Sometimes we need to pass data from a child component to parent component. For example we can have an input child component and a parent that shows the input when input is updated. Let’s make an example of it.
Create a react app with create-react-app and create an Input.js file:
src/Input.js
import React from 'react';
import styled from 'styled-components'
const Input = ({
changeState
}) => {
return (
<StyledInput
type="text"
onChange={changeState}
/>
)
}
const StyledInput = styled.input`
width:20rem;
height:2rem;
border-radius:0.5rem;
border:2px solid black;
margin:5% 0 0 20%;
outline:none;
`
export default Input
The child component takes a function prop, and this function actually updates the state of the parent component. Jump into App.js and modify it like this:
src/App.js
import React, { useState } from 'react';
import Input from './Input';
import styled from 'styled-components';
const App = () => {
const [text, setText] = useState("");
const changeState = (e) => {
const value = e.target.value;
setText(value);
}
return (
<>
<StyledText>{text}</StyledText>
<Input
changeState={changeState}
/>
</>
)
}
const StyledText = styled.div`
margin:0 0 0 20%;
`
export default App
As you can see, we have a text state and a changeState function. We pass changeState function to the Input component, that way the child component is able to pass data to the parent component.
The output looks like this:
When input is “Hello”:
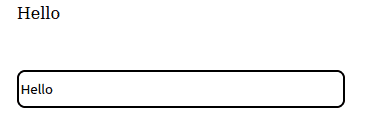
When input is “Hello World !”:
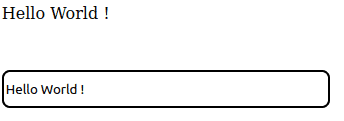
Summary:
Basically, we pass an event handler function to our child component, that modifies the parent component’s state.
That’s it for this article, I hope you enjoyed and learned a lot.
💬 Leave a comment