We have learned about pure components, functional components, class components, and many more. This time around we are going to dig into uncontrolled components react, and how you can implement them with examples.
Uncontrolled Components 🤨
Majority of the time when we are making our custom components we tend to make controlled components since with controlled components you can handle the data within the react component. On the other hand, uncontrolled components are directly manipulated and handled by DOM itself.
How Does That Help Us? 🧐
When you think of it both controlled and uncontrolled components have their pros and cons. However, with uncontrolled components, you can perform react and non-react code since it keeps the source of truth inside the DOM.
Examples
function CustomComponent() {
const emailRef = useRef(null);
const phoneNumberRef = useRef(null);
const firstNameRef = useRef(null);
const lastNameRef = useRef(null);
const referralRef = useRef(null);
const passwordRef = useRef(null);
const onSubmit = (event) => {
alert(
`Current values of the form ${emailRef.current.value}, ${phoneNumberRef.current.value}, ${firstNameRef.current.value}, ${lastNameRef.current.value}, ${referralRef.current.value}, ${passwordRef.current.value}`
);
event.preventDefault();
};
return (
<form onSubmit={onSubmit}>
{/* register our input field with register function provided by the useForm hook */}
<input placeholder="Enter email" ref={emailRef} />
<input placeholder="Enter phone number" ref={phoneNumberRef} />
<input placeholder="Enter first name" ref={firstNameRef} />
<input placeholder="Enter last name" ref={lastNameRef} />
<input placeholder="Enter referral" ref={referralRef} />
<input
placeholder="Enter your password"
type={"password"}
ref={passwordRef}
/>
<input type="submit" />
</form>
);
}
Here is our uncontrolled component handling our form using the useRef hook. One might say uncontrolled components look thinner and tidy, but look at the table below before you make up your mind 😛
feature | uncontrolled | controlled |
---|---|---|
one-time value retrieval (e.g. on submit) | ✅ | ✅ |
validating on submit | ✅ | ✅ |
instant field validation | ❌ | ✅ |
conditionally disabling submit button | ❌ | ✅ |
enforcing input format | ❌ | ✅ |
several inputs for one piece of data | ❌ | ✅ |
dynamic inputs | ❌ | ✅ |
Although, there are times when uncontrolled components are the one and only option like
<input type="file" />
Files inputs are to be set by users themselves and cannot be done programmatically. Or let’s say if you are trying to access an audio player and you need to go into the element itself to do actions like PLAY and PAUSE.
Uncontrolled Form Component In Action
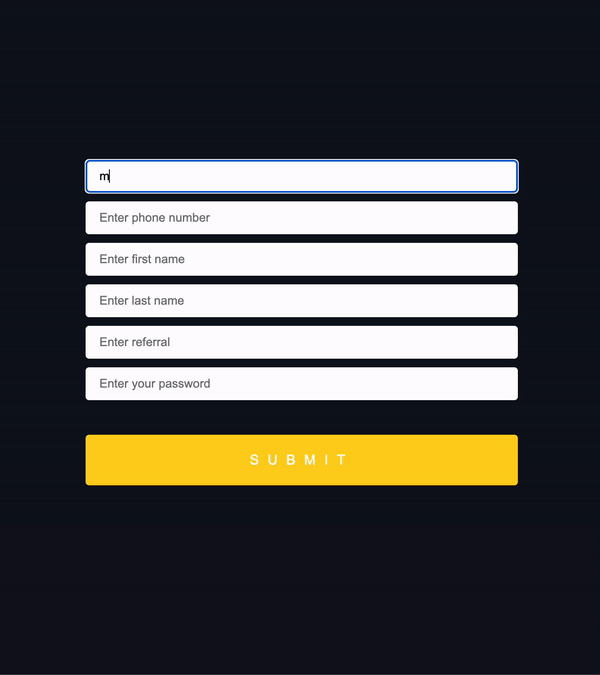
Wrap Up
We have concluded uncontrolled components in this one and technically controlled components as well. I hope you have an idea of how to implement both of them and know better when to use which one. I hope to see you in the next and this is not a goodbye. Take care.
💬 Leave a comment