Running projects locally can sometimes be a tricky topic. New engineers do not tend to focus on learning how to set up the project correctly, but instead want to jump into the app development really quickly. Let’s take a quick look at how you can run your React project locally.
I understand that as a new developer you may not exactly be excited to learn about so many different tools. I have been there and I have delayed this step for as long as I possibly could have. However, it’s really important that you at least try to understand the very basics so that you could at least start the project.
package.json
You should first familiarise yourself with what exactly package.json is. In short, it’s the centre point of any Node project. It’s a JSON file that helps to identify your project and manage any dependencies. When it comes to running the React application itself, you should focus on the scripts section.
Below you can find the package.json file that’s been freshly generated by the create-react-app tool.
{
"name": "scripts-tutorial",
"version": "0.1.0",
"private": true,
"dependencies": {
"@testing-library/jest-dom": "^5.16.2",
"@testing-library/react": "^12.1.4",
"@testing-library/user-event": "^13.5.0",
"react": "^17.0.2",
"react-dom": "^17.0.2",
"react-scripts": "5.0.0",
"web-vitals": "^2.1.4"
},
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject"
},
"eslintConfig": {
"extends": [
"react-app",
"react-app/jest"
]
},
"browserslist": {
"production": [
">0.2%",
"not dead",
"not op_mini all"
],
"development": [
"last 1 chrome version",
"last 1 firefox version",
"last 1 safari version"
]
}
}
Under the scripts section, you can find 4 different key-value entries. Those are:
- start
- build
- test
- eject
These entries are terminal commands, we’re storing them in the package.json file to document them and reuse them easily. You can invoke them by running $ npm run <key> from the root directory of the react application.
A different project will have different scripts defined, so you should make sure to always check the package.json file to check which scripts are available. Sometimes developers put more information in the README.md file so make sure to look there first.
I want you to focus your attention on start and test as that’s what you’ll most likely be interested in.
"scripts": {
"start": "react-scripts start",
"build": "react-scripts build",
"test": "react-scripts test",
"eject": "react-scripts eject"
}
VS Code terminal
To open the VS Code built-in terminal navigate to the Terminal > New Terminal in the settings bar. This should bring up the terminal from the bottom of your screen.
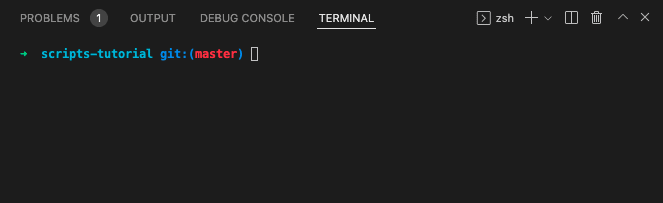
Now you can go ahead and run the start script by running
$ npm run start
You should now see the output saying that the project was successfully started.
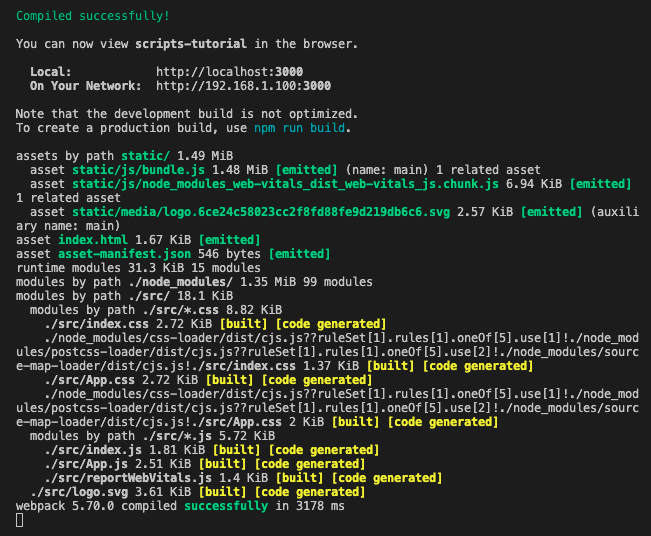
You can stop it by pressing control + C on your keyboard. Now go ahead and run
$ npm run test
to start the script that will run the tests.
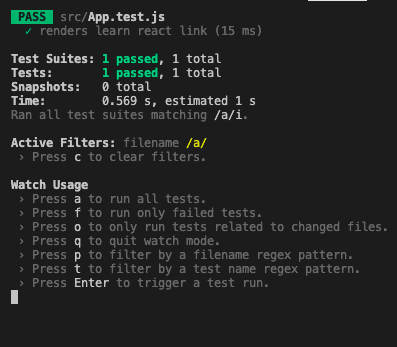
💬 Leave a comment