across the application. Let’s take a quick look at the code.
import logo from './logo.svg';
import styled from 'styled-components'
import './App.css';
function App() {
return (
<div className="App">
<header className="App-header">
<YellowHeader>Hello from Upmostly</YellowHeader>
<img src={logo} className="App-logo" alt="logo" />
<p>
Edit <code>src/App.js</code> and save to reload.
</p>
<a
className="App-link"
href="https://reactjs.org"
target="_blank"
rel="noopener noreferrer"
>
Learn React
</a>
</header>
</div>
);
}
export default App;
const YellowHeader = styled.h1`
color: yellow
`
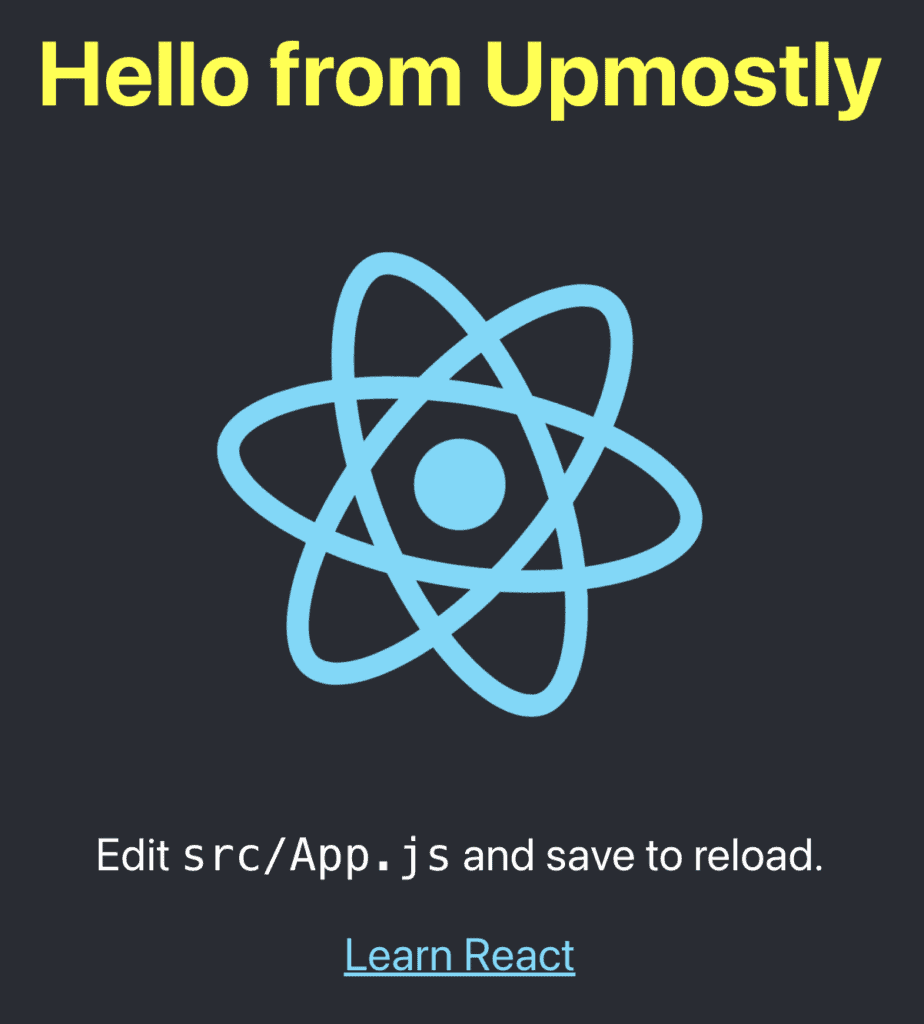
Those are the three most basic ways to style React applications. It’s important to note that this list is not exhaustive. There are many more options out there. Some of which are going to be covered in more detail in the upcoming articles here at Upmostly!
💬 Leave a comment