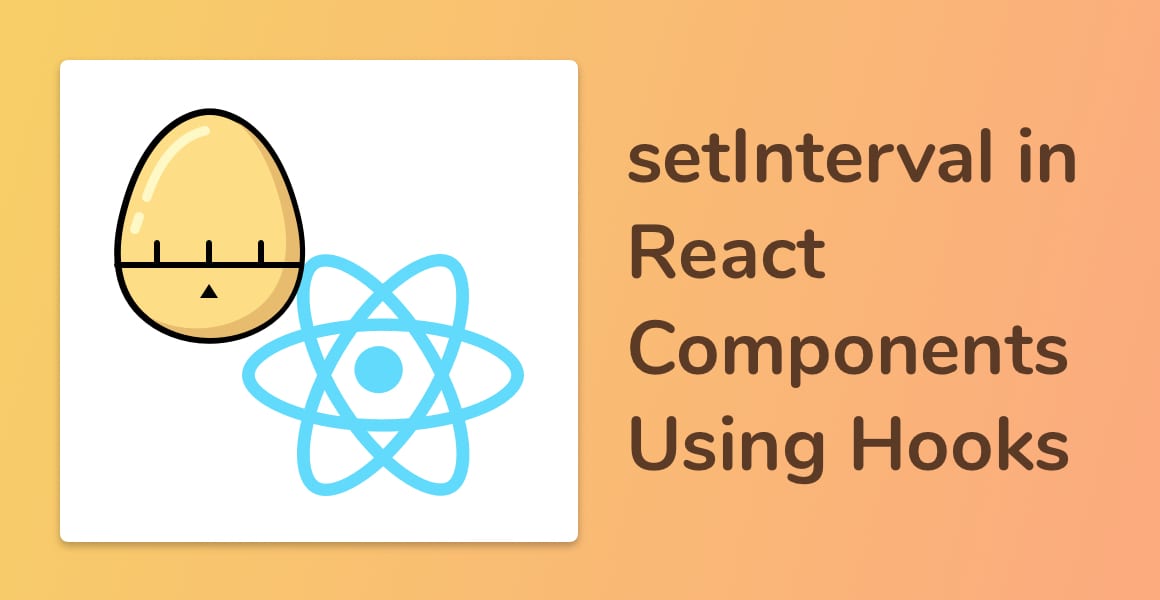
The Window object in JavaScript allows us to execute code at specified intervals. It provides us with two keys methods — setTimeout and setInterval. In this article we are going to learn about the setInterval method. Using setInterval inside React components allows us to execute a function or some code at specific intervals. Let’s explore how to use setInterval in React.
The TL;DR:
useEffect(() => {
const interval = setInterval(() => {
console.log('This will run every second!');
}, 1000);
return () => clearInterval(interval);
}, []);
The code above schedules a new interval to run every second inside of the useEffect Hook. This will schedule once the React component mounts for the first time. To properly clear the interval, we return clearInterval from the useEffect Hook, passing in the interval.
What is setInterval?
setInterval is a method that calls a function or runs some code after specific intervals of time, as specified through the second parameter.
For example, the code below schedules an interval to print the phrase: “Interval triggered” every second to the console until it is cleared.
setInterval(() => {
console.log('Interval triggered');
}, 1000);
setTimeout is a similar method that runs a function once after a delay of time. Learn about setTimeout in React Components using Hooks.
Clearing setInterval in React
A function or block of code that is bound to an interval executes until it is stopped. To stop an interval, you can use the clearInterval() method.
For example, the code below schedules a new interval when the React component mounts for the first time. After the React component unmounts the interval is cleared:
...
useEffect(() => {
const interval = setInterval(() => {
setSeconds(seconds => seconds + 1);
}, 1000);
return () => clearInterval(interval);
}, []);
...
Take a look at the useEffect Hook. At the end of the Hook, we’re returning a new function. This is the equivalent of the componentWillUnmount lifecycle method in a class-based React component. To learn more about the differences between functional components and class-based components check out this guide
The useEffect function returns the clearInterval method with the scheduled interval passed into it. As a result, the interval is correctly cleared and no longer triggers every second after the component unmounts from the DOM.
Above all, when using setInterval, it is imperative that you clear the scheduled interval once the component unmounts.
Using setInterval in React Components
To schedule a new interval, we call the setInterval method inside of a React component, like so:
import React, { useState, useEffect } from 'react';
const IntervalExample = () => {
const [seconds, setSeconds] = useState(0);
useEffect(() => {
const interval = setInterval(() => {
setSeconds(seconds => seconds + 1);
}, 1000);
return () => clearInterval(interval);
}, []);
return (
<div className="App">
<header className="App-header">
{seconds} seconds have elapsed since mounting.
</header>
</div>
);
};
export default IntervalExample;
The example above shows a React component, IntervalExample, scheduling a new interval once it mounts to the DOM.
The interval increments the seconds state value by one, every second.
Finally, we display the number of seconds in the return function to show how many seconds have elapsed since the component mounts.
The code above will give us a working demo that looks something like this:
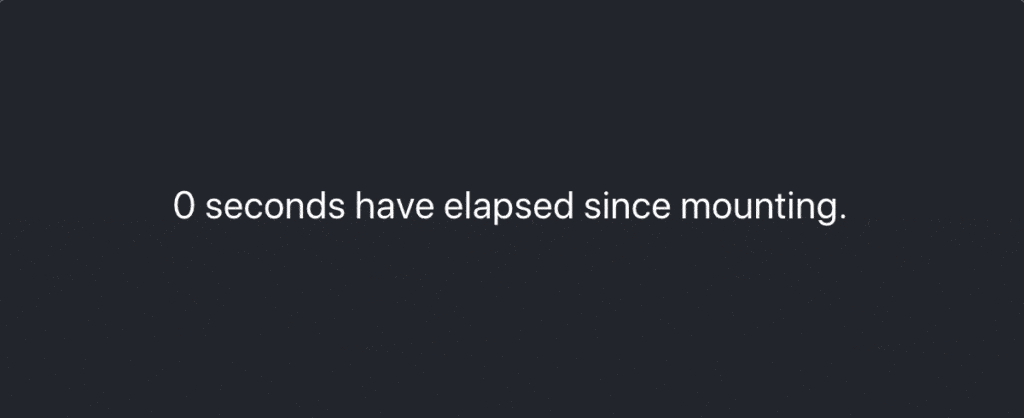
💬 Leave a comment