Views and components are one of the first things you’ll work with when starting a new project in Vue.js. In this article, we’ll explore the nature and functionality of Vue.js views and components, and see if there’s any difference between them.
Views in Vue.js are components that point to the actual web page you want the visitor to visit. Since Vue.js is a component-based framework, the only real distinction between a view and a component is how they are organized within a project’s folder hierarchy.
In the Vue.js framework, there’s really no functional difference between views and components; but there are conceptual ones. Simply put, a view component is what would traditionally be called a “page”, while a component is a reusable piece of code that can be integrated into several views.
In order to navigate between pages, we can use Vue router, which is the standard package for page navigation in Vue.js applications and is widely used in the framework.
With the aid of the Vue router, we can connect the URL/history to the Vue.js components; this allows us to have certain pathways render the corresponding Vue.js view component. On the other hand, we can use several components inside a single Vue page.
In this article we’ll explore in more detail what the difference is between views and components. Let’s get started!
So, What Is a Vue.js Component?
In Vue.js, any .vue file can serve as both a view and a component. Thought to arrange your Vue.js project, what we normally understand as a component is anything that is reusable and we will use in different pages.
A component might be a header, a footer, a badge, a table, some text fields, or a button. You can use any of them in different pages.
Two very common examples are our Header and Footer components. Most pages use them, and it wouldn’t make sense to rewrite them any time we want to show them. Thus, we can create specific components for them:
<!-- Header component -->
<template>
<h2>This is my header component</h2>
</template>
<script>
export default {};
</script>
<style></style>
<!-- Footer component -->
<template>
<h2>This is my footer component</h2>
</template>
<script>
export default {};
</script>
<style></style>
What, then, are pages?
On the other hand, pages are Vue components that will show up in a specific URL and that can’t be reused in different parts of an application.
For example, in a normal web page, we could have the following list of pages:
- Home.
- About.
- Projects.
- Contact Us.
Each one of those pages would be its own .vue file, which could in turn contain different components to make up their structure. For example:
<!-- Home Page -->
<template>
<Header />
<p>This is the content in my Home page</p>
<Footer />
</template>
<script>
import Header from "../components/Header.vue";
import Footer from "../components/Footer.vue";
export default {
components: { Header, Footer },
};
</script>
Folder Organization for Vue.js Components
If you’ve never worked with Vue.js before, you should know that the framework’s components all adhere to a uniform folder structure. This structure is automatically set up when you create a new project.
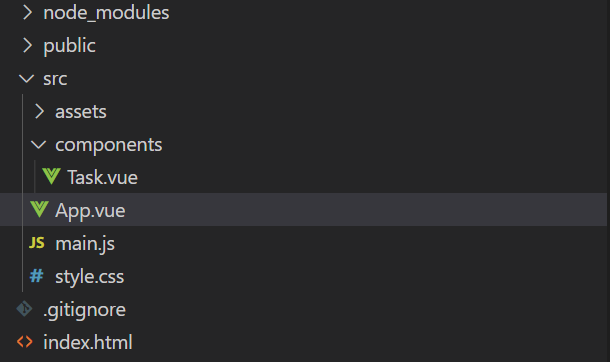
The preceding image shows the default Vue.js project folder structure. The components are in the subdirectory src/components. Even though this kind of folder organization is useful for managing parts of smaller projects, it becomes increasingly difficult to traverse as the project expands.
However, to make it easier to explore larger projects, we need to further organize our components folder structure. For example, most times we separate views and components into their own directories.
Views are the pages we want the user to be able to access. Home.vue and About.vue are two examples of files that can be stored in this folder.
On the other hand, any files that can be utilized elsewhere in the project or in the construction of other view components go in a “components” folder:
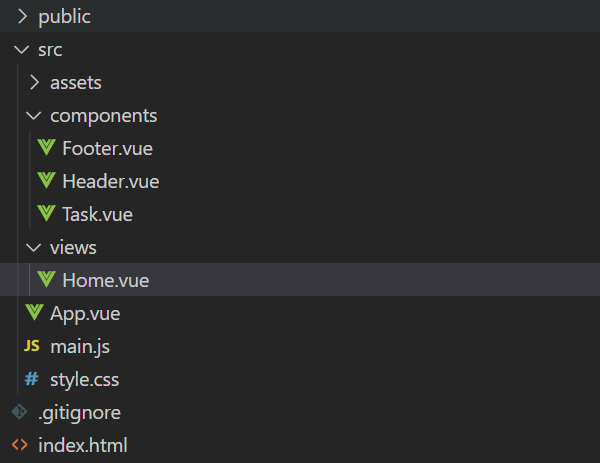
Is Vue Router Necessary for Vue.js?
There is no native router support in Vue.js, as it was originally intended to build SPAs. However, we can use the Vue Router package to navigate between pages, making it an ideal choice for managing routes in Vue.js applications.
When working with Vue.js, you’re free to use any generic routing library you like, but the official one is Vue Router, which is developed and maintained by the same team. By the way, if you don’t know how to install it in your project, we created a guide that you can access here.
Although you can create a Vue project without a router, that would mean that you’re stuck with an SPA. Therefore, you won’t be able to create different pages and navigate between them, at least not without a lot of effort.
Final Reflections
All in all, components are reused elements that can contain their own data and logic. While views are rendered by the router and don’t necessarily have to be reusable, they can still contain child components.
By understanding the definition and use cases for both Vue views and components, you can confidently start tinkering with these fundamental project building blocks in your future web development projects.
Want more? If this article hasn’t quite scratched your Vue itch yet, never fear! Check out some of our other Vue tutorials and articles.
💬 Leave a comment