Navigation is a core element of every web application. It seems like a daunting task for new engineers and in this article, I will try to explain the process step by step.
Redirect
The simplest form of navigation that we can use is to redirect the user to some other resource or page. We’ll use the simple example of writing a static blog post, which redirects to Wikipedia.
function App() {
return (
<div>
<p>
React (also known as React.js or ReactJS) is a free and open-source front-end JavaScript library for building user interfaces based on UI components. It is maintained by Meta (formerly Facebook) and a community of individual developers and companies. React can be used as a base in the development of single-page, mobile, or server-rendered applications with frameworks like Next.js. However, React is only concerned with state management and rendering that state to the DOM, so creating React applications usually requires the use of additional libraries for routing, as well as certain client-side functionality.
</p>
<a href="https://en.wikipedia.org/wiki/React_(JavaScript_library)" target="_blank">
Read more about React
</a>
</div>
)
}
export default App
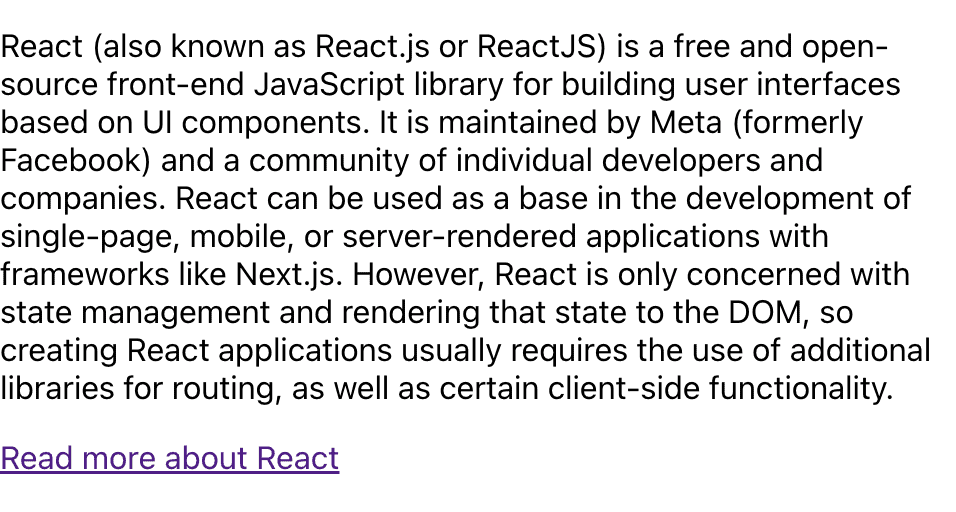
Our application displays a simple description of React (taken from Wikipedia). It also uses the <a> tag to display the clickable link for the user. The href property points to React’s Wikipedia page. When the user clicks on the link a new tab is opened in the browser and the user is taken to the predefined page that’s provided to that href property.
We’re also using target property with value _blank. This tells the browser that we want the link to be opened in a new tab. You can play around with this property and see how the link behaves without it.
Navigation
Navigation within the react application is a bit more difficult process. To handle it we will use the 3rd party library called the React Router. We can install it in our app by running
npm install react-router-dom@6
in the root folder of our project. Now we can start using it in our project.
I have written a simple foo bar navigation app. It has 3 basic routes. Let’s take a look at how it works.
import { render } from "react-dom";
import { BrowserRouter, Routes, Route } from "react-router-dom";
import { Home, Foo, Bar, BarWithID } from "./App";
const rootElement = document.getElementById("root");
render(
<BrowserRouter>
<Routes>
<Route path="/" element={<Home />} />
<Route path="foo" element={<Foo />} />
<Route path="bar" element={<Bar />} />
</Routes>
</BrowserRouter>,
rootElement
);
In the index.js we are importing BrowserRouter, Routes and Route from freshly installed react-router-dom. BrowserRouter is rendered at the top level of our application. It wraps all other JSX elements of the application. This is crucial for the routes to be working correctly.
Next, we’re using Routes to define the list of individual Route elements. This instructs our React Router on which components to render based on the current URL provided in the browser navigation bar.
import { Link } from "react-router-dom";
export const Home = () => {
return (
<div>
<h1>Navigation example</h1>
<nav>
<Link to="/foo">Foo</Link> | <Link to="/bar">Bar</Link>
</nav>
</div>
);
}
export const Foo = () => {
return (
<div >
<h2>Foo</h2>
</div>
);
}
export const Bar = () => {
return (
<div>
<h2>Bar</h2>
</div>
);
}
In App.js I have defined 3 components: Home, Foo and Bar. Home will be used at our starting point. We’re are creating a minimalistic navigation bar there. The first Link element points to the /foo URL and the second one to the /bar.
