Props are a crucial part of React, as they are used to map the unidirectional flow of data from parents to children.
Props, which you can read more about here, stand for “properties”, and are a way for us to pass down state from a Parent Component down to a Child Component in order to alter its behavior (The child’s behavior).
Sometimes, however, due to certain constraints, or circumstances, we would need components to be able to handle situations where props might not be provided and at the same time, not break our application.
So, how can we avoid getting any warnings or issues while still being able to not pass props down to a child component?
Introducing Default Props
Default Props allow us to configure the behavior of a child component in the absence of defined, but not received properties by setting up a default value that will be used in case a certain prop will not be passed down from a parent.
It might sound like an anti-pattern, like “Why would we ever need to design our components to not need certain properties if we have already defined them and we have considered them in our logic processing?“;
Well, it might initially sound like it, but it isn’t, and we’ll look over a simple example that you might be able to abstract away and realize how plausible it might be further on.
Showcase of Default Props
We will look over a very simple application where we are rendering the data of a user to the main page based on a couple of props we are receiving on a UI component.
We’ve done that by creating a User
component that takes some props that represent a couple of styling properties, as well as properties of an user entity:
const User = ({ name, surname, age }) => {
return (
<div style={{ backgroundColor: 'beige', padding: '10px', marginBottom: '10px' }}>
<h2>Name: {name}</h2>
<h2>Surname: {surname}</h2>
<h2>Age: {age}</h2>
</div>
)
}
export default User;
We’ll then render this component inside of the App
component:
function App() {
return (
<div style={{ margin: '50px' }}>
<h1>Current user:</h1>
<User name='default name' surname='default surname' age='default age'/>
</div>
);
}
export default App;
Which will then generate the following UI:
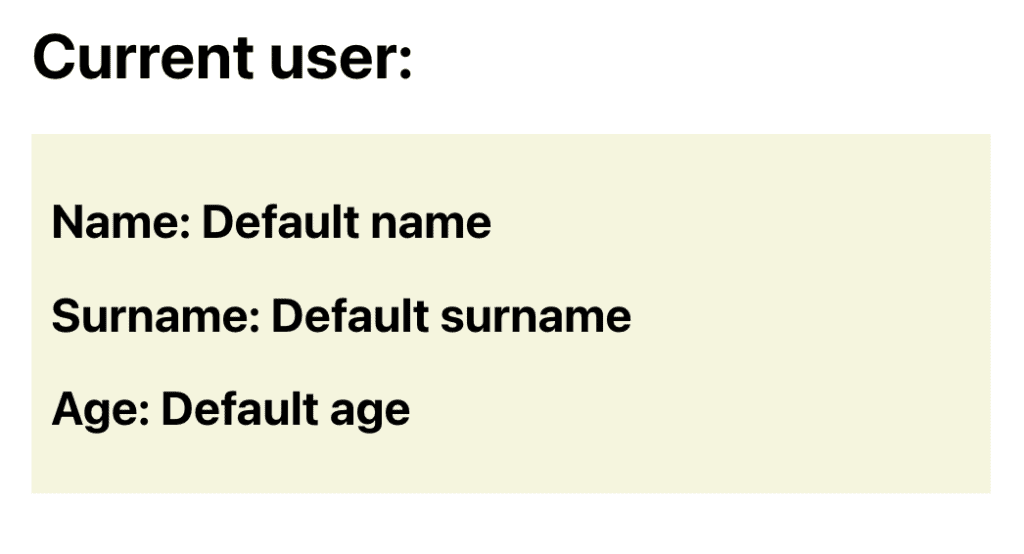
As you can see at the moment that we’re not really sure who the current user of the app is, and, as such, we have decided to pass some dummy data as props.
I would like for those values to be somehow saved so that we wouldn’t have to pass them every time we’ll need to render the User
component.
This can be achieved with the defaultProps
property available on the component itself.
It accepts an object where the key corresponds to the properties (props) names and values to the desired default values.
User.defaultProps = {
name: 'Default name',
surname: 'Default surname',
age: 'Default age',
}
We can now remove the manually passed props in the App.js and we’ll be able to see the same output as we previously did.
Let’s say we are aware of the user’s name and surname but we don’t know their age. We can pass those values to the component. React is smart enough to figure out that we only passed some of the props.
