I think it’s fair to say that everything in React revolves around props and state. If you’re a relatively young engineer then chances are that you have not been exposed to working with class components as much as you should have. Although they are no longer the default way of working with React, there are still a lot of legacy applications using class components.
Let’s take a quick look at how to work with props in class components specifically.
Using props
In class components, props are available on the props object that’s accessible via the this keyword. You can simply access them like you would properties on any other object.
import React from 'react'
class App extends React.Component {
render() {
return <h1>Welcome to {this.props.portal}!</h1>
}
}
export default App
In the above App.js example, we have written a simple class-based component and used the portal prop to help us render the application below.
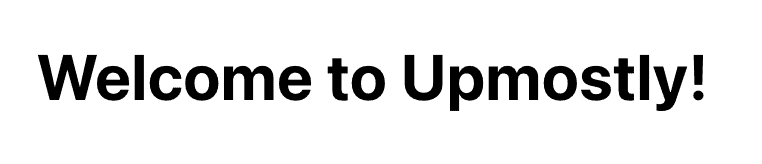
Destructuring props
Destructuring props is a very useful trick that’s commonly applied across big React projects. It results in a slightly cleaner syntax in JSX.
import React from 'react'
class App extends React.Component {
render() {
const { portal } = this.props
return <h1>Welcome to {portal}!</h1>
}
}
export default App
We have rewritten the previous example to take advantage of this patttern, as you can see we have destructured the portal prop from the props object and then use it directly in the application. You may not see much point in handling it this way, but you’ll see why it’s useful when you have to work with components that are rendering many props.
Default props
By using the destructuring assignment you can take advantage of another React feature; the default props.
import React from 'react'
class App extends React.Component {
render() {
const { portal = 'world' } = this.props
return <h1>Hello {portal}!</h1>
}
}
export default App
In the above code snippet, we have defined the default value for he portal prop. In case that the parent component don’t provide it, the portal prop will defaul to the value ‘world’. You can see the output of such case below.
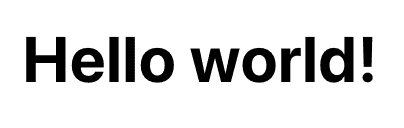
💬 Leave a comment