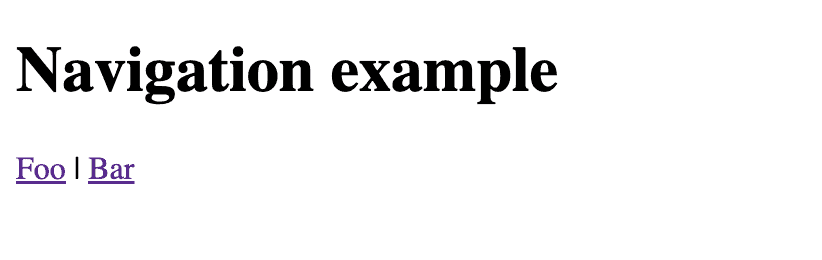
Our browser URL tab currently points to localhost:3000. When we click on either of those links the application will use the to property from Link and push the resulting path to the URL bar. React router will then kick in and load the corresponding component as defined in the index.js.
Let’s click on Foo to navigate to localhost:3000/foo
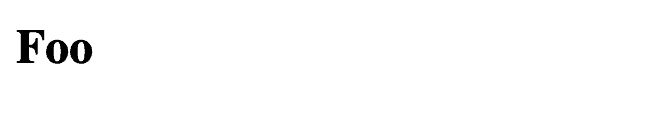
and now let’s click on Bar to navigate to localhost:3000/bar
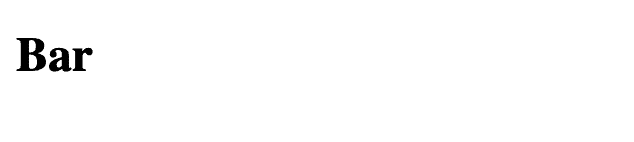
Tada! Looks like everything is working as expected. Now let’s take our app one step further and add support for params. Those can be used to read URL params dynamically and make our application depend on those.
import { render } from "react-dom";
import { BrowserRouter, Routes, Route } from "react-router-dom";
import { Home, Foo, Bar, BarWithID } from "./App";
const rootElement = document.getElementById("root");
render(
<BrowserRouter>
<Routes>
<Route path="/" element={<Home />} />
<Route path="foo" element={<Foo />} />
<Route path="bar" element={<Bar />} />
<Route path="bar/:id" element={<BarWithID />} />
</Routes>
</BrowserRouter>,
rootElement
);
We have added another route to the index.js ==> bar/:id. It will render the BarWithID component and pass the id param to that component. It’s important to use the colon symbol before the id to let the React Router that we expect the id to be dynamic.
import { Link, useParams } from "react-router-dom";
export const Home = () => {
return (
<div>
<h1>Navigation example</h1>
<nav>
<Link to="/foo">Foo</Link> | <Link to="/bar">Bar</Link>
</nav>
</div>
);
};
export const Foo = () => {
return (
<div>
<h2>Foo</h2>
</div>
);
};
export const Bar = () => {
return (
<div>
<h2>Bar</h2>
<Link to="/bar/1"> Bar 1</Link> | <Link to="/bar/2">Bar 2</Link> | <Link to="/bar/3"> Bar 3</Link>
</div>
);
};
export const BarWithID = () => {
const params = useParams();
return (
<div>
<h2>Bar with ID: {params.id}</h2>
</div>
);
};
We have expanded on the Bar component by adding 3 links, each pointing to the corresponding path. We have also added the BarWithID component at the very bottom. It uses the useParams hook provided by the react-router-dom to access the params in the URL and return them to be used in the application. In our simple example, we will simply render those to the screen, but in some more advanced applications, those have more meaning. A good example would be to use those params to fetch data stored in some database.
Let’s go to localhost:3000/bar
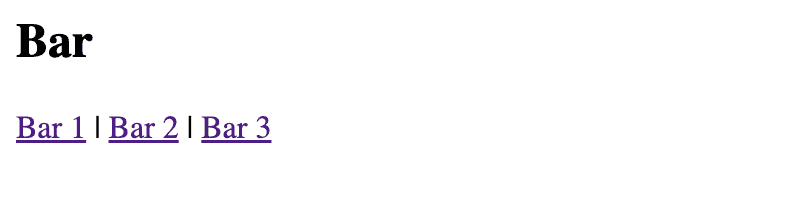
We can now navigate by clicking through the available links. They will display the BarWithID component with the relevant id.
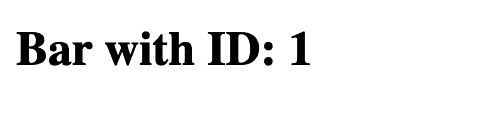
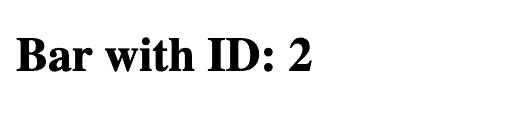
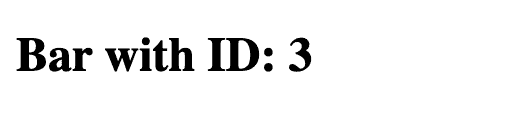
We have covered the most basic navigation use cases in our apps. Make sure to check the official documentation for the React Router. It’s an extremely popular framework that’s being used in production by many companies.
💬 Leave a comment