For the props for which we have not provided an explicit value, React will use their default values.
function App() {
return (
<div style={{ margin: '50px' }}>
<h1>Current user:</h1>
<User name="Tommy" surname="Smith"/>
</div>
);
}
export default App;
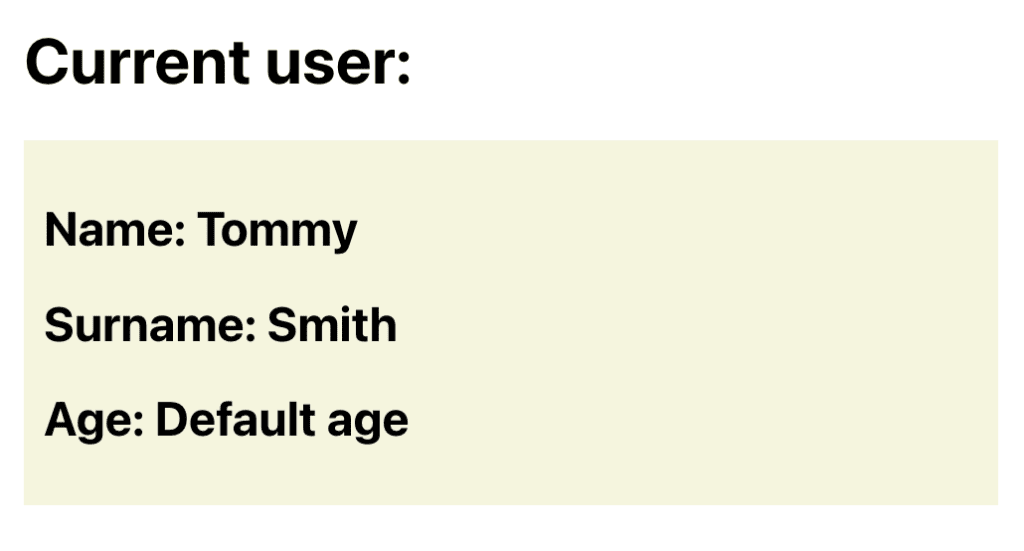
If we wanted to, we could make use of the shorthand notation and provide the default props directly in the component’s definition.
It’s also worth noting that the default props can be used for more than just passing the values to the JSX. We can, for example, use them to handle styling props as well:
const User = ({
name = 'Default name',
surname = 'Default surname',
age = 'Default age',
color = 'lightgreen'
}) => {
return (
<div style={{ backgroundColor: color, padding: '10px', marginBottom: '10px' }}>
<h2>Name: {name}</h2>
<h2>Surname: {surname}</h2>
<h2>Age: {age}</h2>
</div>
)
}
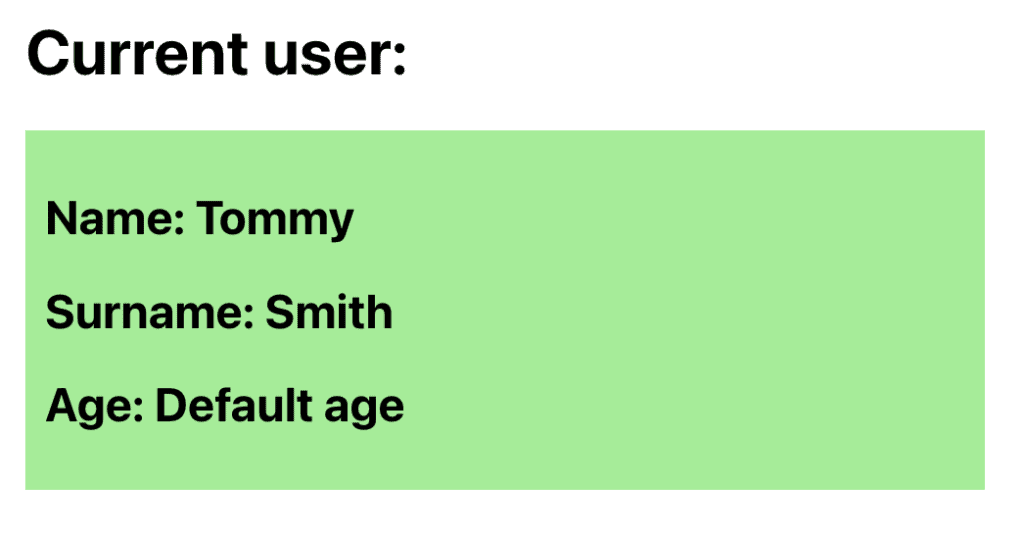
While still on the subject of default props, it’s also a common pattern, and a recommended one at that, to use it alongside the PropTypes pattern, which is actually a library for defining the types for the props you are expecting to be passed down from a parent component.
You can read more about what PropTypes is and how to use it in your project in this article.
Summary
I hope you’ve enjoyed the read and got to learn something new from this article.
In case you feel like I’ve missed something feel free to leave a comment down below where your feedback will be highly appreciated and taken into account.
See you at the next one. Cheers!
💬 Leave a comment