React is a component-based library. We have states and we render a UI that’s synced with our states. For example, let’s think of an error scenario. We have an input tag for a person’s age, when we click to submit button, the age should be shown on the side. But, when the user tries to give a negative input or an input that’s more than 120, we’ll render an error and not submit.
What we’re trying to do is a conditional rendering of an error.
Let’s start building that project. Type this command in the terminal to create a React app:
npx create-react-app conditional-rendering
and after the React app is created, change directory into React app and type this command:
npm install styled-components
styled-components is a popular UI library that lets us write CSS code in our Javascript files.
For more info about styled-components, check out this link:
In the src folder of our app, change the App.js file like this:
src/App.js
import React, { useState } from 'react';
import styled from 'styled-components';
const App = () => {
const [currentInput, setCurrentInput] = useState(null);
const [allAges, setAllAges] = useState([]);
const [errorText, setErrorText] = useState(null);
const inputChangeHandler = (e) => {
const value = e.target.value;
setCurrentInput(value);
}
const submitHandler = () => {
if (currentInput < 0) {
setErrorText("Invalid age, negative ages are not accepted")
}
else if (currentInput > 120) {
setErrorText("Invalid age, maximum accepted age is 120")
}
else {
const newAgesState = [...allAges, currentInput]
setAllAges(newAgesState)
}
}
return <AppWrapper>
<InputWrapper>
<label htmlFor="ageInput">Age</label>
<input name="ageInput" type="number" onChange={inputChangeHandler} />
{errorText !== null ? <Error>{errorText}</Error> : null}
<button onClick={submitHandler}>Submit Age</button>
</InputWrapper>
<AgesColumn>
<AgesTitle>Ages</AgesTitle>
{allAges.map((age, index) => {
return <div key={index}>{age}</div>
})}
</AgesColumn>
</AppWrapper>;
};
const AppWrapper = styled.div`
display:flex;
flex-flow:row nowrap;
width:60%;
justify-content:space-evenly;
align-items:start;
`
const InputWrapper = styled.div`
display:flex;
flex-flow:column nowrap;
align-items:center;
justify-content:space-evenly;
width:20rem;
height:20rem;
border:2px solid black;
`
const AgesColumn = styled.div`
display:flex;
flex-flow:column nowrap;
align-items:center;
justify-content:space-evenly;
`
const AgesTitle = styled.div`
font-size:1.5rem;
font-weight:bold;
`
const Error = styled.div`
border:2px solid red;
width:auto;
color:red;
`
export default App;
Let’s test it, we can successfully submit number 30:
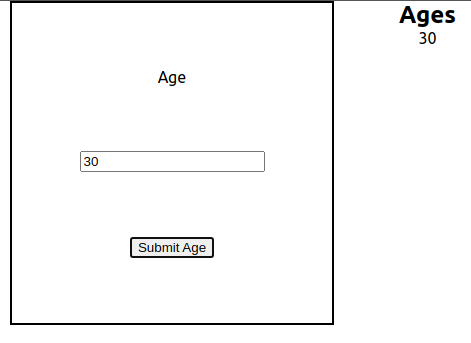
Now if we put -10 as input, we get render an error and submit isn’t successful:
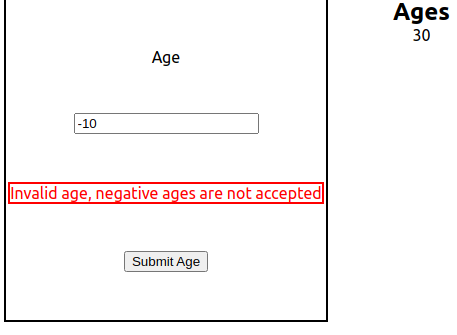
Now let’s try 200 as input, and we render an error again :
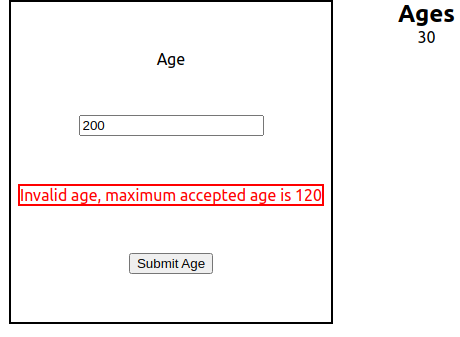
As you can see, we conditionally render an error message. Sometimes the error message is rendered, sometimes not. Sometimes it has different text, depending on the input.
We store the errorText as a state and only render error whenever errorText state is not null.
Let’s polish up the styles a bit and now the app looks better.
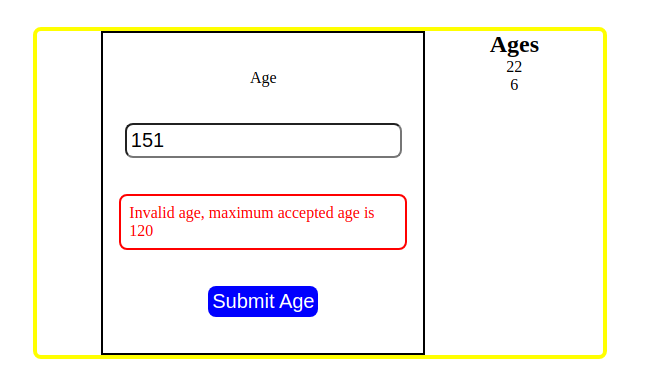
For the full source code for this app, go to: https://github.com/upmostly/conditional-rendering-react
This was a short but useful article about how to use conditional rendering in React. I hope you liked it.
💬 Leave a comment